Lifetime access to this course
Creating, Traversing and Removing Nodes
In this lesson, you'll learn about creating, traversing, and removing nodes in the DOM using JavaScript. These operations are fundamental for dynamically manipulating web pages. ### Creating Nodes JavaScript provides several ways to create HTML elements dynamically. The most commonly used method is document.createElement . ### Creating Elements
The rest of this lesson is waiting.
Join JS Mastery Pro to unlock it.
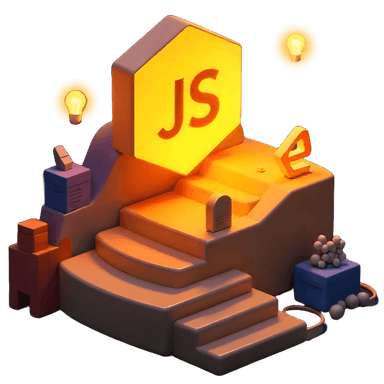
Complete Path to JavaScript Mastery
$300
Buy the Course OnlyBuild advanced web app
Certificate of completion included
Great for focused learners who just want this course
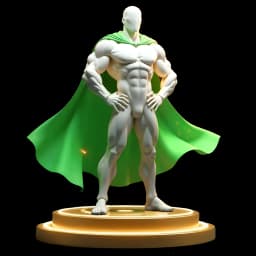
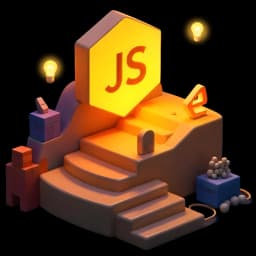
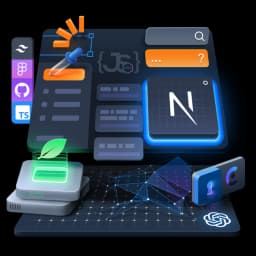
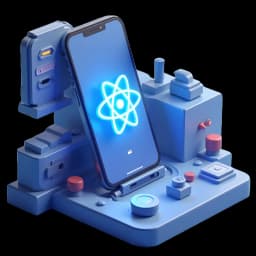
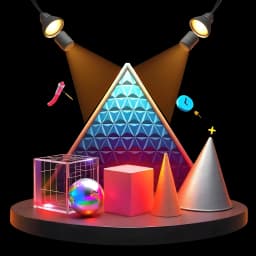
Get Full Access
$149 /month
Lock in a year & save—2 months free!
Access to all courses
Quizzes, mini-challenges, AI summaries, inline sandboxes
Participation in a private community
Build real production-grade apps
Mock interviews & project reviews
Weekly live sessions & member-only access