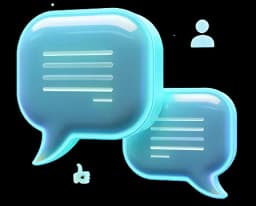
Join the Conversation!
Subscribing gives you access to the comments so you can share your ideas, ask questions, and connect with others.
In this lesson, you'll learn best practices for naming functions in JavaScript, ensuring that your code is readable, maintainable, and follows industry standards.
Choosing good function names is one of the most underrated yet important aspects of writing clean JavaScript. A well-named function makes your code self-explanatory and easy to understand without requiring excessive comments.
In JavaScript, function names should:
Let’s dive into some best practices for naming functions! 🚀
A function should do something, so its name should start with a verb that describes what it does.
function getUserData() {} // Retrieves user data
function sendEmail() {} // Sends an email
function calculateTotal() {} // Calculates total price
function fetchPosts() {} // Fetches posts from an API
function userData() {} // Not clear what this does
function email() {} // Is it sending, receiving, or formatting an email?
function total() {} // What about the total? Calculating it? Displaying it?
JavaScript follows the camelCase naming convention for function names.
function getUserInfo() {}
function calculatePrice() {}
function sendNotification() {}
function GetUserInfo() {} // ❌ PascalCase (used for classes, not functions)
function calculate_price() {} // ❌ Snake_case (not standard in JavaScript)
function sendnotification() {} // ❌ Hard to read
Function names should be short yet meaningful. Avoid unnecessary words while keeping them clear.
function fetchOrders() {} // Clear and concise
function validateInput() {} // Describes the function’s role
function startGame() {} // Makes it obvious what the function does
function fetchAllUserOrdersFromDatabaseTable() {} // ❌ Too long and detailed
function doStuff() {} // ❌ Too vague, what does it actually do?
function handleClick() {} // ❌ Generic, better to specify: handleButtonClick()
A great way to improve clarity is to use common function prefixes that indicate the function’s purpose.
function getUserProfile() {}
function fetchOrders() {}
function retrieveSettings() {}
function updateProfile() {}
function modifyCart() {}
function setTheme() {}
function isUserLoggedIn() {}
function hasPermission() {}
function canAccessPage() {}
function handleFormSubmit() {}
function onButtonClick() {}
function processPayment() {}
Avoid generic function names like , , or . These don’t provide any useful information about what the function actually does.
function processInfo() {} // What info? Processing it how?
function handleStuff() {} // What "stuff" is being handled?
function processUserLogin() {}
function handleFileUpload() {}
If a function returns a boolean value (true/false), use prefixes like:
function isUserAdmin() { return true; }
function hasValidToken() { return false; }
function canAccessDashboard() { return true; }
function userAdmin() {} // ❌ Doesn't indicate it returns true/false
function checkToken() {} // ❌ Not clear if it returns a boolean
function editProfile() {} // ❌ Sounds like an action, not a check
If you're working in a team or on a large project, keep function names consistent. If you use for one API call, use it for others too.
function fetchUsers() {}
function fetchPosts() {}
function fetchComments() {}
function getUsers() {}
function retrievePosts() {}
function loadComments() {}
Write function names in full words instead of abbreviations or unclear shorthand.
function calcPr() {} // ❌ What does "Pr" stand for?
function getUdt() {} // ❌ Too cryptic
function calculatePrice() {}
function getUserData() {}
Naming functions well is one of the easiest ways to write clean, readable JavaScript code. A function name should tell you exactly what the function does—without needing to read its implementation! If you follow these best practices, your JavaScript code will be much easier to read, maintain, and debug. Now that you know how to name functions properly, let’s move on to writing better, more efficient functions in JavaScript! 🚀
"Please login to view comments"
Subscribing gives you access to the comments so you can share your ideas, ask questions, and connect with others.
How did you manage to remove the blur property and reach here?
Upgrading gives you access to quizzes so you can test your knowledge, track progress, and improve your skills.