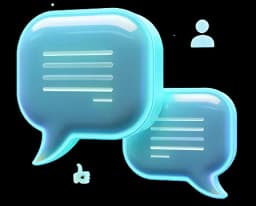
Join the Conversation!
Subscribing gives you access to the comments so you can share your ideas, ask questions, and connect with others.
In this lesson, you'll learn about classes in JavaScript, which provide a structured way to create objects with shared properties and methods. Classes are a key feature of object-oriented programming, allowing you to define blueprints for objects.
A class in JavaScript is a blueprint for creating objects. It defines the properties and methods that the objects created from the class will have. This makes it easy to create multiple objects with the same structure.
To define a class, you use the keyword followed by the class name. Inside the class, you define a method, which is used to initialize the object's properties.
class Person {
constructor(name, age, working) {
this.name = name;
this.age = age;
this.working = working;
}
}
Once you have defined a class, you can create instances of it using the keyword. This creates a new object with the structure defined by the class.
let user = new Person("John", 24, true);
console.log(user);
Using classes allows you to create multiple objects with the same structure without having to manually define each one. This is especially useful when you need to create many similar objects, such as users or products.
let user1 = new Person("Alice", 30, false);
let user2 = new Person("Bob", 28, true);
console.log(user1); // Person {name: "Alice", age: 30, working: false}
console.log(user2); // Person {name: "Bob", age: 28, working: true}
Classes in JavaScript provide a powerful way to define and manage objects with shared properties and methods. By using classes, you can create clean and maintainable code, making it easier to manage complex applications. Understanding how to define and use classes is essential for effective object-oriented programming in JavaScript.
"Please login to view comments"
Subscribing gives you access to the comments so you can share your ideas, ask questions, and connect with others.