Lifetime access to this course
Spread Syntax
In this lesson, you'll learn about the spread syntax in JavaScript, a powerful feature introduced in ES6 that allows you to expand elements of an iterable (like an array or string) in places where multiple arguments or elements are expected. This feature is particularly useful for working with arrays and objects, making your code more concise and readable. ### Understanding Spread Syntax The spread syntax ... allows you to expand an iterable into individual elements. It is the opposite of rest syntax, which collects multiple elements into a single array. Spread syntax can be used in function calls, array literals, and object literals.
The rest of this lesson is waiting.
Join JS Mastery Pro to unlock it.
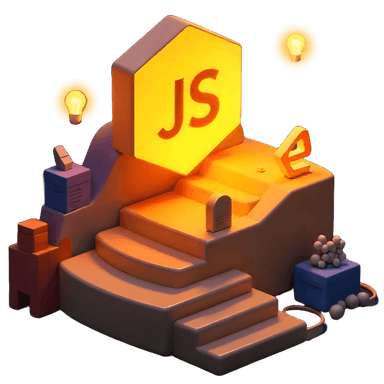
Complete Path to JavaScript Mastery
$300
Buy the Course OnlyBuild advanced web app
Certificate of completion included
Great for focused learners who just want this course
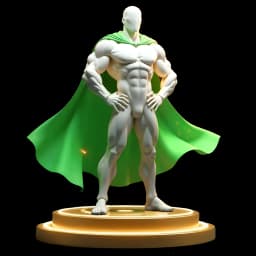
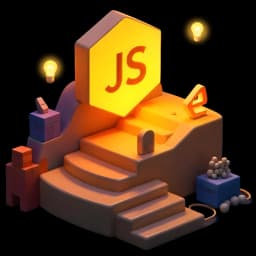
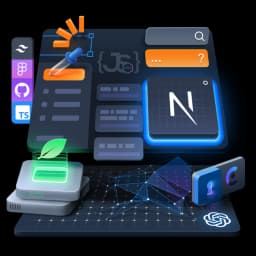
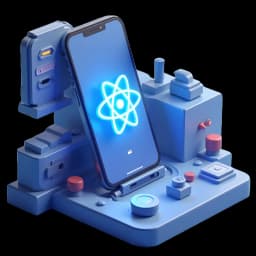
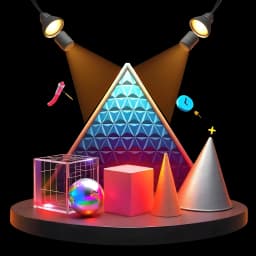
Get Full Access
$120 /month
Lock in a year & save—2 months free!
Access to all courses
Quizzes, mini-challenges, AI summaries, inline sandboxes
Participation in a private community
Build real production-grade apps
Mock interviews & project reviews
Weekly live sessions & member-only access