Lifetime access to this course
Async JavaScript & Callbacks Part 1
In this lesson, you'll learn about handling asynchronous operations in JavaScript, focusing on data fetching, callback functions, and how to simulate asynchronous behavior. These concepts are essential for building applications that interact with external data sources, such as APIs. ### Simulating Asynchronous Data Fetching Let's start with a real-world scenario: fetching data from an API. APIs, or Application Programming Interfaces, allow you to access data from various services. When fetching data, the time it takes can vary based on factors like data size and network speed. Unlike setTimeout , where you specify a delay, real data fetching times are unpredictable. Consider the following example where we simulate fetching a user from a database:
The rest of this lesson is waiting.
Join JS Mastery Pro to unlock it.
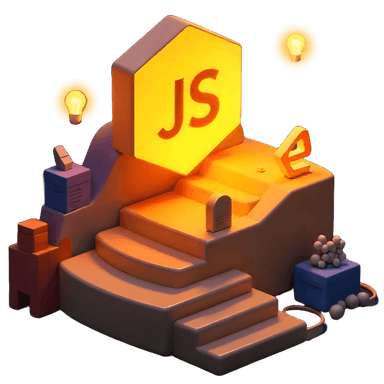
Complete Path to JavaScript Mastery
$300
Buy the Course OnlyBuild advanced web app
Certificate of completion included
Great for focused learners who just want this course
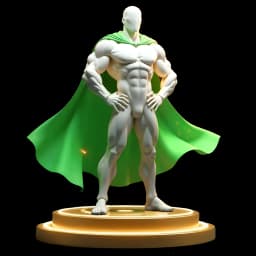
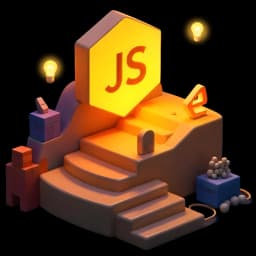
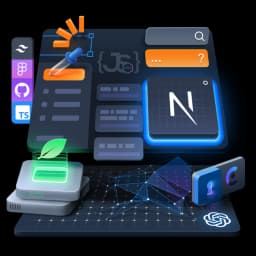
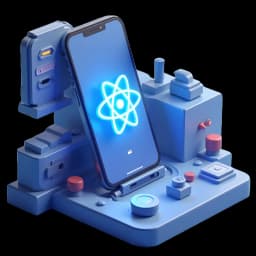
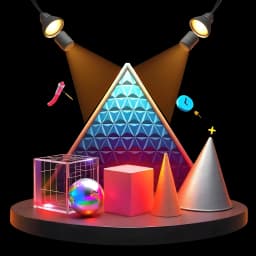
Get Full Access
$120 /month
Lock in a year & save—2 months free!
Access to all courses
Quizzes, mini-challenges, AI summaries, inline sandboxes
Participation in a private community
Build real production-grade apps
Mock interviews & project reviews
Weekly live sessions & member-only access