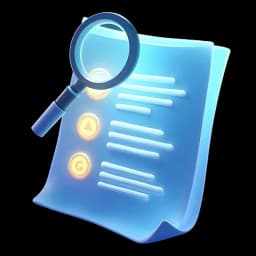
Quick Lecture Overview
Subscribing gives you access to a brief, insightful summary of each lecture to stay on track.
In this lesson, you'll learn about the statement in JavaScript, a fundamental concept for controlling the flow of logic in your programs.
You might have read the title of the current section, "Logic and Control Flow" and wondered what it means. It's much simpler than it may seem.
In every programming language, we have something known as an statement .
An if statement consists of a condition that is evaluated to either or and a block of code. If the condition is , then the code inside the block will run; otherwise, it's going to be skipped. It's that simple. Let's explore it in an example:
Imagine a nightclub that only allows people over the age of 18 to enter.
const age = 18;
if (age >= 18) {
console.log('You may enter, welcome!');
}
statements can also have and statements. To continue with our example:
const age = 18;
if (age >= 18) {
console.log('You may enter, welcome!');
} else if (age === 18) {
console.log('You just turned 18, welcome!');
}
Notice how we have another condition there. If we run this code, what do you expect to see in your console?
Some of you might expect the code that's under the statement, but currently, that would not be the case. Let's test it out.
Why did the first block get logged out? For a really simple reason. It was the first one to pass the check. Our first condition specified that the age must be more than or equal to 18. 18 is indeed equal to 18. So how could we fix this? We can simply change the condition to just a greater than sign.
But what if the person is younger than 18? We currently aren't handling that case. That's where the statement comes in handy. We implement it like this:
const age = 18;
if (age > 18) {
console.log('You may enter, welcome!');
} else if (age === 18) {
console.log('You just turned 18, welcome!');
} else {
console.log('Go away!');
}
Try noticing the difference between the and the compared to the statement. Can you see it?
That's it! You've just learned one of the key concepts of all programming languages! Congrats! 😊
By logging in, you'll unlock full access to this and other free tutorials on JSM Pro.
Why? Logging in lets us personalize your learning experience, track your progress, and keep you in the loop with new workshops, coding tips, and platform updates.
You'll also be the first to know about upcoming launches, events, and exclusive discounts.
No spam—just helpful content to level up your skills.
If that sounds fair, go ahead and log in to continue →
Enter your name and email to get instant access
##Looks like we found a thief monkey By the way, I liked the trick how you reached till here. You have a good sense of humor. You will improve a lot if you join our course with this passion.
var
(function-scoped, outdated)let
(block-scoped, modern and recommended)const
(block-scoped, cannot be reassigned)_
, or $
let let = 5;
is invalid)myVar
and myvar
are different)string
, number
, boolean
, null
, undefined
, bigint
, symbol
Objects
, Arrays
, Functions
Subscribing gives you access to a brief, insightful summary of each lecture to stay on track.
00:00:00 You might've read the title of the current module, Logic and Control Flow, and wondered what it means.
00:00:07 It's much simpler than it may seem.
00:00:08 In every programming language, we have something known as an if statement.
00:00:14 An if statement consists of a condition that is evaluated to either true or false and then a block of code.
00:00:22 If the condition is true, then the code inside the block will run.
00:00:26 Otherwise, it'll be skipped.
00:00:28 It's that simple.
00:00:30 Let me show you how it looks like on an example.
00:00:32 Imagine there's a nightclub.
00:00:34 that only allows people that are over the age of 18 to enter.
00:00:38 We would need to have some kind of a variable to define our age.
00:00:41 But then, how would that guard look like?
00:00:44 Well, it's just a simple if statement.
00:00:46 We can say if, and then use a condition, age is greater than or equal to, remember, we talked about comparison operators before,
00:00:55 18, then we can open up a new block of code.
00:01:01 That looks something like this.
00:01:03 Just a pair of curly braces.
00:01:05 And here we can console log something like, you may enter.
00:01:11 Welcome.
00:01:11 And there we go.
00:01:12 We immediately get this console log within our terminal.
00:01:15 Now, if statements can also have else if and else statements.
00:01:20 To continue with our example, let's add an else if.
00:01:24 And for example, here we can check if age is exactly 18. And in that case, we can say, consulog, you are lucky.
00:01:34 You just turned 18. Something like that, right?
00:01:39 Now, before you go ahead and run this code, what do you think the output will be?
00:01:43 The first one, you may enter, or the second one, you're lucky, you just turned 18. Keeping in mind that our age is exactly 18. Well,
00:01:52 it'll be okay to expect that the second block of code will be executed.
00:01:57 The one where age is exactly equal to 18. But that is not the case.
00:02:02 We once again get, you may enter, welcome.
00:02:05 So, why did this block of code get locked out?
00:02:09 Well, for a simple reason, really.
00:02:11 It was the first one to pass the check.
00:02:14 Here, we're checking whether it's greater than or equal to.
00:02:17 18 is indeed equal to 18. So, how can we fix this?
00:02:22 Well, we can simply change the condition to just a greater than sign.
00:02:27 In that case, this one will no longer be true, and it'll go to an Elsev.
00:02:32 You're lucky, you just turned 18. But what if the person is younger than 18? We aren't currently handling that case, and that's exactly where the else
00:02:42 statement comes in handy.
00:02:43 We can say else, cons a log, and we will be a bit rude here and say go away.
00:02:50 Of course, we get the second message right now, but if we lower the age to something like 15, you can see the go away message.
00:02:58 Now, try noticing the difference between the if and the else if statements compared to the else statement.
00:03:06 Can you see it?
00:03:07 The else statement doesn't have a condition.
00:03:09 That's because if nothing is matched, then else is executed.
00:03:14 Else doesn't need a condition.
00:03:16 If none of the other conditions match the true, else runs.
00:03:20 So, that's it!
00:03:21 You've just learned one of the key concepts of every single programming language.
00:03:25 Congrats!