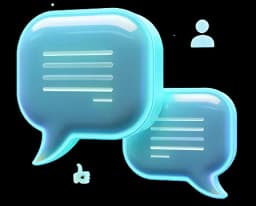
Join the Conversation!
Subscribing gives you access to the comments so you can share your ideas, ask questions, and connect with others.
In this lesson, you'll learn about working with classes in JavaScript, which are not only used for styling elements but also for manipulating them dynamically.
While classes are often associated with styling elements using CSS, they can also be leveraged in JavaScript to perform actions on elements with specific classes. This allows for dynamic interactions and modifications on a web page.
Classes are commonly used to apply styles to elements. Multiple class names can be assigned to an HTML element, separated by spaces. Here's an example:
<head>
<style>
.menu-item {
background-color: black;
color: white;
}
.menu-item.active {
background-color: white;
color: black;
}
</style>
</head>
<body>
<ul>
<li class="menu-item">Menu 1</li>
<li class="menu-item active">Menu 2</li>
<li class="menu-item">Menu 3</li>
</ul>
</body>
To interact with elements based on their classes, you can use JavaScript. For example, you might want to add an active class to a menu item when it's clicked and remove it from others.
Here's how you can modify the previous example to achieve this behavior:
<head>
<style>
.menu-item {
background-color: black;
color: white;
}
.menu-item.active {
background-color: white;
color: black;
}
</style>
<script>
function menuClicked(currentElement) {
const menuItems = document.getElementsByClassName("menu-item");
for (var i = 0; i < menuItems.length; i++) {
menuItems[i].classList.remove('active');
}
currentElement.classList.add("active");
}
</script>
</head>
<body>
<ul>
<li class="menu-item" onclick="menuClicked(this)">Menu 1</li>
<li class="menu-item active" onclick="menuClicked(this)">Menu 2</li>
<li class="menu-item" onclick="menuClicked(this)">Menu 3</li>
</ul>
</body>
Understanding how to work with classes in JavaScript is crucial for creating interactive and dynamic web pages. By combining CSS and JavaScript, you can enhance user experiences and create more engaging interfaces .
"Please login to view comments"
Subscribing gives you access to the comments so you can share your ideas, ask questions, and connect with others.