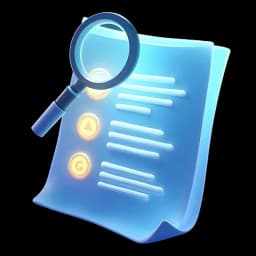
Quick Lecture Overview
Subscribing gives you access to a brief, insightful summary of each lecture to stay on track.
In this lesson, you'll learn about the switch statement in JavaScript, which is useful for performing different operations based on various conditions.
The switch statement is extremely similar to the statement. They can be used interchangeably, but there are situations where a switch is preferred.
With statements, you mostly have just a few conditions: one for the , a few for the , and the final statement. If you have a larger number of conditions, you might want to consider using the statement. Let's explore how it works.
The statement is used to perform different operations based on different conditions.
Let's say that you have a variable called .
var superHero = 'Captain America';
Based on the name of the superhero, you want to display his voice line.
We can do that using the . The takes in a value and then checks it against a bunch of :
switch (superHero) {
case 'Iron Man':
console.log('I am Iron Man...');
break;
case 'Thor':
console.log('That is my hammer!');
break;
case 'Captain America':
console.log('Never give up.');
break;
case 'Black Widow':
console.log('One shot, one kill.');
break;
}
In this example, we passed the variable to the statement.
It executes the first check with a triple equal sign. It looks something like this:
'Captain America' === 'Iron Man'
Since this evaluates to , it skips it and goes to the next one. As soon as it finds the one that matches correctly, it prints the output.
What is that keyword?
The keyword ends the when we get the correct .
If we omit the statement, the next will be executed even if the condition does not match the .
And finally, what if none of the names in the match the name of our ? There must be something like an statement, right? There is! That something is called .
If none of the match, the case is going to be executed. We can implement it like this:
switch (superHero) {
case 'Iron Man':
console.log('I am Iron Man...');
break;
case 'Thor':
console.log('That is my hammer!');
break;
case 'Captain America':
console.log('Never give up.');
break;
case 'Black Widow':
console.log('One shot, one kill.');
break;
default:
console.log('Enter a valid superhero name');
break;
}
That's it! Now you know how to use the . As always, I would advise going into the Chrome console or a code sandbox and playing with it yourself. You learn the most by trying things yourself.
By logging in, you'll unlock full access to this and other free tutorials on JSM Pro.
Why? Logging in lets us personalize your learning experience, track your progress, and keep you in the loop with new workshops, coding tips, and platform updates.
You'll also be the first to know about upcoming launches, events, and exclusive discounts.
No spam—just helpful content to level up your skills.
If that sounds fair, go ahead and log in to continue →
Enter your name and email to get instant access
##Looks like we found a thief monkey By the way, I liked the trick how you reached till here. You have a good sense of humor. You will improve a lot if you join our course with this passion.
var
(function-scoped, outdated)let
(block-scoped, modern and recommended)const
(block-scoped, cannot be reassigned)_
, or $
let let = 5;
is invalid)myVar
and myvar
are different)string
, number
, boolean
, null
, undefined
, bigint
, symbol
Objects
, Arrays
, Functions
Subscribing gives you access to a brief, insightful summary of each lecture to stay on track.
00:00:00 Let's talk about switch statements.
00:00:02 They're super similar to the if statement.
00:00:05 As a matter of fact, you can use ifs and switches interchangeably, but there are situations where a switch is preferred.
00:00:13 With if statements, you mostly have just a few conditions.
00:00:18 One for the if, a few else ifs, and finally a final else statement.
00:00:23 But if you have a larger number of conditions, you might want to consider using the switch statement.
00:00:28 So let me show you how it works.
00:00:30 Let's say you have a variable called superhero.
00:00:34 And let's say you choose something like Captain America.
00:00:38 Based on the hero's name, you might want to display its voice line.
00:00:42 And we can do that using a switch statement.
00:00:44 The switch statement takes in a key.
00:00:47 This key is the variable that it'll try to compare with the value.
00:00:51 And you might see that this switch statement got auto-populated for me, because I started typing it, and then I selected this switch statement,
00:00:59 which auto-filled it for me.
00:01:00 You can do that or we can write it together from scratch.
00:01:04 So the first thing we need is something to compare against the other values.
00:01:09 In this case, we're going to take in our superhero value, and then we can have different cases that we're going to check that value against.
00:01:18 So we can say, in case the hero is Iron Man, we can then render a console log of I am Ironman, and we need to break that case to stop the execution.
00:01:36 Next, we can have another case.
00:01:38 For example, here will be Thor.
00:01:42 And then we can have a Consolog, something like that is my hammer.
00:01:46 We can break it and have another case where it'll be something like Captain America.
00:01:53 And we'll Consolog something like never give up.
00:01:59 Sounds like something he would say.
00:02:01 And we can break it.
00:02:02 And that's good enough for me.
00:02:04 So now if you save it, you'll see that we obviously get never give up because in this case, the superhero is Captain America.
00:02:13 Of course, if you change it to something like Iron Man, you're going to get a different quote.
00:02:19 This is very similar to using if and else statements, but it looks a bit cleaner.
00:02:24 Sometimes you can even put it in a single line like this.
00:02:31 So it doesn't take a lot of space, but you can still understand exactly what is happening, right?
00:02:37 Much better than chaining many if statements.
00:02:40 The way in which this work is that we pass the superhero variable to the switch statement.
00:02:45 What JavaScript then does is it takes that value and compares it with a triple equal sign with the first case.
00:02:52 So if superhero is triple equal to Iron Man, Only if that is true, it'll render this one.
00:03:01 If it's not, then it'll continue down below.
00:03:04 And what is that break keyword?
00:03:05 Well, the break ends the switch statement when we get the correct case.
00:03:09 If you forget to add a break, it'll continue going through all of the other cases, and you'll get three different console logs.
00:03:16 And finally, what happens if none of the cases match the name of our superhero?
00:03:22 There must be something like an else statement, right?
00:03:25 Well, there is.
00:03:26 It's called default.
00:03:28 You can say default and just render a console log of something like enter a valid superhero name.
00:03:39 And for the syntax to be valid, we have to add a colon right here.
00:03:43 If we do that and change the name to something invalid and save it, you can see that we have enter a valid superhero name.
00:03:52 That's it.
00:03:53 Now you know how to use a switch statement.
00:03:55 As always, to fully understand how it works, I would recommend just switching some things up a bit, thinking of a new example on your own,
00:04:02 and then testing it across different cases.
00:04:05 That's the only way to truly understand how everything works under the hood.