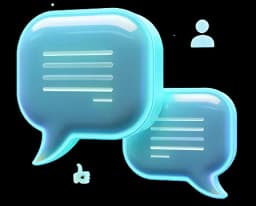
Join the Conversation!
Subscribing gives you access to the comments so you can share your ideas, ask questions, and connect with others.
In this lesson, you'll learn about rest parameters in JavaScript, a feature introduced in ES6 that allows functions to accept an indefinite number of arguments as an array. This feature simplifies the handling of variadic functions, making your code cleaner and more flexible.
Rest parameters enable you to represent an indefinite number of arguments as an array . This is particularly useful when you want to work with functions that can take a variable number of arguments .
The rest parameter syntax uses three dots followed by a name, which collects all remaining arguments into an array.
Example:
function calculateTotal(...numbers) {
let sum = 0;
for (const num of numbers) {
sum += num;
}
return sum;
}
console.log(calculateTotal(1, 2, 3)); // Expected output: 6
console.log(calculateTotal(1, 2, 3, 4)); // Expected output: 10
Key Points:
function displayInfo(first, second, ...additionalInfo) {
console.log("First:", first);
console.log("Second:", second);
console.log("Additional Info:", additionalInfo);
}
displayInfo("apple", "banana", "cherry", "date", "elderberry");
// First: apple
// Second: banana
// Additional Info: ["cherry", "date", "elderberry"]
function scaleValues(factor, ...values) {
return values.map((value) => factor * value);
}
const scaledArray = scaleValues(3, 10, 20, 30);
console.log(scaledArray); // [30, 60, 90]
Before rest parameters, you had to convert to a normal array:
function oldStyleFunction(a, b) {
const arrayVersion = Array.prototype.slice.call(arguments);
// — or —
const arrayVersion2 = [].slice.call(arguments);
// — or —
const arrayVersionFrom = Array.from(arguments);
const firstElement = arrayVersion.shift(); // OK, gives the first argument
}
With rest parameters, you can directly access a normal array:
function newStyleFunction(...args) {
const arrayVersion = args;
const firstElement = arrayVersion.shift(); // OK, gives the first argument
}
Rest parameters simplify the handling of functions with variable numbers of arguments, reducing boilerplate code and enhancing readability. By using rest parameters, you can write more flexible and maintainable JavaScript functions. This feature is especially useful in modern JavaScript development, where functions often need to handle dynamic input. Use a code sandbox or console to practice these examples and deepen your understanding of rest parameters.
"Please login to view comments"
Subscribing gives you access to the comments so you can share your ideas, ask questions, and connect with others.