Lifetime access to this course
Array Methods
In this lesson, you'll learn about various array methods in JavaScript, which provide powerful ways to manipulate and interact with arrays. We'll cover both basic and advanced methods, with examples to illustrate their use. ### Array Methods Arrays in JavaScript come with a variety of built-in methods that allow you to manipulate their data, such as adding or removing elements at certain positions. Let's take a look at some of the most basic and useful ones. ### Basic Array Methods
The rest of this lesson is waiting.
Join JS Mastery Pro to unlock it.
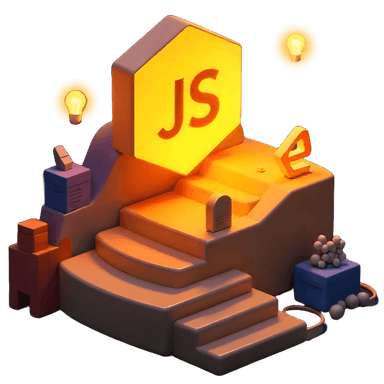
Complete Path to JavaScript Mastery
$300
Buy the Course OnlyBuild advanced web app
Certificate of completion included
Great for focused learners who just want this course
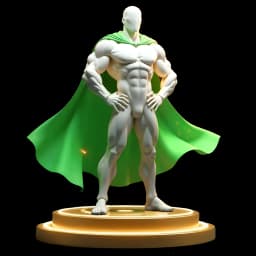
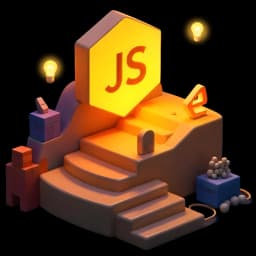
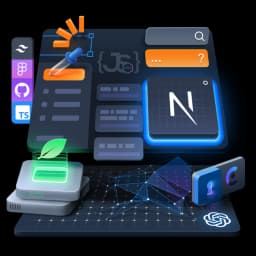
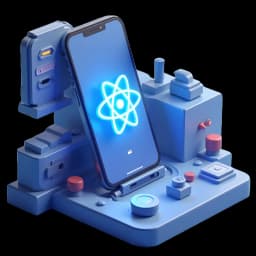
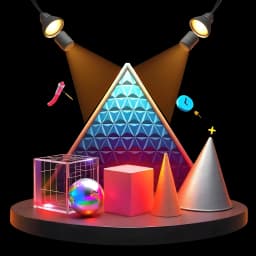
Get Full Access
$120 /month
Lock in a year & save—2 months free!
Access to all courses
Quizzes, mini-challenges, AI summaries, inline sandboxes
Participation in a private community
Build real production-grade apps
Mock interviews & project reviews
Weekly live sessions & member-only access