Lifetime access to this course
Selecting Elements
In this lesson, you'll learn about selecting elements in the DOM using JavaScript, which is a fundamental skill for manipulating and interacting with web pages. ## Selecting Elements One of the key tasks in JavaScript is to modify or manipulate existing elements on a web page. To do this, you first need to select the elements you want to work with. There are several methods to select elements in the DOM. ### 1. Finding Elements by ID
The rest of this lesson is waiting.
Join JS Mastery Pro to unlock it.
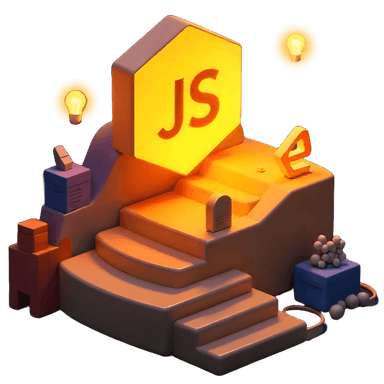
Complete Path to JavaScript Mastery
$300
Buy the Course OnlyBuild advanced web app
Certificate of completion included
Great for focused learners who just want this course
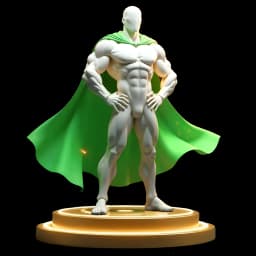
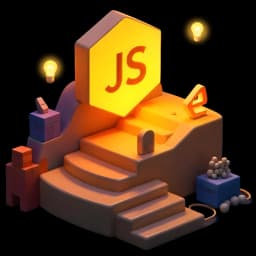
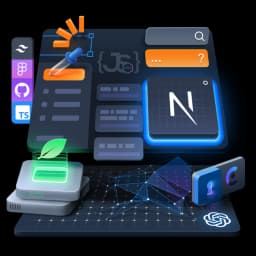
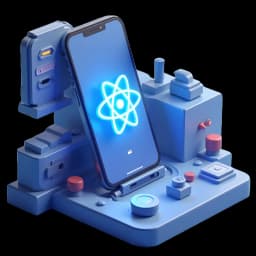
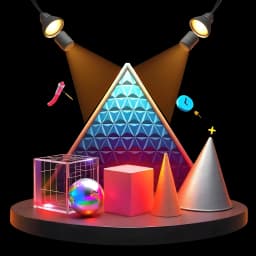
Get Full Access
$120 /month
Lock in a year & save—2 months free!
Access to all courses
Quizzes, mini-challenges, AI summaries, inline sandboxes
Participation in a private community
Build real production-grade apps
Mock interviews & project reviews
Weekly live sessions & member-only access