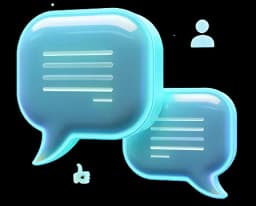
Join the Conversation!
Subscribing gives you access to the comments so you can share your ideas, ask questions, and connect with others.
In this lesson, you'll learn about promises in JavaScript, which provide a more readable and manageable way to handle asynchronous operations compared to callback functions. Promises help you avoid "callback hell" and make it easier to handle both successful and failed asynchronous operations.
In the last lecture, we witnessed the challenges of callback hell. Promises come to the rescue by offering a cleaner and more structured way to handle asynchronous tasks.
They are objects that represent the eventual completion (or failure) of an asynchronous operation and its resulting value. Promises can either return the successfully fetched data or an error.
Let's create a simple promise:
// Creating the promise
const promise = new Promise((resolve, reject) => {
setTimeout(() => {
console.log('Got the user');
resolve({ user: 'Adrian' });
}, 2000);
});
// Getting the data from the promise
promise.then((user) => {
console.log(user);
});
Promises also make it easy to handle errors. You can replace with to simulate an error:
// Creating the promise
const promise = new Promise((resolve, reject) => {
setTimeout(() => {
console.log('Got the user');
// resolve({ user: 'Adrian' });
reject('Error');
}, 2000);
});
// Getting the data from the promise
// .then gives us the result of the resolve and .catch gives us the result of the reject
promise.then((user) => {
console.log(user);
}).catch((error) => {
console.log(error);
});
Let's refactor the previous callback example using promises:
console.log(1);
const fetchUser = (username) => {
return new Promise((resolve, reject) => {
setTimeout(() => {
console.log('Now we have the user');
resolve(username);
}, 2000);
});
}
const fetchUserPhotos = (username) => {
return new Promise((resolve, reject) => {
setTimeout(() => {
console.log('Now we have the photos');
resolve(['photo1', 'photo2']);
}, 2000);
});
}
const fetchPhotoDetails = (photo) => {
return new Promise((resolve, reject) => {
setTimeout(() => {
console.log('Now we have the photo details');
resolve('details...');
}, 2000);
});
}
// Calling the functions with promises
fetchUser('Adrian')
.then((user) => fetchUserPhotos(user))
.then((photos) => fetchPhotoDetails(photos[0]))
.then((detail) => console.log(detail))
.catch((error) => console.log(error));
console.log(2);
In the next lesson, you'll learn about , a recent addition to JavaScript that builds on promises to make asynchronous code even more intuitive.
"Please login to view comments"
Subscribing gives you access to the comments so you can share your ideas, ask questions, and connect with others.