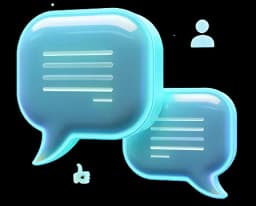
Join the Conversation!
Subscribing gives you access to the comments so you can share your ideas, ask questions, and connect with others.
In this lesson, you'll learn about the and methods in JavaScript, which are used to test whether some or all elements in an array pass a specified test.
The method tests whether at least one element in the array passes the test implemented by the provided function. It returns if the callback function returns a truthy value for any array element; otherwise, it returns .
Let's say we want to check if there are any even numbers in an array:
var numbers = [1, 3, 5, 8, 9];
// Check if there is at least one even number
var hasEvenNumber = numbers.some(function(number) {
return number % 2 === 0;
});
console.log(hasEvenNumber); // true
The method tests whether all elements in the array pass the test implemented by the provided function. It returns if the callback function returns a truthy value for every array element; otherwise, it returns .
Let's say we want to check if all numbers in an array are positive:
var numbers = [1, 3, 5, 8, 9];
// Check if all numbers are positive
var allPositive = numbers.every(function(number) {
return number > 0;
});
console.log(allPositive); // true
The and methods are powerful tools for evaluating conditions across array elements, making them essential for data validation and logic checks in JavaScript.
"Please login to view comments"
Subscribing gives you access to the comments so you can share your ideas, ask questions, and connect with others.