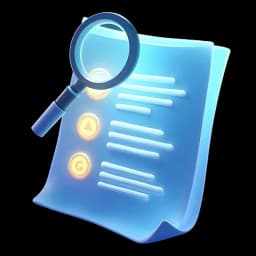
Quick Lecture Overview
Subscribing gives you access to a brief, insightful summary of each lecture to stay on track.
In this lesson, you'll learn about the return statement in JavaScript functions, which is crucial for understanding how functions output values and control flow.
Every function in JavaScript returns unless otherwise specified. That means if we don't explicitly specify what we want our function to return , it will always return .
Let's create a function called . It will take in one parameter, called , and return that number multiplied by two. Don't worry about arrow function syntax too much; we'll cover it in greater detail in the next lesson! 😊
Here's a simple function that returns the sum of numbers and :
function add(a, b) {
return a + b;
}
This function will return the sum of and . We can then invoke the function and save the to a variable:
const sum = add(2, 2);
When we log out our value, we get :
console.log(sum); // 4
Awesome! The statement not only returns values from a function but also assigns them to whatever called the function!
Another important rule of the statement is that it stops function execution immediately.
Consider this example where we have two statements in our function:
function test() {
return true;
return false;
}
test(); // true
The first statement immediately stops execution of our function and causes it to return . The code on line three, ; is never executed.
We can also test it by adding some console logs:
function test() {
console.log(1);
return true;
console.log(2);
return false;
console.log(3);
}
test(); // true
As you can see, we only get the console log with the value of . As soon as the function returns something, it doesn't care about anything else. It's done its job, and now other JavaScript code can be run.
In the next lesson, we're going to explore , the modern way of writing JavaScript functions.
By logging in, you'll unlock full access to this and other free tutorials on JSM Pro.
Why? Logging in lets us personalize your learning experience, track your progress, and keep you in the loop with new workshops, coding tips, and platform updates.
You'll also be the first to know about upcoming launches, events, and exclusive discounts.
No spam—just helpful content to level up your skills.
If that sounds fair, go ahead and log in to continue →
Enter your name and email to get instant access
##Looks like we found a thief monkey By the way, I liked the trick how you reached till here. You have a good sense of humor. You will improve a lot if you join our course with this passion.
var
(function-scoped, outdated)let
(block-scoped, modern and recommended)const
(block-scoped, cannot be reassigned)_
, or $
let let = 5;
is invalid)myVar
and myvar
are different)string
, number
, boolean
, null
, undefined
, bigint
, symbol
Objects
, Arrays
, Functions
Subscribing gives you access to a brief, insightful summary of each lecture to stay on track.
00:00:00 you need to understand how the return statement in JavaScript function works.
00:00:05 Not only to be able to know how the function's output values, but also to understand the control flow of the function.
00:00:13 See, every function in JavaScript returns undefined unless otherwise specified.
00:00:18 That means that if you don't explicitly specify what we want our function to return, it'll always return undefined.
00:00:25 Let's create a function called add.
00:00:28 You can use the function declaration or an arrow function.
00:00:32 I'll say const add, and I'll make it accept two parameters, a and b.
00:00:39 We can create an arrow and then open up a function block.
00:00:43 And I'll make it return a plus b.
00:00:46 Now, as you know, you can create a new variable called sum and make it equal to a function call where you can pass two arguments.
00:00:55 Let's say two plus two, and then you can console log the output.
00:01:01 that'll look something like this, and you're going to get back four.
00:01:04 But now what's super important to learn here is that as soon as you return from a function, its execution stops.
00:01:12 What if you have more things happening right here?
00:01:14 For example, let's say that you're returning just the keyword true.
00:01:19 But then later on, you do some logic and then you want to return false.
00:01:24 So now let's rename this function to return booleans.
00:01:27 That seems like a valid name and it doesn't have to accept any parameters in this case.
00:01:32 And we'll rename the output to just result.
00:01:36 Now, what do you think the output will be?
00:01:38 Return true and then return false.
00:01:41 That would make sense, right?
00:01:43 Well, if we save it, you'll notice that it only returns true.
00:01:49 That's because of a very important function rule, and that is that the return statement stops function execution immediately.
00:01:58 So as soon as you return out of the function, you cannot get back into it unless you recall it.
00:02:04 And our editor even says that.
00:02:06 It says unreachable code detected.
00:02:08 So, the first return statement immediately stops the execution of the function and causes it to return true.
00:02:15 And the code on line 3, return false, is never executed.
00:02:19 You can also test that by adding some consul logs.
00:02:22 I'll add a consul log of 1, then below it I'll add a consul log of 2, and then I'll add a consul log of 3. Which ones do you think we'll get?
00:02:33 Well, as you can see, we only get a console log of 1, because after we return, nothing else in the function happens.
00:02:40 It doesn't care about anything else.
00:02:42 Its job is done, and now other JavaScript code can run.
00:02:46 Oh, that's a good point to mention.
00:02:48 See, this function code is stored right here from line 1 to line 7, and we can even collapse it.
00:02:55 But JavaScript typically runs linearly, from top to bottom.
00:02:59 But in this case, it'll notice that this is a function declaration, and it won't immediately execute the code.
00:03:06 Rather, only when it sees a call, it'll start executing it.
00:03:10 And then when it finishes, it'll continue executing it below.
00:03:14 But with that in mind, now we know a bit more about the function's return statement and how if you don't explicitly pass it,
00:03:21 by default, every function just returns undefined.
00:03:24 How you can explicitly define specific values to be returned and how we can store them to a variable.
00:03:30 And most importantly, how return statements also affect the function's control flow.
00:03:36 Now, remember that I told you that I'll tell you a bit more about arrow functions.
00:03:41 I showed you a square example, where we declare an arrow function called square that takes in a number, multiplies it, and returns it.
00:03:50 Typically, functions have many more blocks of code, like a console log here, maybe another variable right here, and a lot of stuff happening.
00:03:59 But in very simple situations, where the only thing that this function has is just a single return statement, there's another alternative way to write
00:04:10 an arrow function with an even shorter and more concise version.
00:04:14 So whenever a function consists only of a single return statement, and only then, you can write it in one line like this.
00:04:22 By completely removing the curly braces, as well as the return keyword, because then this is considered an automatic return.
00:04:32 And let me show you that that indeed works by defining a result, making it equal to the call of the square.
00:04:39 Of course, make sure to console log the result.
00:04:41 And as you can see, we get back the number 25. So you don't necessarily have to open up a block, say return, and only there return those values,
00:04:52 which takes three lines.
00:04:53 If it is super simple, you can just remove the curly braces and return it in a single line.
00:04:59 Even though this might seem crazy right now, like why would I need to do this?
00:05:03 There are many great use cases for this that simplify your code and make it more readable.