Lifetime access to this course
Async/Await
In this lesson, you'll learn about async/await , a modern addition to JavaScript that simplifies working with promises. Async/await provides a more intuitive and readable way to handle asynchronous operations, making your code look and behave more like synchronous code. ## Async/Await Async/await is a syntactic sugar built on top of promises. It allows you to write asynchronous code that looks and behaves like synchronous code, which makes it easier to read and maintain. ### Advantages
The rest of this lesson is waiting.
Join JS Mastery Pro to unlock it.
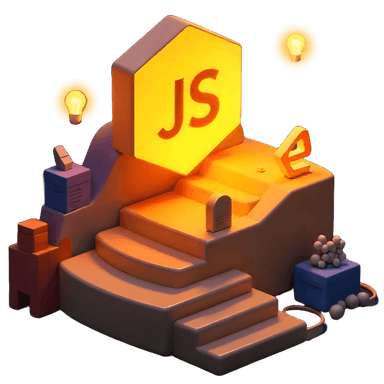
Complete Path to JavaScript Mastery
$300
Buy the Course OnlyBuild advanced web app
Certificate of completion included
Great for focused learners who just want this course
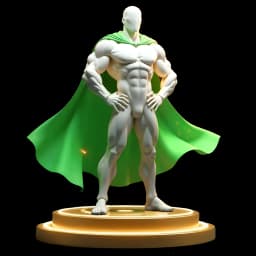
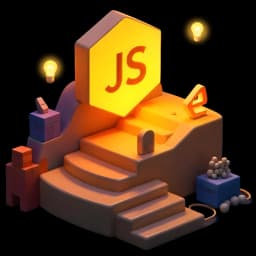
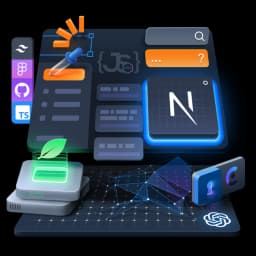
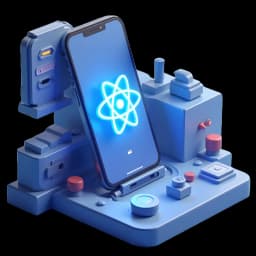
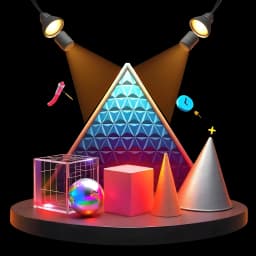
Get Full Access
$120 /month
Lock in a year & save—2 months free!
Access to all courses
Quizzes, mini-challenges, AI summaries, inline sandboxes
Participation in a private community
Build real production-grade apps
Mock interviews & project reviews
Weekly live sessions & member-only access