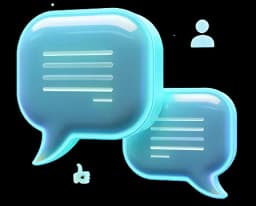
Join the Conversation!
Subscribing gives you access to the comments so you can share your ideas, ask questions, and connect with others.
In this lesson, you'll learn about the operator in JavaScript, which inverts the truthiness of values, helping you manage conditions effectively.
An exclamation sign is known as the NOT operator. It reverses the boolean result of the condition.
The syntax is pretty simple:
console.log(!true); // false
The operator accepts a single argument and does the following:
For instance:
console.log(!true); // false
console.log(!0); // true
A double NOT is sometimes used for converting a value to boolean type:
console.log(!!"truthy"); // true
console.log(!!null); // false
"Please login to view comments"
Subscribing gives you access to the comments so you can share your ideas, ask questions, and connect with others.
How did you manage to remove the blur property and reach here?
Upgrading gives you access to quizzes so you can test your knowledge, track progress, and improve your skills.
By logging in, you'll unlock full access to this and other free tutorials on JSM Pro.
Why? Logging in lets us personalize your learning experience, track your progress, and keep you in the loop with new workshops, coding tips, and platform updates.
You'll also be the first to know about upcoming launches, events, and exclusive discounts.
No spam—just helpful content to level up your skills.
If that sounds fair, go ahead and log in to continue →
Enter your name and email to get instant access
##Looks like we found a thief monkey By the way, I liked the trick how you reached till here. You have a good sense of humor. You will improve a lot if you join our course with this passion.
var
(function-scoped, outdated)let
(block-scoped, modern and recommended)const
(block-scoped, cannot be reassigned)_
, or $
let let = 5;
is invalid)myVar
and myvar
are different)string
, number
, boolean
, null
, undefined
, bigint
, symbol
Objects
, Arrays
, Functions
Subscribing gives you access to a brief, insightful summary of each lecture to stay on track.
00:00:00 Before, I showed you a simple way to figure out if a value is truthy or falsy.
00:00:04 And that is to just pass it into the condition of an if statement.
00:00:08 If it comes out on the other end as a part of an if block, then it's truthy.
00:00:12 If it goes to an end block, it is falsy.
00:00:14 But there are actually two even simpler ways to figure out the truthiness of a specific value.
00:00:20 The second one is to use the not operator.
00:00:23 Yep, this one right here.
00:00:25 The way in which it works is you can use a double exclamation sign or a double knot to convert a value to a boolean type.
00:00:34 Let me show you what I mean.
00:00:35 If you have a string that has some value, it is obviously truthy, right?
00:00:40 We can say something like test, just not to confuse yourselves.
00:00:44 And then as we learned, if you negate a truthy value, we get back false.
00:00:50 But now if you add an additional exclamation mark at the start of it, you will do a double negation.
00:00:56 So if you negate false once again, you will get true.
00:01:00 What does this mean?
00:01:01 Well, in simple words, this means that the value is truthy because we converted it one to a false and then once again, to a true.
00:01:10 In a similar way, if you do a double negation of a falsy value, like zero, you will get back false, letting you know that it is indeed falsy.
00:01:19 The only reason why I wanted to show this to you is because you might see it sometime in the wild.
00:01:24 And basically what this is doing is it is converting a value to its true Boolean type.
00:01:32 So converting truth into true and false to a real Boolean false.
00:01:37 Good.
00:01:37 Now you know that too, but I think there's an even better way.
00:01:40 And that is to use a JavaScript Boolean class.
00:01:45 That'll look something like this.
00:01:47 Conzalog, and you can use the Boolean class and wrap whatever you want with it.
00:01:54 If you wrap a value of test, you will get true, because this one will convert it into a boolean true.
00:02:02 And in a similar way, if you pass something like null, zero, or undefined, we will get false.
00:02:10 So it works in exactly the same way, but it is a bit clearer of what's happening behind the scenes.
00:02:16 Now, just to finalize this conversation about the logical operators and truth and false values, think of JavaScript as super lazy.
00:02:23 It wants to do the least amount of work possible to get to its return value.
00:02:28 So with the end operator, JavaScript will first try to return the first false value.
00:02:35 because that's the only thing it has to do.
00:02:37 No matter how truthy values it has, for the AND comparison to work, all of them must be truthy.
00:02:43 So, as soon as it finds the false ones, it'll exit and return it.
00:02:47 In this case, an empty string.
00:02:49 And it's the same thing with the OR operator.
00:02:52 It'll work in the other way around.
00:02:54 JavaScript will first try to return the first truthy value that it finds.
00:02:58 So if it immediately finds a string of test, no matter how many false values there are, it doesn't matter.
00:03:05 It'll immediately return a string of test.
00:03:08 Great.
00:03:09 Hopefully now you have a better understanding of the logical operators and truth and false values.
00:03:14 I don't expect you to immediately know how you'll be able to use these within your code, but I want you to know how they work.
00:03:21 So as I teach you more things, such as the switch statements, ternary operators, for loops and functions, at the back of your mind,
00:03:30 you always know what truth and false are so that you can use it alongside your other JavaScript code.