Lifetime access to this course
ES6+ What you already know
In this lesson, you'll explore some of the key features introduced in ES6, also known as ECMAScript 6. These features have significantly enhanced JavaScript, making it more powerful and easier to work with. Let's dive into what ES6 brought to the table and how you're already using these features in your code. ### What is ES6? ECMAScript 6, or simply ES6, is the 6th edition of the ECMAScript standard, which specifies the core features of JavaScript. Released in 2015, ES6 introduced a host of new features that have become essential for modern JavaScript development. Think of ES6 as a major update to JavaScript, similar to a significant update to your favorite software or game. ## Key Features of ES6
The rest of this lesson is waiting.
Join JS Mastery Pro to unlock it.
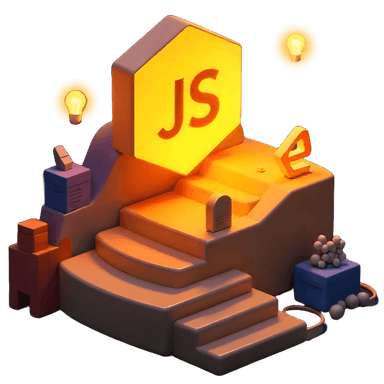
Complete Path to JavaScript Mastery
$300
Buy the Course OnlyBuild advanced web app
Certificate of completion included
Great for focused learners who just want this course
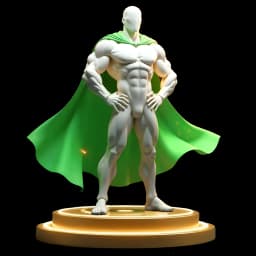
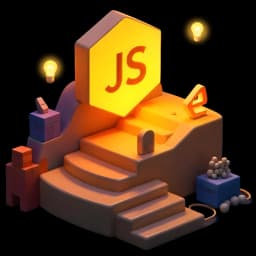
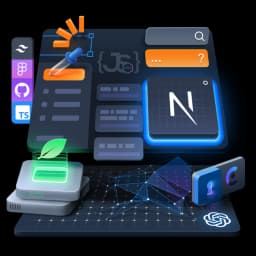
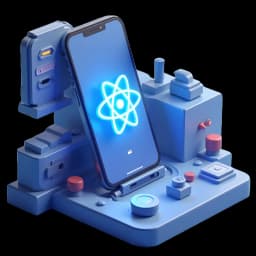
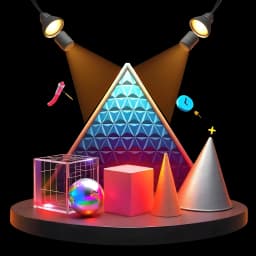
Get Full Access
$120 /month
Lock in a year & save—2 months free!
Access to all courses
Quizzes, mini-challenges, AI summaries, inline sandboxes
Participation in a private community
Build real production-grade apps
Mock interviews & project reviews
Weekly live sessions & member-only access