Lifetime access to this course
Imports & Exports
In this lesson, you'll learn about imports and exports in JavaScript, which are essential for organizing and managing code, especially in larger applications like those built with React. These features allow you to split your code into modules, making it more maintainable and reusable. ### Understanding Imports and Exports JavaScript modules allow you to break up your code into separate files, each responsible for a specific piece of functionality. This modular approach is particularly useful in frameworks like React, where components and utilities are often separated into different files. ## Exporting from a Module In JavaScript, you can export variables, functions, or objects from a module so that they can be used in other files. There are two main types of exports: named exports and default exports.
The rest of this lesson is waiting.
Join JS Mastery Pro to unlock it.
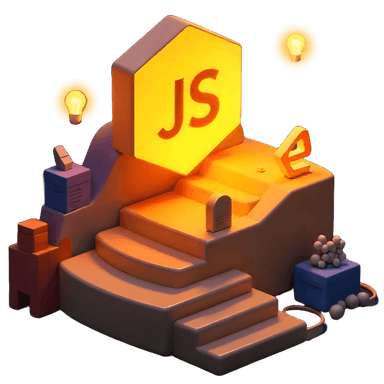
Complete Path to JavaScript Mastery
$300
Buy the Course OnlyBuild advanced web app
Certificate of completion included
Great for focused learners who just want this course
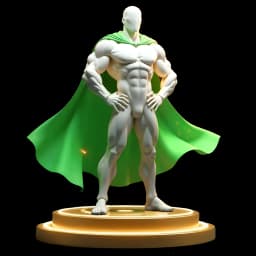
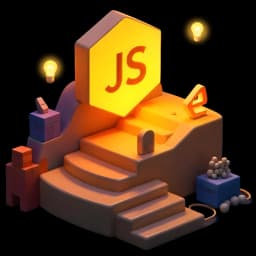
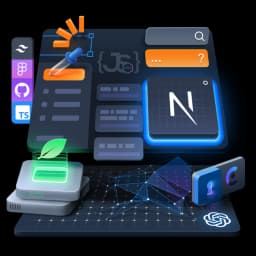
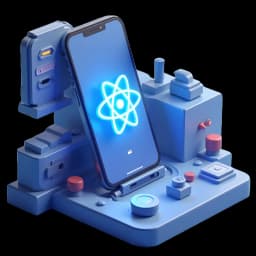
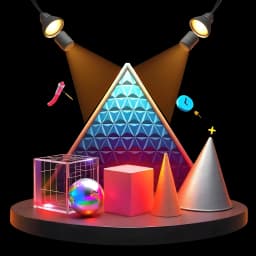
Get Full Access
$120 /month
Lock in a year & save—2 months free!
Access to all courses
Quizzes, mini-challenges, AI summaries, inline sandboxes
Participation in a private community
Build real production-grade apps
Mock interviews & project reviews
Weekly live sessions & member-only access