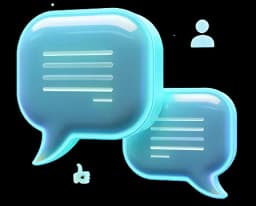
Join the Conversation!
Subscribing gives you access to the comments so you can share your ideas, ask questions, and connect with others.
In this lesson, you'll learn about the method in JavaScript, which is used to execute a function for each element in an array. This method provides a cleaner and more readable alternative to the traditional loop for certain use cases.
The method performs an action for each element in the array. While you can achieve the same result using a standard loop, offers a more concise and expressive syntax.
Let's take an example where we need to log each value in an array called to the console, along with their respective indices . Here's how we can do it using a standard loop:
let names = ['Jon', 'Jenny', 'Johnny'];
for (let i = 0; i < names.length; i++) {
console.log(i, names[i]);
}
// Output:
// 0 'Jon'
// 1 'Jenny'
// 2 'Johnny'
In this case, we declare and initialize a variable , and the loop compares it with . If the expression evaluates to , the loop executes. After the loop body is executed, the value of increments by one.
Here is the syntax of the method:
array.forEach((value, index) => {
// ...
});
The first argument of the method is the function you want to execute for each element of the array. This can have three arguments:
So, we can perform the same action using in this manner:
let names = ['Jon', 'Jenny', 'Johnny'];
names.forEach((value, index) => {
console.log(index, value);
});
If you want to use a named function as a callback, it can be done like this:
function logArrayElement(element, index) {
console.log(index, element);
}
names.forEach(logArrayElement);
Ensure that the order of arguments in the predefined/named function used as the callback matches the syntax requirements .
This method returns and is not chainable, meaning you can't call another method on the array after using . Therefore, you cannot use cascading notation with .
let returnValue = names.forEach(function (value) {
console.log(value);
});
console.log(returnValue); // undefined
Typically, this method is used to execute , such as logging all the elements in the array after performing a series of actions on the array.
Use When:
Don't Use When:
Here is a real example where can be used:
let sum = 0;
const numbers = [65, 44, 12, 4];
numbers.forEach((number) => {
sum += number;
});
console.log(sum); // 125
The method is a powerful tool for iterating over arrays, providing a clean and expressive way to perform actions on each element.
"Please login to view comments"
Subscribing gives you access to the comments so you can share your ideas, ask questions, and connect with others.