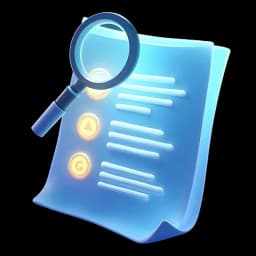
Quick Lecture Overview
Subscribing gives you access to a brief, insightful summary of each lecture to stay on track.
In this lesson, you'll learn about and loops in JavaScript, which are essential for repeating actions multiple times efficiently.
Sometimes we want to repeat an action a number of times. For example, let’s imagine we want to display numbers from zero to nine on the console. You might think of doing something like this:
console.log(0);
console.log(1);
console.log(2);
console.log(3);
console.log(4);
console.log(5);
console.log(6);
console.log(7);
console.log(8);
console.log(9);
But that’s not a good idea at all. Instead, we use or loops.
The loop is more complex, but it’s also the most commonly used loop. It’s called a loop because it runs "for" a specific number of times .
loops are declared with three optional expressions separated by semicolons: initialization, condition, and final-expression, followed by a statement (usually a block statement).
for ([initialization]; [condition]; [final-expression]) {
// statement
}
Let's use a loop to print numbers from zero to nine:
for (let i = 0; i < 10; i++) {
console.log(i);
}
Expressions in a loop are optional, meaning we can skip parts. For example, we can initialize the loop variable before the loop like this:
let i = 0; // we have i already declared and assigned
for (; i < 10; i++) { // no need for "initialization"
console.log(i); // 0, 1, 2, ..., 9
}
We can even remove everything, creating an infinite loop :
for (;;) {
// repeats without limits
}
The loop is simpler than the loop and is used when the number of iterations is not known beforehand. It continues to execute a block of code as long as a specified condition evaluates to .
while (condition) {
// statement
}
Let's use a loop to print numbers from zero to nine:
let i = 0;
while (i < 10) {
console.log(i);
i++;
}
A loop can also become an infinite loop if the condition never evaluates to . Be cautious to ensure that the loop has a way to terminate:
while (true) {
// This will run forever unless there's a break statement
}
By logging in, you'll unlock full access to this and other free tutorials on JSM Pro.
Why? Logging in lets us personalize your learning experience, track your progress, and keep you in the loop with new workshops, coding tips, and platform updates.
You'll also be the first to know about upcoming launches, events, and exclusive discounts.
No spam—just helpful content to level up your skills.
If that sounds fair, go ahead and log in to continue →
Enter your name and email to get instant access
##Looks like we found a thief monkey By the way, I liked the trick how you reached till here. You have a good sense of humor. You will improve a lot if you join our course with this passion.
var
(function-scoped, outdated)let
(block-scoped, modern and recommended)const
(block-scoped, cannot be reassigned)_
, or $
let let = 5;
is invalid)myVar
and myvar
are different)string
, number
, boolean
, null
, undefined
, bigint
, symbol
Objects
, Arrays
, Functions
Subscribing gives you access to a brief, insightful summary of each lecture to stay on track.
00:00:00 Sometimes, you might want to repeat an action a specific number of times.
00:00:05 For example, let's say you want to display numbers from 0 to 9 on the console.
00:00:10 Of course, your initial thought would be to say console.log and then display the 0. Then, you might want to duplicate it,
00:00:17 say 1, two, three, and so on.
00:00:21 But you can already see how this can take not only a lot of time, but also a lot of space within your terminal.
00:00:28 And if you don't know these handy keystrokes that I'm using right now, that allow me to duplicate a line very easily, it might take you much longer than
00:00:36 it took me.
00:00:37 But this is not a good idea at all.
00:00:40 Instead, you can use the for or while loops.
00:00:44 So, let's explore them one by one.
00:00:46 The for loop is more complex, but it's also the most commonly used loop.
00:00:51 It's called a for loop because it runs for a specific number of times.
00:00:56 For loops are declared with three optional expressions, separated by semicolons.
00:01:02 Initialization, condition, and final expression.
00:01:06 And then you can open up a block of code that'll be executed for a specific number of times.
00:01:12 The initialization is executed once before the loop starts.
00:01:17 It's typically used to define and set up your loop variable.
00:01:22 Then there's the condition.
00:01:24 It's evaluated at the beginning of every loop iteration, and the loop will continue as long as the condition evaluates the true.
00:01:33 But if the condition is false at the start of the iteration, the loop will stop executing.
00:01:39 And then there's the final expression.
00:01:41 It's executed at the end of each loop iteration, and it's usually used to either increment or decrement your loop counter.
00:01:49 Let me show you how we can use a for loop to print numbers from 0 to 9. We start with a 4, and you open up a block of code.
00:01:59 Next, we can have our initialization.
00:02:02 In this case, we can define a let i variable is equal to 0. That is the starting point where we initialize things.
00:02:11 In programming, we start counting from 0, so you'll see this used quite often.
00:02:16 And i stands for index, and it is a standard name for loop variables.
00:02:21 You could say something like a, b, c, index, or whatever you want, but you'll often see index as it's super simple and easy to understand.
00:02:31 Next, we need the condition.
00:02:33 I'll set the condition to i is lower than 10. Before each loop execution, it checks if i is less than 10, and if i is equal to or greater than 10, the
00:02:45 loop will terminate.
00:02:46 And then there's the final expression.
00:02:48 We can use i++.
00:02:50 This is the increment operator, or you can also use i is equal to i plus one, or i plus equal to one would work as well.
00:03:00 All of these are different ways of incrementing a number.
00:03:03 Finally, right here within the code block, we can just console log the i.
00:03:08 So if I save it, you can see the numbers from zero to nine printed right here to the console.
00:03:13 It works like this.
00:03:15 We first define i as 0, while i is less than 10, increment i, and then for each iteration, const log di.
00:03:24 So for the first one, it is 0, it logs it, then it is still less than 10, so increment it, and then log it.
00:03:33 Now it is 1, so 1 is still less than 10, increment it and then log it, it is 2, and then keep repeating that as long as this condition is true.
00:03:43 Now, keep in mind that expressions for a for loop are optional, which means that you can skip either one of these three expressions.
00:03:51 For example, you can initialize the loop variable before the loop, like this.
00:03:57 Let i is equal to zero, and then here, you don't need to provide anything, and it'll still work.
00:04:03 You can also remove absolutely everything, which will create an infinite loop, but trust me, you don't want to do that as it'll block your browser.
00:04:11 Good.
00:04:12 Now we know how the for loops work.
00:04:14 As a reminder, I'll leave this pseudo code right here, as well as an example where we're console logging the numbers from 0 to 9. And then there are while loops.
00:04:26 The while loop is simpler than the for loop, and you want to use it when you don't know for how many times you want to run that loop.
00:04:34 It will continue executing the block of code as long as the specified condition is true.
00:04:40 It looks something like this.
00:04:41 while condition, then just keep running this block of code.
00:04:47 Or we can try to perform the same thing as we had before, but now with a while loop.
00:04:52 You can do that by defining the variable of i right here, and then you can say while i is less than 10, you can just console.log that i,
00:05:05 but then here, since we don't have access to this final expression, you have to increment the i right here within this block of code.
00:05:13 And once again, you get the numbers from 0 to 9. We initialize i to 0 before the loop starts.
00:05:20 The condition will continue as long as i is less than 10. And then we manually increment i within the loop.
00:05:27 Now, a while can also become an infinite loop if you simply say true right here.
00:05:33 But again, don't save this because triggering infinite loops is never what you want and it can block out your browser.
00:05:39 Since I don't want to do that, I'll simply comment this out for now.
00:05:43 And that brings us to the end of the, at least so far, the most exciting module, logic and control flow, where you learned how you can make your code execute
00:05:53 different things based on different conditions, using logical operators and truth and false values.
00:05:59 Next, let's explore one of the most important things that makes JavaScript the language that it is today, functions.