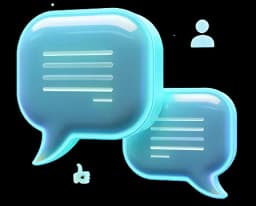
Join the Conversation!
Subscribing gives you access to the comments so you can share your ideas, ask questions, and connect with others.
In this lesson, you'll learn about arrow functions in JavaScript, which provide a modern and concise way to define functions.
Arrow functions have a key difference from "normal" functions : they do not create their own value.
The keyword is a special JavaScript reserved keyword, which we'll explain in more detail later. For now, just know that arrow functions inherit from their surrounding context. In most cases, this behavior is beneficial, so you'll often see arrow functions used in JavaScript code.
Here's how you can declare a function using arrow function syntax:
const square = (number) => {
return number * number;
}
Arrow functions also have a shorter and more concise version. Whenever a function consists of a single , you can write it in one line:
const square = (number) => number * number;
Practically, this means that people use arrow functions when they want to maintain the same context as the surrounding code.
Arrow functions are a powerful tool in JavaScript, especially when you want to write clean and concise code. As you become more familiar with them, you'll find them to be an invaluable part of your JavaScript toolkit.
"Please login to view comments"
Subscribing gives you access to the comments so you can share your ideas, ask questions, and connect with others.
How did you manage to remove the blur property and reach here?
Upgrading gives you access to quizzes so you can test your knowledge, track progress, and improve your skills.