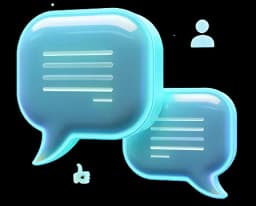
Join the Conversation!
Subscribing gives you access to the comments so you can share your ideas, ask questions, and connect with others.
In this lesson, you'll learn about truthy and falsy values in JavaScript, which are crucial for understanding how conditions are evaluated in your code.
In the previous section, we learned about strict and loose equality in JavaScript. Equality always results in a boolean value, which can either be or .
Unlike some other languages, and in JavaScript are not limited to boolean data types and comparisons . They can take many other forms.
In JavaScript, we have something known as truthy values and falsy values.
Understanding truthy and falsy values in JavaScript is essential . If you don't know which values evaluate to truthy and which to falsy, you might struggle to read and understand other people's code.
Longtime JavaScript developers often use the terms "truthy" and "falsy" but for those new to JavaScript, these terms can be a bit confusing.
When we say a value is "truthy" in JavaScript, we don't just mean that the value is . Rather, it means the value coerces to when evaluated in a boolean context. Let's explore what that means.
The easiest way to learn truthy and falsy values is to memorize the falsy values. There are only six falsy values; all other values are truthy.
FALSY VALUES:
Note: An empty array is not falsy.
Everything that is not falsy
That's a pretty straightforward list. But how can we actually use truthiness? Let's look at an example.
Taking advantage of truthiness can make your code more concise. You don't need to explicitly check for , , etc. Instead, you can just check whether a value is truthy. However, there are some caveats to keep in mind.
Example:
let value = "Hello";
if (value) {
console.log("This is a truthy value!");
} else {
console.log("This is a falsy value!");
}
"Please login to view comments"
Subscribing gives you access to the comments so you can share your ideas, ask questions, and connect with others.
By logging in, you'll unlock full access to this and other free tutorials on JSM Pro.
Why? Logging in lets us personalize your learning experience, track your progress, and keep you in the loop with new workshops, coding tips, and platform updates.
You'll also be the first to know about upcoming launches, events, and exclusive discounts.
No spam—just helpful content to level up your skills.
If that sounds fair, go ahead and log in to continue →
Enter your name and email to get instant access
##Looks like we found a thief monkey By the way, I liked the trick how you reached till here. You have a good sense of humor. You will improve a lot if you join our course with this passion.
var
(function-scoped, outdated)let
(block-scoped, modern and recommended)const
(block-scoped, cannot be reassigned)_
, or $
let let = 5;
is invalid)myVar
and myvar
are different)string
, number
, boolean
, null
, undefined
, bigint
, symbol
Objects
, Arrays
, Functions
Subscribing gives you access to a brief, insightful summary of each lecture to stay on track.
00:00:00 In the previous module, we talked about strict and loose equality.
00:00:05 We said that equality always results in a Boolean value, which can either be true or false.
00:00:11 But here's where JavaScript gets tricky.
00:00:13 Unlike some other languages, true and false in JavaScript are not limited to Boolean data types and comparisons.
00:00:21 They can take many other forms.
00:00:23 In JavaScript, we have something known as truthy and falsy values.
00:00:29 Truthy expressions are the ones that always evaluate to a boolean true, and falsy expressions are ones that always evaluate to a boolean false.
00:00:40 Understanding truthy and falsy values in JavaScript is essential.
00:00:45 If you don't know which values evaluate to truthy and which to falsy, you might struggle to read and understand other people's code.
00:00:53 When I say a value is truthy in JavaScript, I don't just mean that value is a boolean true.
00:00:59 Rather, it means that it coerces to true when evaluated in a boolean context.
00:01:04 But of course, that doesn't mean a lot.
00:01:06 It's just words.
00:01:07 So let me explain what it means in a simpler way.
00:01:10 First, we can talk about falsy values.
00:01:13 The easiest way to learn truthy and falsy values is to memorize the falsy ones because there are only six falsy values.
00:01:22 All other values in the entirety of the JavaScript programming language are truthy.
00:01:28 So let's remember the falsy ones.
00:01:31 The first one on the list is a boolean false.
00:01:34 Of course, that's a falsy value, right?
00:01:36 After that, we have a zero.
00:01:39 This is a number zero.
00:01:42 Next, we have any kind of an empty string, be that a single-quoted string, double-quoted string, or empty backticks.
00:01:50 The fourth value that coerces to false C is a null.
00:01:55 The fifth one is, can you guess it?
00:01:57 undefined, and the sixth one is nan, not a number.
00:02:03 You might think that an empty object or an empty array would also fall into a category of falsy values, but that is incorrect.
00:02:12 That is a super common mistake that beginners make, so don't make it.
00:02:16 But how can you trust me?
00:02:18 How can you know that what I'm saying is true?
00:02:21 Am I just making this up?
00:02:23 Well, I will show you how to test the truthiness or falsiness of specific values.
00:02:29 Now that you know which ones are falsy, they're only six, you also need to know which ones are truthy, right?
00:02:36 Just before we can test it.
00:02:37 And truthy values are, in simple words, all values That are not falsy.
00:02:45 Pretty simple, right?
00:02:46 But what does this even mean for us?
00:02:49 Well, you can use the truthy and falsy values to make your code more concise because then you don't need to explicitly check for undefined or empty strings
00:02:59 or something else.
00:03:00 You can just check whether a value is truthy.
00:03:02 For example, let's say you have any kind of a value.
00:03:05 I'll call it value.
00:03:07 In this case, we can make it a string of hello.
00:03:10 And now you want to check the truthiness of that value.
00:03:14 The simplest way to check it would be to create an if statement and check whether a value exists.
00:03:22 Just be passing it like this.
00:03:23 Now, why would this if tell us anything?
00:03:26 Well, think about it.
00:03:27 By default, if will only render this block of code if the value is true.
00:03:34 So we can say true right here.
00:03:36 And it will render this block of code if a value is false.
00:03:41 We learned that in the last lesson, right?
00:03:43 But in this case, we get obviously true because this is a Boolean true.
00:03:47 But now what would happen if we only put the value right here and don't make any kind of a comparison on it?
00:03:54 We just pass the value itself.
00:03:56 we still get true, which means that we entered this block of code.
00:04:01 This wouldn't really be true because it's not a boolean true, but it is truthy.
00:04:07 And this one is falsy.
00:04:09 I can even make this into a template string and say value of value is truthy.
00:04:17 And I can copy this and duplicate it right below.
00:04:21 And I can say value is falsy if it reaches this second block of code.
00:04:26 So in this case, we obviously get hello is truthy.
00:04:30 We don't even need to say value.
00:04:32 There we go.
00:04:33 Now, what if we pass an empty string?
00:04:35 We get nothing or an empty string is falsy.
00:04:38 What if we pass something like a string of test?
00:04:42 Test is truthy.
00:04:43 Something is there.
00:04:45 What if we pass any kind of a number?
00:04:47 That number is truthy.
00:04:49 A boolean true is truthy.
00:04:52 Anything you can think of, like an empty array or an empty object, are also truthy.
00:04:57 But now, if we pass any of the values on the list of the falsy values, such as a 0, you can see that 0 is falsy.
00:05:05 Null, what about null?
00:05:07 Null is falsy.
00:05:09 Undefined is falsy as well.
00:05:12 So hopefully this is starting to make sense.
00:05:14 Even though I didn't yet fully explain how this will help you in writing your JavaScript programs, I just want you to understand that there are truthy
00:05:22 and falsy values.
00:05:23 Truthy values are all the values that are not falsy and falsy values are just these six.
00:05:30 And let's be honest, it kind of makes sense.
00:05:32 False is falsy, zero, empty strings, nulls, undefineds, and nones.
00:05:38 In the upcoming lessons, it'll start making so much more sense of why is it crucial that when I give you a value, you immediately know what that value is,
00:05:47 either truthy or falsy, because it'll significantly impact the way you can read and understand the code that you're writing.