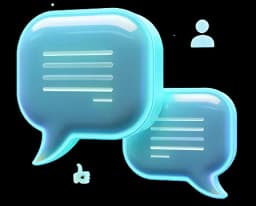
Join the Conversation!
Subscribing gives you access to the comments so you can share your ideas, ask questions, and connect with others.
In this lesson, you'll learn about the differences between synchronous and asynchronous JavaScript. Understanding these concepts is crucial for writing efficient and responsive code, especially when dealing with tasks like data fetching and user interactions.
Synchronous JavaScript is code that is executed line by line, with each task completing instantly. There is no delay in the execution of tasks for these lines of code.
Here's an example of synchronous JavaScript:
const functionOne = () => {
console.log('Function One');
functionTwo();
console.log('Function One, Part 2');
}
const functionTwo = () => {
console.log('Function Two');
}
functionOne();
// Output:
// Function One
// Function Two
// Function One, Part 2
Now, let's explore asynchronous JavaScript by modifying the functions:
const functionOne = () => {
console.log('Function One'); // 1
functionTwo();
console.log('Function One, Part 2'); // 2
}
const functionTwo = () => {
setTimeout(() => console.log('Function Two'), 2000); // 3
}
functionOne();
// Output:
// Function One
// Function One, Part 2
// (after a two-second delay)
// Function Two
Why doesn't JavaScript wait?
What is asynchronous JavaScript?
Asynchronous JavaScript involves code where some tasks take time to complete. These tasks run in the background while the JavaScript engine continues executing other lines of code. When the result of the asynchronous task is available, it is then used in the program.
Understanding the difference between synchronous and asynchronous JavaScript is vital for building applications that are both efficient and responsive. As we progress, you'll learn more about handling asynchronous operations using promises, async/await, and other techniques.
"Please login to view comments"
Subscribing gives you access to the comments so you can share your ideas, ask questions, and connect with others.