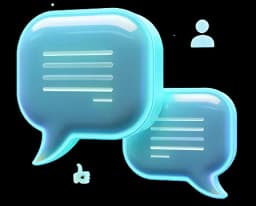
Join the Conversation!
Subscribing gives you access to the comments so you can share your ideas, ask questions, and connect with others.
In this lesson, you'll learn about declaring and invoking functions in JavaScript, which are essential for structuring and executing code efficiently.
As we learned in the last section, functions are subprograms designed to perform a particular task.
The code of the function is executed when the function is called. This is known as invoking a function. Let's first revisit the process of creating or defining a function.
There are a few different ways to define a function in JavaScript:
A Function Declaration defines a named function. To create a function declaration, you use the keyword followed by the name of the function.
function name(parameters) {
// statements
}
A Function Expression defines a named or anonymous function. An anonymous function is a function that has no name. In the example below, we are setting the anonymous function object equal to a variable.
let name = function(parameters) {
// statements
}
An Arrow Function Expression is a shorter syntax for writing function expressions.
const name = (parameters) => {
// statements
}
Arrow functions are the most modern way to create JavaScript functions. For that reason, we're going to explore them in much more detail in the upcoming lessons! 😊
Functions execute when they are called. This process is known as . You can invoke a function by referencing the function name, followed by an open and closed parenthesis: .
Let's explore an example.
First, we’ll define a function named . This function will take one parameter, . When executed, the function will log that back to the console:
function sayHi(name) {
console.log(`Hi, ${name}`);
}
To our function, we call it while passing in the singular argument. Here I am calling this function with the name :
sayHi('Joe'); // Hi, Joe
Notice how we got back the console log, but we also got back . Why is that? That is connected to the return of the function. If a function does not explicitly return a value, it returns by default. We'll explore this concept further in upcoming lessons.
"Please login to view comments"
Subscribing gives you access to the comments so you can share your ideas, ask questions, and connect with others.
How did you manage to remove the blur property and reach here?
Upgrading gives you access to quizzes so you can test your knowledge, track progress, and improve your skills.
By logging in, you'll unlock full access to this and other free tutorials on JSM Pro.
Why? Logging in lets us personalize your learning experience, track your progress, and keep you in the loop with new workshops, coding tips, and platform updates.
You'll also be the first to know about upcoming launches, events, and exclusive discounts.
No spam—just helpful content to level up your skills.
If that sounds fair, go ahead and log in to continue →
Enter your name and email to get instant access
##Looks like we found a thief monkey By the way, I liked the trick how you reached till here. You have a good sense of humor. You will improve a lot if you join our course with this passion.
var
(function-scoped, outdated)let
(block-scoped, modern and recommended)const
(block-scoped, cannot be reassigned)_
, or $
let let = 5;
is invalid)myVar
and myvar
are different)string
, number
, boolean
, null
, undefined
, bigint
, symbol
Objects
, Arrays
, Functions
Subscribing gives you access to a brief, insightful summary of each lecture to stay on track.
00:00:00 As I mentioned in the last lesson, functions are sub-programs designed to perform a specific task.
00:00:07 The code of the function is executed when the function is called.
00:00:11 You know that by now.
00:00:12 This is also known as invoking a function.
00:00:15 So let's first revisit the process of creating or defining a function.
00:00:20 Because there are a few different ways in which we can define a function in JavaScript.
00:00:25 First, there is a typical function declaration.
00:00:30 We saw it already.
00:00:32 You say function, define a name of the function, pass in some params, and then open up a function block.
00:00:39 Pretty simple, right?
00:00:40 But there is also something known as a function expression.
00:00:45 It looks like this.
00:00:46 You can say let.
00:00:48 define the name of the function and make it equal to what appears to be a function call, but in this case, it's a function expression where you can pass
00:00:58 some params and then open up a block of code.
00:01:01 So this is basically the same thing as above, but just done in a bit of a different way.
00:01:06 With a function declaration, you have to pass a name.
00:01:10 But with the function expression allows you to specifically define a function name and store it to a variable to be called later.
00:01:17 Or you can also define it as anonymous function.
00:01:20 An anonymous function is a function that has no name.
00:01:23 So that is this one right here.
00:01:25 And we're setting that function to equal to the name variable.
00:01:29 Still, both of them will be called in the same way, just like this.
00:01:33 And finally, there is a third way to declare functions, and it is called an arrow function expression, or simply called arrow functions.
00:01:45 It looks like this.
00:01:46 const name is equal to.
00:01:50 a pair of parentheses where you pass the params, and then you put an equal sign and a greater than sign to form an arrow.
00:02:00 And then you can open up a new block of code like this.
00:02:03 Once again, it is very similar to the two ways above, but it is just a new syntax that allows you to write it in a shorter way.
00:02:10 Arrow functions are the most modern way of writing JavaScript functions.
00:02:14 So for that reason, we're going to explore them in much more detail in the upcoming lessons.
00:02:18 Just so we don't get this error, I'll name these functions func1, func2, and func3.
00:02:26 And you can try calling them, or in other words, invoking them.
00:02:30 You already know how to do that.
00:02:31 You define a function name, and then you open up a pair of parentheses.
00:02:36 Let's make this function actually do something.
00:02:39 I'll call it sayHi.
00:02:42 And I'll take in a name and then within it, we can just put a console log and say something like hi, and then pass over a name.
00:02:51 So now you can call this function by calling say hi.
00:02:54 And then if you call it without any parameters, you'll see hi undefined.
00:03:01 Okay.
00:03:01 This is a pretty interesting way to see the undefined data type in the wild.
00:03:05 Why did it happen?
00:03:06 Well, that's because we didn't pass any arguments to fill up the name param.
00:03:10 But if you pass something like, hi there, John, it'll say hi, John.
00:03:17 Now, another quick question for you.
00:03:18 Why didn't we have to add a return keyword from this function?
00:03:23 Well, that's because the function itself automatically console-lock the output.
00:03:28 But a more typical use case would be to return this string right here, this template string, And then you would be able to pass the name,
00:03:39 but then you have to store that.
00:03:41 So you'd have to say something like const greeting is equal to the function call of the sayHi function with the argument of John.
00:03:49 And then you would be able to console log this greeting.
00:03:55 There we go.
00:03:56 You can also modify it, but you can see now the output of the function changes based on the param that you passed right here.
00:04:04 Now, why did we test this arrow function and not the other two ways of writing functions?
00:04:08 Even though you'll see all three of these ways in the wild, you'll see arrow functions most often.
00:04:14 In the next lesson, let's dive a bit deeper into explaining the return statement of a function.
00:04:19 Maybe the function's most important part.