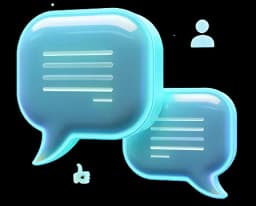
Join the Conversation!
Subscribing gives you access to the comments so you can share your ideas, ask questions, and connect with others.
In this lesson, you'll learn about the difference between and in JavaScript functions, which is crucial for understanding how data is passed to functions.
If you’re new to JavaScript, you may have heard the terms and used interchangeably. While very similar, there is an important distinction between these two concepts.
Let's revisit our example to illustrate this distinction. We would say that our function accepts one . Parameters can be named anything. The only thing that matters is the order. Let's try replacing with .
const sayHi = (firstName) => {
console.log(`Hi, ${firstName}`);
}
sayHi('Joe'); // Hi, Joe
Nothing changed; our function still works. This shows that are just names we create for the we're planning to pass into the function . As you can see, when calling the function, we have one . The argument is a real JavaScript value, in this case, a of .
Let's try adding another to practice a bit more. Suppose we want to take in both the name and the age of the person. To do that, we'd need to add a second , separated by a comma . We also need to provide an to fill the value of age when making a function call:
const logAge = (name, age) => {
console.log(`${name} is ${age} years old.`);
}
logAge('Joe', 25); // Joe is 25 years old.
Understanding the difference between and is key to effectively using functions in JavaScript. As you practice, you'll become more comfortable with how data flows through your functions .
"Please login to view comments"
Subscribing gives you access to the comments so you can share your ideas, ask questions, and connect with others.
How did you manage to remove the blur property and reach here?
Upgrading gives you access to quizzes so you can test your knowledge, track progress, and improve your skills.
By logging in, you'll unlock full access to this and other free tutorials on JSM Pro.
Why? Logging in lets us personalize your learning experience, track your progress, and keep you in the loop with new workshops, coding tips, and platform updates.
You'll also be the first to know about upcoming launches, events, and exclusive discounts.
No spam—just helpful content to level up your skills.
If that sounds fair, go ahead and log in to continue →
Enter your name and email to get instant access
##Looks like we found a thief monkey By the way, I liked the trick how you reached till here. You have a good sense of humor. You will improve a lot if you join our course with this passion.
var
(function-scoped, outdated)let
(block-scoped, modern and recommended)const
(block-scoped, cannot be reassigned)_
, or $
let let = 5;
is invalid)myVar
and myvar
are different)string
, number
, boolean
, null
, undefined
, bigint
, symbol
Objects
, Arrays
, Functions
Subscribing gives you access to a brief, insightful summary of each lecture to stay on track.
00:00:00 I already told you about parameters and arguments, right?
00:00:03 But if you're new to JavaScript, it's possible that you might use those terms interchangeably.
00:00:09 Parameters, arguments, does it matter?
00:00:12 And what is the difference?
00:00:13 Well, getting back to our past example, as I already told you, parameters are called parameters when they're used in a function declaration,
00:00:21 and they're called arguments when they're used in a function call.
00:00:24 A parameter is like a variable name that you give it.
00:00:28 like a meaningful name for a value within that function block, but you won't be able to access it outside.
00:00:37 So if you try to say console log number, you cannot access it.
00:00:41 It is undefined.
00:00:42 It is only accessible within that function block.
00:00:46 I can actually return it like this so we can see it better.
00:00:50 And of course, within here, we can console log that number, but outside of it, we can't.
00:00:57 So think of a parameter, like a variable that you can use within a function block.
00:01:02 And then think of an argument as the real value that you pass into the function when making a function call, like any kind of a data type,
00:01:12 like a string or a number or anything like that.
00:01:15 And I know I'm repeating myself, but heck, when I was a beginner, I remembered that I used to confuse those two terms.
00:01:21 So I want to make sure you got it right from the start.
00:01:24 Let me give you one more example.
00:01:26 Let's say you have a function, say hi, which we had before this time in form of an arrow function.
00:01:32 And this function will take in a parameter of first name and it'll immediately console log hi.
00:01:41 First name.
00:01:42 Now you can call that sayHi function and say, Adrian.
00:01:47 Hi, Adrian.
00:01:48 Now let's try adding another parameter to practice a bit more.
00:01:52 Maybe something like age.
00:01:55 And in this case, we can make this equal to, let's do 25. And we can use it right here and say first name is age years old.
00:02:06 So here we're practicing template strings too.
00:02:08 And you get the output.
00:02:09 So you get the idea.
00:02:11 You can add as many parameters as you want, and you can fill them in with any kind of data types that we learned before when you call the function.
00:02:19 So now that you know the basics of the functions, they're not that tough, are they?
00:02:25 And you might see some names that are used for functions so far, like sayHi, greet, and so on.
00:02:33 You'll notice that there's something in common with how we're naming these functions.
00:02:37 So let's discuss it in the next lesson.