Lifetime access to this course
Writing Small Functions
In this lesson, you'll explore the benefits of writing small functions in JavaScript and how they contribute to clean, maintainable code. Small functions are a cornerstone of good software design, promoting readability, reusability, and ease of testing. Let's dive into why small functions matter and how to effectively implement them in your code. ## Small Functions ### Do One Thing (DOT) Principle The Do One Thing principle, often referred to as Curly’s Law, suggests that functions should perform a single task. This approach ensures that each function is focused and easy to understand. A function should either perform an action or return a result, but not both.
The rest of this lesson is waiting.
Join JS Mastery Pro to unlock it.
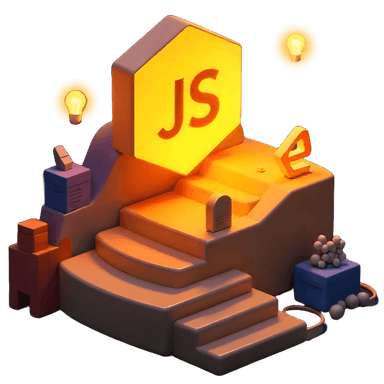
Complete Path to JavaScript Mastery
$300
Buy the Course OnlyBuild advanced web app
Certificate of completion included
Great for focused learners who just want this course
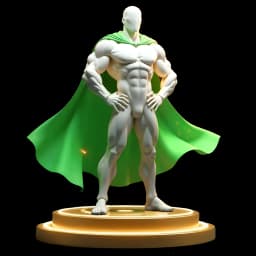
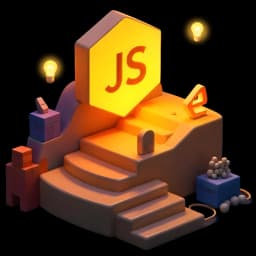
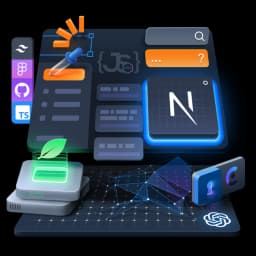
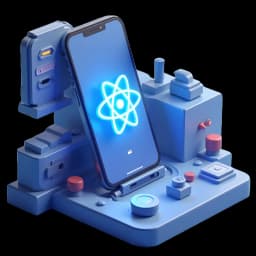
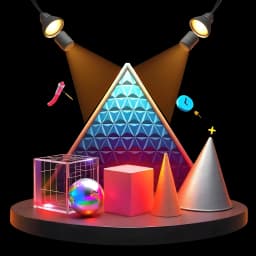
Get Full Access
$120 /month
Lock in a year & save—2 months free!
Access to all courses
Quizzes, mini-challenges, AI summaries, inline sandboxes
Participation in a private community
Build real production-grade apps
Mock interviews & project reviews
Weekly live sessions & member-only access