Lifetime access to this course
Array.reduce()
In this lesson, you'll learn about the array.reduce() method in JavaScript, which is used to reduce an array to a single value by iterating over its elements and applying a function. ### Array.reduce() The reduce() method starts with all the elements from an array, iterates over them, and computes them to a single value . It's one of the more complex array methods, but it's incredibly powerful for tasks like summing numbers , finding maximum values , and more. #### Example: Calculating Total Price
The rest of this lesson is waiting.
Join JS Mastery Pro to unlock it.
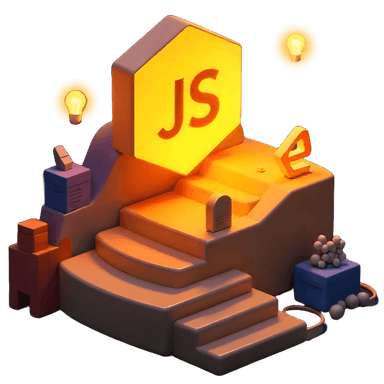
Complete Path to JavaScript Mastery
$300
Buy the Course OnlyBuild advanced web app
Certificate of completion included
Great for focused learners who just want this course
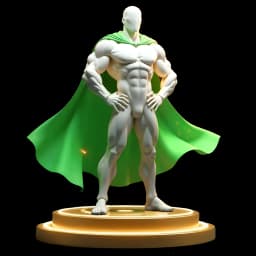
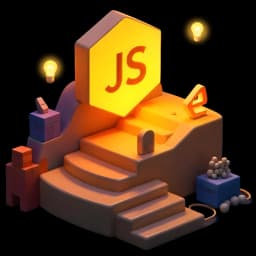
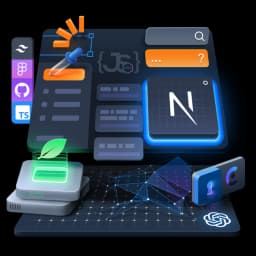
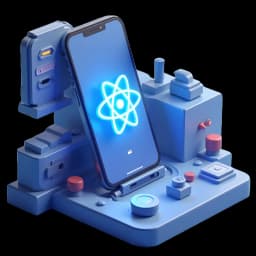
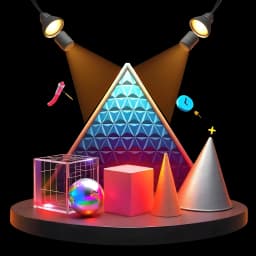
Get Full Access
$120 /month
Lock in a year & save—2 months free!
Access to all courses
Quizzes, mini-challenges, AI summaries, inline sandboxes
Participation in a private community
Build real production-grade apps
Mock interviews & project reviews
Weekly live sessions & member-only access