Lifetime access to this course
Array.filter()
In this lesson, you'll learn about the array.filter() method in JavaScript, which is used to create a new array containing elements that pass a specified test. This method is a powerful tool for extracting elements from an array based on specific criteria . ### Array.filter() The filter() method creates a new array with all elements that pass the test implemented by the provided function. It's a concise and efficient way to filter out unwanted elements from an array. #### Filtering Positive Numbers Let's start with a simple example where we filter out positive numbers from an array:
The rest of this lesson is waiting.
Join JS Mastery Pro to unlock it.
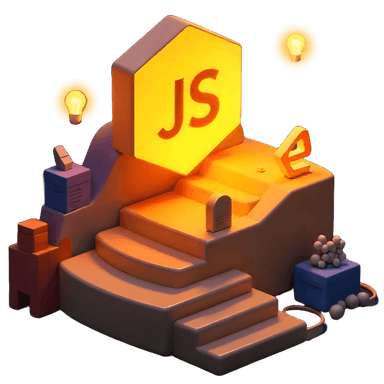
Complete Path to JavaScript Mastery
$300
Buy the Course OnlyBuild advanced web app
Certificate of completion included
Great for focused learners who just want this course
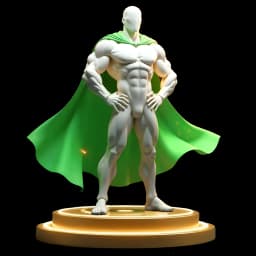
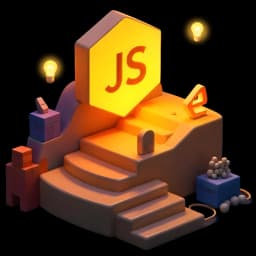
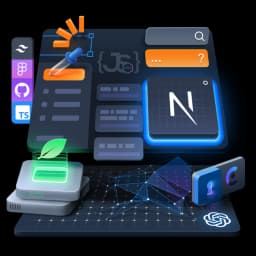
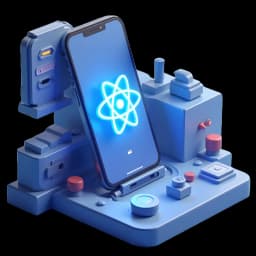
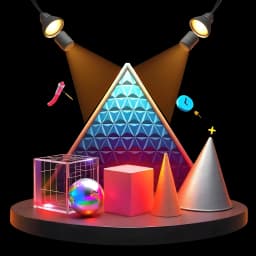
Get Full Access
$149 /month
Lock in a year & save—2 months free!
Access to all courses
Quizzes, mini-challenges, AI summaries, inline sandboxes
Participation in a private community
Build real production-grade apps
Mock interviews & project reviews
Weekly live sessions & member-only access