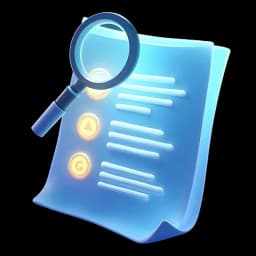
Quick Lecture Overview
Subscribing gives you access to a brief, insightful summary of each lecture to stay on track.
In this lesson, you'll learn about functions in JavaScript, which are essential for organizing and reusing code efficiently.
In this section, we're going to talk about functions. I'm really excited to show you how functions work! 😊 Functions are one of the most interesting and important parts of any programming language.
So what are functions, and why should we use them? A JavaScript function is a block of code designed to perform a particular task. Remember that: a block of code designed to perform a particular task. We often need to perform similar tasks many times in our application.
Functions are the main building blocks of a program. They allow the code to be called many times without repetition.
You've already seen a function in JavaScript. Not only have you seen it, but you've also used it multiple times by now. It was the function called . has the task of printing values to the console. After finishing this section, you'll be able to create your own functions as well! 😊
Now, let's dive right in!
When talking about functions, you're often going to hear two terms: and . So let's explain each one of these.
Function: A function is a special value with one purpose: it represents some code in your program. Functions are handy if you don’t want to write the same code many times. Calling a function like tells the computer to run the code inside it and then go back to where it was in the program. There are many ways to define a function in JavaScript, with slight differences in what they do.
A consists of:
I'm first going to show you an example of a simple function called :
function square(number) {
return number * number;
}
Let's break it down:
Let's break it down:
The function body, enclosed in curly braces {}, contains the code to be executed. In this example, it returns the parameter multiplied by itself.
The statement is crucial; it specifies the value that will be returned by the function. To retrieve values from functions, we need to call them.
a function does not it. Defining it simply names the function and specifies what to do when the function is called.
the function actually performs the specified actions with the indicated parameters. For example, if you define the function , you could call it as follows:
square(5);
Here, we have the function name followed by parentheses. Inside the parentheses, we put .
Arguments are the values we want to pass to our parameters.
For example, if we send the of , the parameter becomes . The function then multiplies it by itself and returns the result.
As you can see, we get . Exactly as expected.
To use the from the function, we can store it in a :
const result = square(5);
This stores the return value of the function (called with an argument of ) in the variable. Now we can log it to the console:
console.log(result);
By logging in, you'll unlock full access to this and other free tutorials on JSM Pro.
Why? Logging in lets us personalize your learning experience, track your progress, and keep you in the loop with new workshops, coding tips, and platform updates.
You'll also be the first to know about upcoming launches, events, and exclusive discounts.
No spam—just helpful content to level up your skills.
If that sounds fair, go ahead and log in to continue →
Enter your name and email to get instant access
##Looks like we found a thief monkey By the way, I liked the trick how you reached till here. You have a good sense of humor. You will improve a lot if you join our course with this passion.
var
(function-scoped, outdated)let
(block-scoped, modern and recommended)const
(block-scoped, cannot be reassigned)_
, or $
let let = 5;
is invalid)myVar
and myvar
are different)string
, number
, boolean
, null
, undefined
, bigint
, symbol
Objects
, Arrays
, Functions
Subscribing gives you access to a brief, insightful summary of each lecture to stay on track.
00:00:00 In this module, we're going to talk about functions.
00:00:04 I'm really excited to show you how they work as functions are one of the most interesting and most important parts of any programming language.
00:00:13 So what are they and why should we use them?
00:00:16 Well, a JavaScript function is a block of code designed to perform a particular task.
00:00:22 Remember that, a block of code designed to perform a task.
00:00:28 We often need to perform different tasks many times in our applications, and functions are the main building blocks of a program.
00:00:36 They allow the code to be called many times without repetition.
00:00:40 Believe it or not, you've already seen a function in JavaScript.
00:00:44 Not only have you seen it, but you've also used it yourself many times throughout this course.
00:00:50 It's a function called ConsoleLog.
00:00:53 ConsoleLog is a function that has the task of printing values to the console.
00:00:58 After we complete this module, you'll be able to create your own functions as well.
00:01:02 So, let's dive right in.
00:01:05 When talking about functions, you're often going to hear two terms — function declaration and function call.
00:01:12 So, let me explain each one of these.
00:01:14 A function declaration consists of the function keyword, followed by the function name, and then a list of parameters enclosed in parentheses,
00:01:23 and a block of code enclosed in curly braces.
00:01:26 Let me show you an example of a very simple function declaration.
00:01:30 As I mentioned, we start with the keyword function.
00:01:34 Then you define a function name, such as square, if we want to get the square of a number.
00:01:40 Then you define a list of parameters.
00:01:43 In this case, I'll only define one parameter called number, and you open up a function block with curly braces.
00:01:50 Then you define what you want that function to do, In this case, I want it to return just a number multiplied by itself.
00:01:59 So return the square of that number.
00:02:01 Let's recap it one more time.
00:02:03 Function is the reserved JavaScript keyword for creating a function.
00:02:08 Square is the name of the function.
00:02:10 You can name it however you'd like.
00:02:12 And then parameters are inside of these parentheses.
00:02:16 These are the values that you send to your function when calling it.
00:02:21 In this case, the function square takes only one parameter called number, and you can name those parameters however you'd like.
00:02:28 For example, it can be A, B, C.
00:02:31 All of the rules that we talked about when naming variables apply here too.
00:02:35 Finally, we have the function body enclosed in curly braces, which contain the code that has to be executed.
00:02:42 The return statement here is crucial.
00:02:45 It specifies that the value will be returned from the function.
00:02:48 But when will the value be returned?
00:02:51 Or in other words, when will this function be executed?
00:02:55 Well, when we call it.
00:02:57 See, defining a function does not execute it.
00:03:01 Defining it simply names the function and specifies what should be done once the function is called.
00:03:08 But calling the function actually performs the specified actions within the specific parameters.
00:03:14 For example, if you define this function square, you can call it like this.
00:03:20 square, parentheses, and then you can see my code editor automatically tells me what I need to pass into it.
00:03:26 In this case, a number.
00:03:28 So if I pass the number 5, this means that I have just tried to call the function square with a parameter number of 5. The things that we put inside of
00:03:39 a function called parentheses are called arguments.
00:03:43 Arguments are the values that you pass into our parameters.
00:03:48 So two different names for a similar thing, but in different places within a function declaration.
00:03:56 This number right here is a parameter, but within a function call, we call it an argument.
00:04:02 Why do we have two different names?
00:04:04 Because we pass the argument of five, which is then used as a parameter within this function.
00:04:10 Now, so far we were able to test our code within our console, but nothing is getting returned.
00:04:18 If I save it, we don't see either 5 or 25. We don't see the output of the function.
00:04:25 Well, that's because even though we're calling it, we have to log it to the console.
00:04:29 So what you could do is just wrap this function call with a console.log that would look something like this, and you're going to get the output of 25.
00:04:39 But what's even better is if you stored the value or the result of the function call.
00:04:45 So to use the value from a function, we can store it in a variable by saying something like const result is equal to this function call.
00:04:56 This stores the return value of the square function called with the argument of five in the result variable.
00:05:04 And now we can more easily log it to the console by saying console log result.
00:05:10 And we indeed do get 25. So that my friend is a function.
00:05:15 There are a lot of new keywords and specific names to remember.
00:05:19 Function, return, parameters, arguments, calls and declarations.
00:05:25 And it might seem confusing right now, but trust me, it'll become second nature.
00:05:30 Some beginner developers struggle with understanding what is a function declaration and what is a function call.
00:05:36 That's exactly why I took my time with this Intro to Functions lesson to really drill it down to make sure you remember it.
00:05:43 And I will do it one more time.
00:05:45 A function declaration is a place where you first define your function.
00:05:51 Using the function keyword, you define a function name and you choose which parameters you want your function to accept.
00:05:59 Then you open up a function block and define what your function should do.
00:06:06 But that on itself just sets this to be executed later on within the code.
00:06:12 If you don't call it, nothing will happen.
00:06:14 So then comes the function call.
00:06:17 Function call happens when you use the function name and then put a pair of parentheses right here.
00:06:23 In case you want to pass some arguments into the function to fill up your params, you can do that within the parentheses.
00:06:31 And if you want to store the return value of your function, you can do that right here within a new variable that you'll create and make it equal to the
00:06:40 call of this function.
00:06:41 And then you can do whatever you want with it, such as const logit.
00:06:45 If this made sense, and if you like this a bit of a deeper explanation, please let me know in the comments below this lesson.
00:06:51 And if this seems like a very nice introduction to functions, feel free to leave a review on TrustPilot as well.
00:06:57 I'm looking forward to your feedback.
00:06:59 And in the next lesson, we'll dive a bit deeper into different ways in which you can declare your functions.