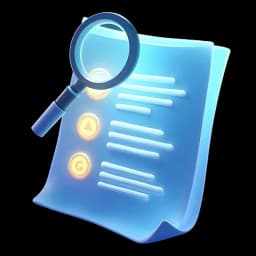
Quick Lecture Overview
Subscribing gives you access to a brief, insightful summary of each lecture to stay on track.
In this lesson, you'll gain deeper insights into asynchronous JavaScript, including Promises, async/await, and API data fetching. Additionally, you'll revisit the concept of object destructuring, which is a powerful feature in JavaScript for extracting values from objects and arrays.
To truly master these concepts, I recommend watching this comprehensive video that covers:
To follow along with the video and practice these concepts, you'll need to have Node.js installed on your system. Node.js provides a runtime environment for executing JavaScript code outside of a browser, which is essential for server-side development and running JavaScript applications locally.
Installation Steps:
Once Node.js is installed, you'll be ready to dive deeper into these advanced JavaScript topics.
By logging in, you'll unlock full access to this and other free tutorials on JSM Pro.
Why? Logging in lets us personalize your learning experience, track your progress, and keep you in the loop with new workshops, coding tips, and platform updates.
You'll also be the first to know about upcoming launches, events, and exclusive discounts.
No spam—just helpful content to level up your skills.
If that sounds fair, go ahead and log in to continue →
Enter your name and email to get instant access
##Looks like we found a thief monkey By the way, I liked the trick how you reached till here. You have a good sense of humor. You will improve a lot if you join our course with this passion.
var
(function-scoped, outdated)let
(block-scoped, modern and recommended)const
(block-scoped, cannot be reassigned)_
, or $
let let = 5;
is invalid)myVar
and myvar
are different)string
, number
, boolean
, null
, undefined
, bigint
, symbol
Objects
, Arrays
, Functions
Subscribing gives you access to a brief, insightful summary of each lecture to stay on track.
00:00:00 Hello everyone and welcome to another JavaScript Mastery video.
00:00:04 In this tutorial we will build a simple but extremely educational application that is going to improve your overall knowledge of destructuring and async await.
00:00:15 The program will take in a currency code we want to convert from and currency code we want to convert to as well as the amount of money.
00:00:23 The output will result in a correct exchange rate from not one but two different APIs.
00:00:30 In simple words, we're going to build a currency converter.
00:00:34 But on the way, we're going to learn a lot about async functions.
00:00:38 As you can see here, we don't have one, but two async functions.
00:00:42 And at the end, we also have the promise.all.
00:00:46 We also have a lot of destructuring happening here.
00:00:48 You can see we are destructuring the data, destructuring the name, destructuring the data, and then the rates.
00:00:54 And then at the end, we also have some array destructuring right there.
00:00:59 And finally, but most importantly, we're going to take a look at this amazing tool called Quokka.js, which enables you to see in real time in your editor,
00:01:08 the values of all the variables you have.
00:01:11 Quokka is a developer productivity tool for rapid JavaScript development.
00:01:15 Simply said, using Quokka, all values are updated and displayed in your code editor next to your code as you type.
00:01:24 No more needs for cons and logs or losing yourself while trying to debug a simple error.
00:01:29 In here we have the value of the euro, the exchange rate and it's all listed immediately on the right side of the editor.
00:01:36 You can see we're getting the names here and at the end we're getting the full output in real time on the right side.
00:01:42 That's crazy.
00:01:43 I was really astonished when I found out about this tool.
00:01:46 You can install Quokka on all major code editors.
00:01:50 It is supported for Visual Studio Code, JetBrains IDE, Atom, and also Sublime Text.
00:01:57 The only thing that you have to do to install it is to go into your code editor of choice and then install the extension.
00:02:04 That's it.
00:02:05 In this case, we're going to install it together using Visual Studio Code.
00:02:09 To install it, you're going to go to your extensions tab in Visual Studio Code.
00:02:13 That's this one right there.
00:02:15 And you're going to type in Quokka with a double K.
00:02:19 You can get it right there.
00:02:20 You can see 758,000 downloads.
00:02:23 And you can click install right there.
00:02:25 I already have it installed.
00:02:26 So I'm not going to do that.
00:02:28 And once it's installed, that's it, we can now run Quokka commands.
00:02:32 So you can now open a command palette by pressing Ctrl or Command Shift P.
00:02:38 And then this little window is going to pop up.
00:02:40 You can now start typing and enter a new file.
00:02:44 So you can click new file right there.
00:02:47 And we're going to explore some of the pre-built examples that showcase how useful Quokka really is.
00:02:53 Let's try with a simple array.concat.
00:02:57 Once you click it, it's going to open the file for you, and now it immediately starts running Quokka.
00:03:03 So what can we do to test it out?
00:03:05 Let's try double clicking the variable array1, as you can see the values are immediately there, or array2.
00:03:13 As you can see, on the right side, you immediately get their values.
00:03:17 Isn't that crazy?
00:03:19 Finally, all console logs are immediately shown on the right side as well.
00:03:23 As you type, Quokka is going to let you know if you have any errors or if you misspelled a variable name.
00:03:29 For example, let's try with arr1 instead of array1.
00:03:33 As you can see, Quokka immediately saved me there.
00:03:36 Let's try adding that array.
00:03:38 and there we go it immediately shows the green square and we have our values on the right side you might have noticed that we have colored squares on the
00:03:47 left side green means that something has been successfully executed red is obviously an error and white means that we didn't even reach that line in our
00:03:57 code that's really handy because as you can see the error occurred on line four so line six wasn't even executed With the pro version of Quokka you can
00:04:06 also use this handy syntax.
00:04:08 Comment and then question mark.
00:04:10 As you can see, we immediately get the values of our variables on the right side.
00:04:15 I'm still shocked on how well this works.
00:04:17 Finally, since we are using Quokka, we didn't even have to do icons a lot.
00:04:22 We can immediately get the values just by hovering over the expression.
00:04:27 One last thing that I would like to show you in this little Quokka demo is the value explorer.
00:04:33 So if you create an object right there, let's try with const person.
00:04:37 And let's say that that person has a first name.
00:04:40 Let's do John, as we always do.
00:04:43 And let's say that the person also has a car.
00:04:46 That car is going to have a color of red, and it's going to have wheels of four, just like that.
00:04:54 Now, if you take a look, we have the simple person object.
00:04:57 Again, with Quokka, we can easily see the values of it.
00:05:00 That's really handy.
00:05:01 But Quokka has something even more powerful for seeing where the values are.
00:05:05 It's called Value Explorer.
00:05:07 It's right here on your left side sidebar.
00:05:10 You can click this Quokka icon and it's going to lead you to the value explorer.
00:05:15 In here you can see where your values are.
00:05:18 If we type right there you can see that online one we have the array with three elements and then you can see what elements do we have.
00:05:25 then again with array two really simple example but with person now if you click this you can see we have the first name of john but we also have a nested
00:05:34 car property right there which we also get all the properties so when could this be extremely useful when you have a huge file with a lot of lines a lot
00:05:43 of variables that maybe you didn't declare but you fetched from somewhere with this you could easily know what values are you getting from these variables
00:05:52 extremely useful tool.
00:05:53 There's a link in the description.
00:05:55 Definitely make sure to give it a try.
00:05:57 The community version is completely free.
00:06:00 Now let's dive into the main project of this video, the currency converter.
00:06:12 The first thing we're going to do is create a new folder.
00:06:16 We can name it something like currency converter.
00:06:20 I have one so I'm going to name it YouTube.
00:06:22 You can just name yours currency converter and then we're going to drag and drop it into our Visual Studio code.
00:06:29 In there, it should open and then we're going to open our terminal.
00:06:33 You can open your terminal by going to view and then write their terminal at the top.
00:06:39 Once it opens, we're going to run npm init and then dash y.
00:06:45 Once you do this, it's going to generate a package that JSON and that's going to enable us to run npm install, and then axios.
00:06:53 We're going to use axios to make our API calls.
00:06:58 Once that is done, we can create a file, we can name it index.js.
00:07:04 and all of our code is going to be written in there.
00:07:06 Great, so we can now close our terminal and then in here, I'm going to paste some code.
00:07:12 And these will be the links to our APIs.
00:07:15 In this application, we're going to receive data from two asynchronous sources.
00:07:21 First one is called Fixer for an exchange rates and currency conversion JSON API.
00:07:27 You'll need to sign up for free so that you can use API's access key.
00:07:31 This API will provide us with the data needed to calculate exchange rate between currencies.
00:07:37 you can go to fixer.io, link is going to be down in the description, and then you can click get free API key.
00:07:44 After you sign up, you should be able to get the API for free.
00:07:48 So that's the first one.
00:07:49 And the second one is called rest countries.
00:07:52 This API will give us all countries that we can use our new currency in.
00:07:57 So we can go to currency.
00:07:58 And then in here, if we try to make a mock request, let's try pasting that right there.
00:08:04 So for example, we can use the COP, Colombian peso in one country, and that country is Colombia.
00:08:11 Great.
00:08:12 So that's how our APIs are going to work.
00:08:14 If we come back in here, This code is going to be pasted in the description so you can take a look at it.
00:08:19 Make sure to put your own API key right there.
00:08:22 And with that, we're basically ready to start with coding.
00:08:26 We're going to run the command palette by pressing Command Shift P or Control Shift P on Windows.
00:08:32 And then we're going to click Start on current file with Quokka.
00:08:36 This is going to make sure to start the output using the Quokka.
00:08:39 And you should see Quokka index.js also green colored squares should appear on the left side.
00:08:45 Great.
00:08:46 Now if we double click something you can see we get the value of it immediately on the right side.
00:08:52 That's amazing.
00:08:53 So let's talk about async await for a second because whole this project is going to be about learning async await.
00:09:01 Async await is in my opinion the best way to write asynchronous code.
00:09:05 It is built on top of promises, therefore it is also non-blocking.
00:09:10 The big difference is that asynchronous code written using async and await looks and behaves a little more like synchronous code.
00:09:19 This is where all its power lies.
00:09:21 So how can we await for something?
00:09:24 Well, we can await for it inside of asynchronous functions.
00:09:28 And how do we create asynchronous functions?
00:09:30 Well, you create a normal function, let's do something like const add is equal to a function that's going to return a plus b,
00:09:39 whereas a and b are parameters.
00:09:42 The only thing you have to change to make this function asynchronous is to add the async keyword before the function.
00:09:49 That's it.
00:09:50 Now, if we tried calling the function with two and three, using quokka is going to be pretty straightforward, we immediately get the value.
00:09:57 The thing is, the return value of the asynchronous function is not going to be the value itself.
00:10:04 It's going to be a promise.
00:10:06 If there is only one thing that you get from this whole video is that you need to remember asynchronous functions return promises.
00:10:14 They don't return values.
00:10:17 async functions provide us with a clean and concise syntax that enables us to write less code to accomplish the same outcome we would get with promises.
00:10:27 The second most important aspect of asynchronous functions is that they can be paused by using await.
00:10:33 So in here, if we do something like cons sum is equal to await a plus b, then we can return the sum Basically what we are saying is that,
00:10:44 and again see how quokka saved me there from this typo, is again that we need to wait for the value to come here.
00:10:51 This example doesn't make any sense in the asynchronous world because calculation of two numbers is synchronous.
00:10:59 Great.
00:10:59 Now that we talked a bit about async functions, let's dive right into creating our program.
00:11:05 Our goal for this program is to have three functions, not one, not two, but three asynchronous functions.
00:11:13 The first one is going to fetch data about currencies.
00:11:16 So we're going to write that there, fetch data about currencies.
00:11:20 The second function is going to fetch data about countries.
00:11:25 We set countries that we can use our newly exchange currency in.
00:11:29 And the third function is going to gather all that information into a single place and then output it nicely to the user.
00:11:36 So we can say output data.
00:11:38 With that said, we can now get to creating our first asynchronous function.
00:11:44 The name of our first function is going to be get exchange rate.
00:11:51 and it's going to accept two parameters.
00:11:53 First one is going to be fromCurrency and the second one is going to be toCurrency.
00:11:59 So when we're calling this function getExchangeRate, First thing that we have to provide is from which currency do we want to exchange money from?
00:12:07 In this case, let's do a USD, which is American dollar.
00:12:12 And then the second parameter is going to be the money we want to exchange to, so we can put that as Euro.
00:12:19 Great.
00:12:19 Now, if we save that, in here, we implement the logic.
00:12:24 First, we have to make a call to our first API to get some data about exchange rates.
00:12:29 So we can do const.
00:12:31 And let's do response.
00:12:32 const response is equal to axios.get.
00:12:37 And then in here, we're going to put this fixer API that we had stored above.
00:12:42 Now, what Quokka does extremely well is if you just hover over this response here, you're going to get a scrollable window where you can see all the data
00:12:51 that we are getting.
00:12:52 Obviously, we're not concerned with the data about the request itself, we only want to get the data.
00:12:57 So if you scroll, there we go.
00:13:00 There is a data object belonging inside of our response object.
00:13:05 So we can console log that like this.
00:13:08 Let's do response.data.
00:13:10 Keep in mind, we don't even have to console log anymore.
00:13:13 I can just do this.
00:13:15 And you can see that we get undefined, although we were expecting to see some data.
00:13:20 That is because from this line to this line, the code goes synchronously.
00:13:25 It happens instantaneously.
00:13:26 Line 12, 13, 14. By the time that our code go to line 14, this response to data here, JavaScript didn't yet fetch the data about a response and we couldn't
00:13:38 get it.
00:13:39 That's why we have to tell JavaScript, oh, wait a second.
00:13:43 And that's why we use the await keyword.
00:13:45 But there's only one more rule.
00:13:47 To actually await for something, we have to have the asynchronous function wrapping that await keyword.
00:13:53 Now, if we tried console logging this, we're just hovering over it.
00:13:57 Now you can see we do get real data.
00:13:59 And I keep saying console log it, but using Quokka, you don't have to console log anything anymore.
00:14:05 So in here, we do get the date, we get the base currency, and we get timestamps.
00:14:10 The only thing we really need are rates.
00:14:12 That's why we can access only response.data.rates.
00:14:16 And that should give us some meaningful information.
00:14:19 Now, if we do this, we get all the data here, but it also shows up here.
00:14:23 And that's it, the value of all the currencies in the world when compared to the euro.
00:14:28 Now, if you used destructuring before, then this seems kind of redundant.
00:14:31 We don't have to do response, that data, that rates, just to get the rates.
00:14:36 We only need the rates, right?
00:14:37 So we can immediately destructure the things from the response.
00:14:41 So in here, we can use the destructuring assignment, which is just an empty object right there.
00:14:47 So right now, once you do that, we are immediately inside the response, because in here was the place where response was.
00:14:55 So we can say, what do you want to take from the response?
00:14:59 And in this case, we want to take the data, right?
00:15:03 So right now, we say we want the data.
00:15:05 And now we're basically at the same place, as you can see, we do get the rates, but we didn't have to say response that data that rates.
00:15:12 And finally, we can do the same thing to destructure the rates only, we're going to use the colon sign and then put another pair of curly braces.
00:15:20 And then in there, we're going to say take the rates from the data object.
00:15:25 And using this syntax, we immediately get the rates and now we can do some logic with them.
00:15:30 So what logic are we going to do?
00:15:32 Well, since euro is our base currency, we're going to create euro there.
00:15:37 And we're going to say one divided by and now we have defined the currency want to exchange from in the rates array.
00:15:46 So we're going to say rates and then from currency.
00:15:49 Now, what is this going to be equal to?
00:15:51 Let's see.
00:15:52 we get 0.84 which means that one US dollar is equal to 0.84 euros.
00:15:59 Great, now on the second line we have to calculate the exchange rate.
00:16:04 The exchange rate is going to be equal to euro times, now we have to find the currency we want to exchange to which is rates and then to currency.
00:16:15 In here, let's see what do we get with this nice syntax.
00:16:18 And as you can see, we again get 0.84 because we are transferring immediately to euros.
00:16:25 But let's try something like Australian dollar.
00:16:27 And if you can see correctly, we get 1.4. Great, that works.
00:16:33 The only thing this function is going to do is return the exchange rate.
00:16:37 So that's exactly what we're going to do.
00:16:39 We can get rid of these comments and that's it.
00:16:42 Amazing.
00:16:43 Using Quokka, we can immediately see what this function is returning.
00:16:46 You can see it is an asynchronous function because we have this then there, but once we call the dot then, then it's going to return 1.4. Now onto the
00:16:55 second function.
00:16:56 Fetching data about the countries, we can use the Australian dollar in.
00:17:00 The second function is going to be called getCountries and it's going to be equal to a function of course, to an error function.
00:17:08 It's going to accept one parameter which is going to be equal to a currency code, basically the three letter acronym for each country and then in there
00:17:17 we'll also try to fetch some data, this time data about the specific country or more specifically in which countries can a currency be used in.
00:17:27 So what can we do here?
00:17:29 Well, we of course get the response again.
00:17:31 And now we already learned once we are getting data from an asynchronous source, like an API, we have to put a weight right there.
00:17:39 And because of that, we also have to make that function asynchronous.
00:17:43 And then what did they tell you at the beginning?
00:17:45 What's the most important lesson of this video?
00:17:48 asynchronous functions return promises.
00:17:51 And you'll see what I mean by that at the end of this video.
00:17:54 Now we are awaiting axios that get call and then in there we're going to use a template string.
00:18:01 First thing in here is going to be the rest API countries link.
00:18:04 So that's going to be this one here.
00:18:07 And then we're going to do forward slash and then another pair of a template variable.
00:18:12 And it's going to be currency code.
00:18:15 So now this is giving us some data.
00:18:17 Let's see what data are we getting.
00:18:18 Again, with Qualcomm, it is extremely easy.
00:18:21 We have to call the function getCountries.
00:18:23 We're going to call it with something like Let's do Australian dollar.
00:18:27 We double click the response, we hover over it and we immediately get data.
00:18:32 Again, we are not concerned with the request data.
00:18:34 The only thing that we need is the data object inside of there so we can find it.
00:18:39 We don't even have to search for it because every Axios call always has that response data so we already know that it is in there.
00:18:47 If we try hovering over this, you can see we get all the countries that we can use the Australian dollar in, one of them being Antarctica.
00:18:55 I didn't know that.
00:18:57 Great.
00:18:58 We also have Australia and Christmas Island.
00:19:02 Great.
00:19:02 Amazing.
00:19:03 So now we're going to immediately destructure that data as we do here and get the same values of course, if you can see that.
00:19:11 That's it.
00:19:11 We again get array of countries with a lot of more values there.
00:19:15 But the only thing that we need from these countries is going to be just the name.
00:19:20 So to do that, we're going to map or this array.
00:19:23 So that's going to be data.map.
00:19:25 And in each thing, we get the country information, right?
00:19:29 Well, if we return the country, then again, we would get all of this because we are returning the whole object, we are not doing anything.
00:19:35 But we only want to return country.name.
00:19:40 And now if we actually hover over this, we should see only the names of the countries.
00:19:46 And you can see we get a nice looking array with all the countries right there.
00:19:50 Again, how easy it is with Quokka.js.
00:19:53 we can do a short destructuring there we're going to immediately destructure the name from the country and then just return the name right there just like
00:20:02 that and then if we check what data is equal to we should be able to see let's take a look yeah there we go we get everything but we don't want to look
00:20:11 for data we want to look for the thing that we stored our names in So that's gonna be a return and then data.map.
00:20:18 We are returning the array of country names from our getCountries function, just like that.
00:20:24 Again, if we put a comment, comment and question mark, we can see all the values there.
00:20:28 That does belong to a pro version, but you can see all the values right there.
00:20:32 If you're not getting any of this live data right there, make sure to call this function because only then, if you call it,
00:20:39 you can see that these lines appear right there.
00:20:42 Otherwise, it's not getting called.
00:20:44 With that said, we are ready to create our third function.
00:20:47 It's going to be called convert currency because it's going to be doing most of the work.
00:20:52 Convert currency.
00:20:54 And then right there, it's going to be an asynchronous function, which is going to accept all three things from currency,
00:21:03 to currency and it is also going to accept the amount of money we want to transfer.
00:21:08 That's going to be equal to an arrow function and then in there the first thing we're going to do is just one small thing that's going to help some users
00:21:17 with the input.
00:21:18 We're going to take that input called from currency and we're going to make it equal to the same input but we're going to call the to uppercase method
00:21:27 on it.
00:21:28 because if we enter right there at the start, if we tried entering the value of lowercase USD, let's see what do we get back.
00:21:37 If we hover over this, we get none, nothing number.
00:21:41 So this API cannot work with the values that are not uppercase.
00:21:45 It's good to have that in mind.
00:21:46 And we don't want the user to worry about that.
00:21:49 So that's why we fixed it right there.
00:21:51 And we're going to do the same thing for two currency as well.
00:21:55 Two currency.
00:21:57 Great.
00:21:58 Now we can start getting the data from these two APIs.
00:22:03 As we said, asynchronous functions return promises and they don't return the data that we want.
00:22:11 So to get to the data, we need to wait for the promise to be fulfilled.
00:22:15 So how does that work?
00:22:17 Well, we can see that this function right there, let's take this for example, getCountries.
00:22:21 This function returns an array of country names.
00:22:24 That's good.
00:22:25 So now if you want to get to the output of that thing, let's do something like const countries to store it in a variable.
00:22:32 is equal to and then we're going to call getCountries and we're going to provide it to currency because we want to get the countries for the currency we
00:22:42 exchanged in.
00:22:43 Great, so right now let's see what the countries are equal to.
00:22:47 We of course have to call the function convertCurrency and let's pass for example Australian dollar, let's pass the American dollar and then $20 just like that.
00:22:58 Now let's see what this value is going to be equal to.
00:23:02 you can see we do get the values here, but Quokka is really like in front of us all here.
00:23:08 It says then and then gives us the value.
00:23:10 It basically says what the value is going to be after we make the await keyword.
00:23:15 But this data isn't really here yet.
00:23:18 So now let's use that Konza log to see what countries are equal to.
00:23:23 As you can see, it is a promise.
00:23:25 Countries are not an array.
00:23:27 They're a promise that waits to resolve to actual countries.
00:23:32 I know it may sound complicated, but the only thing you have to do to get the actual data is put that await keyword in front of it.
00:23:40 And now you can see countries become actual countries.
00:23:44 That's it.
00:23:45 And then we can do the same thing to get the data for the exchange rates.
00:23:50 So we're going to say const exchange rate is going to be equal to a weight.
00:23:56 We call the function getExchangeRate and we pass in two parameters.
00:24:01 As we said, it's going to be from currency.
00:24:06 and two currency, just like that.
00:24:09 Now let's see what the exchange rate is going to be equal to.
00:24:12 I'm going to console log it just below.
00:24:13 And if we hover over it, we get 0.715. That's good.
00:24:19 We get the exchange rate.
00:24:20 Now what's happening with this thing right there is that we are first making the API call to this API, which takes time.
00:24:27 Let's say that we come into our code, we are here at second zero.
00:24:31 Then we wait for this request.
00:24:34 And then after it's done, basically, let's say that two seconds passed, I'm now exaggerating.
00:24:39 Then we have to also wait two seconds for this API call as well.
00:24:44 And then after we can finally console log something, four seconds have passed.
00:24:49 that is not ideal.
00:24:51 So using promises, we can do something much more efficient.
00:24:55 We're going to await for all the promises at once.
00:24:59 That means that we're going to call this thing at the same time as this thing.
00:25:04 How do we do that?
00:25:05 Well, we say await promise.all.
00:25:09 And then in there, we pass an array of functions we want to call.
00:25:14 So we're going to take these functions and then put them right there.
00:25:17 Now we're not going to get values in here.
00:25:20 So I'm going to delete these variables.
00:25:23 like that, but we will get values right here in front of it.
00:25:27 We can say const values is equal to a weight of this promise.
00:25:33 Great.
00:25:33 Now, since we're in an array, these are going to be commas and not semicolons.
00:25:40 and now what is the execution time of this well at the top we are at the zero second and then let's say that both of these calls take two seconds to go
00:25:50 now we saved double the time because both of these calls were being made simultaneously there is only one more thing that we have to do to make these calls
00:26:00 run simultaneously and that is removing the await keyword before each one.
00:26:05 When we had await we were first doing this one which took let's say two seconds and then we were waiting for the first one to finish and then we were calling
00:26:13 this one so that's two plus two is four as we said before but if you remove the await right there then these two are going to happen simultaneously and
00:26:22 we are only going to await for the response of both of them and there we get the values.
00:26:28 Now we are also going to use some array destructuring.
00:26:32 In here you can see we're consalogue in the countries and the exchange rate but we currently don't have them, we had them for each thing.
00:26:39 So now this thing is going to return an array where the first thing is going to be the response of the getExchangeRate function.
00:26:46 And what is the response of the exchange rate function?
00:26:49 Well, it is the exchange rate.
00:26:52 And then the second thing are countries, because countries are coming back from the getCountries function.
00:26:59 So this is a rate is structuring.
00:27:01 In here, you can name this anything you want.
00:27:03 And you can name this anything you want.
00:27:04 But the only important thing is that the get exchange rate, which is the first thing here in this array corresponds to the first index get countries in
00:27:12 the same way is now the first index.
00:27:14 So it's happening on the second place, we can now get rid of these comments, we just made our app so much more efficient.
00:27:21 Now we have to calculate the converted amount, we're going to do that by typing const, converted amount, which is going to be equal to parentheses amount
00:27:34 times the exchange rate.
00:27:35 So in here we have the exchange rate, and we're going to call the toFixed method on it, which is just going to have two decimal places.
00:27:43 Now, if we console log the converted amount, you can see on the right side, it is 14.33. If we remove this toFixed, it's going to be a longer number,
00:27:52 as you can see right there, it's meaningless, we just need two values.
00:27:56 and now using this converted amount we can finally return a string which is going to be meaningful to the user that's going to look something like this
00:28:05 we're going to have a string literal and then in there we're going to have amount from currency these are all variables dynamic variables is worth and
00:28:17 now converted amount to currency Let's finish this sentence, go into the next row and we can say, you can spend these in the following countries.
00:28:32 And then in there, we can say countries just like that.
00:28:37 And now finally, how are we going to retrieve the value from this function?
00:28:41 Well, since convert currency is an asynchronous function as well, we have to make sure to do the dot then on it.
00:28:49 So if you do that, then it accepts one callback function.
00:28:53 And in there, you get the value of the result.
00:28:56 So we're going to say a result right there.
00:28:58 And we're going to console.log.result.
00:29:01 And we can also change the dot catch on it.
00:29:05 Catch is going to happen if the error occurs.
00:29:08 So in there, we're going to say error and console.log the error, just like that.
00:29:14 Now let's see what do we get.
00:29:16 If you hover over this function, you can see we get that then but now let's try cons logging just the result or we can immediately hover over it you can
00:29:25 see 20 australian dollars is now worth 14.3 us dollars and you can spend this in the following countries There are a lot of countries so this is hard to read.
00:29:36 Let's try to transfer the dollars to Croatian Kunas because for a fact I know that there's only one country that you can use the Croatian Kuna in and now
00:29:46 if we try hovering that you can see we get 20 dollars is worth 126 Croatian Kunas.
00:29:52 You can spend this in the following countries, Croatia.
00:29:56 that's it now we get the values so to receive the value from an asynchronous function you have to chain a dot then and dot catch on it or you could do
00:30:06 the await syntax but for that we would have to be in another asynchronous function again and finally let's add try catch blocks to deal with error cases
00:30:17 in the first function something can go wrong of course we're going to wrap this whole thing in a try and catch block.
00:30:24 So right there, try catch.
00:30:26 And then we're going to put all of this successful data.
00:30:29 So that happens if everything goes successfully, if we do get a data, then we come here and return the exchange rate.
00:30:36 But in there, we're going to throw the error if we do any errors.
00:30:40 So we can say something like throw new error.
00:30:45 And the error is going to say, unable to get currency and then we can say which one from currency and to currency.
00:30:58 That's it.
00:30:58 Now if something goes wrong, if someone calls this function with wrong countries that don't exist, we are going to say unable to get currency from currency
00:31:07 and to currency.
00:31:08 As you can see, it is extremely easy adding some error handling using try and catch and that's because we are using async and await.
00:31:17 Great.
00:31:18 Now let's remove these comments and move to the second function.
00:31:22 Something can go wrong there as well.
00:31:24 So we're going to wrap this whole thing in a try and catch block that looks like this.
00:31:30 And we're going to again pull all the successful data at the top, cons data, return data.
00:31:36 And then in there, we're going to throw new error.
00:31:40 And that error can say unable to get countries that use and in here we can say currency code.
00:31:50 Just like that.
00:31:51 If we indent this properly, remove the spaces, remove the comments.
00:31:57 And now finally we have the convert currency.
00:32:00 Do we need some error handling here?
00:32:02 Well, not really.
00:32:03 If the error happens, then these functions are going to return the error and we're not going to get the final result.
00:32:09 And to see if everything is finally working, we're going to run it in the console.
00:32:13 We didn't need the console the whole time because we had Quokka, but now let's just test it out.
00:32:18 And I just remembered that this has to be const Axios is equal require Axios if we want to run this in node.
00:32:27 So we're going to do that.
00:32:29 And now we can open the terminal and run node index.js.
00:32:34 As you can see, we get $20 is worth 126 Croatian kunas.
00:32:39 You can now spend this in following countries, Croatia.
00:32:42 Let's try with a few more examples.
00:32:44 Let's try calling our function with American dollar and let's see Canadian dollar for example.
00:32:51 Recall it and we get $20 is worth 27 Canadian dollars.
00:32:56 You can use them in Canada.
00:32:57 Let's try something like Australian dollar.
00:33:01 And we get that 20 Australian dollars is worth 19 Canadian dollars.
00:33:05 That's pretty close.
00:33:07 Just before you go away from this video, post recording JavaScript Mastery is here.
00:33:12 Now I'm speaking after the video has been recorded, I noticed some things while I was editing.
00:33:17 First of all, for the last few minutes of the video, you might have noticed that we also had a wait right there.
00:33:23 That is a mistake.
00:33:24 As explained in the video, we don't have the await keyword inside of the promise that all calls.
00:33:30 We only have it before the promise that all.
00:33:33 And the last thing that I wanted to tell you is that if you're using node 14.3 or later, you have access to something called top level await.
00:33:43 That means that you should technically be able to create a variable here and then use the await keyword like this and then we should be able to get the
00:33:53 value of a result just like that.
00:33:55 As you can see we do get it.
00:33:57 Top level await is still kind of new so feel free to use the .ben and .catch without any troubles but if you want to be advanced,
00:34:04 if you want to google a bit and see how top level await works definitely give it a try.
00:34:09 That is it for this video.
00:34:11 We did quite a lot.
00:34:12 The application is as I said really really simple but extremely educational.
00:34:17 You'll learn how async await works, how to use the promise at all, how to do some destructuring, and you also heard about the phenomenal tool called Quokka.js.
00:34:27 If you haven't already, link is in the description, check it out.
00:34:31 that is it for this video if you have any more video ideas or topics you'd like me to explain feel free to ask in the description also if you'd like me
00:34:40 to do a video which explains all the details about what quokka offers please let me know in the comments in this video we explored only the main ones like
00:34:50 highlighting and the live code but there are many more great features that's going to be it for this video and see you in the next one