Lifetime access to this course
Accessing, Adding, and Updating and Object's Properties
In this lesson, you'll learn about accessing, adding, and updating properties of objects in JavaScript using dot notation and square bracket notation. ### Accessing, Adding, and Updating an Object's Properties Objects in JavaScript are collections of key-value pairs, and you can access, add, or update these properties using two main notations: dot notation and square bracket notation. ## Dot Notation .
The rest of this lesson is waiting.
Join JS Mastery Pro to unlock it.
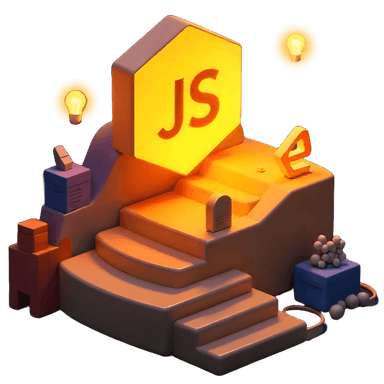
Complete Path to JavaScript Mastery
$300
Buy the Course OnlyBuild advanced web app
Certificate of completion included
Great for focused learners who just want this course
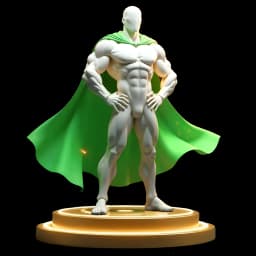
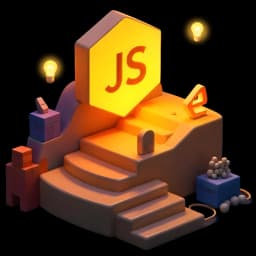
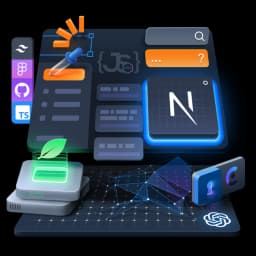
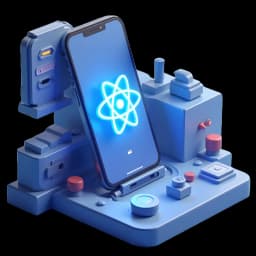
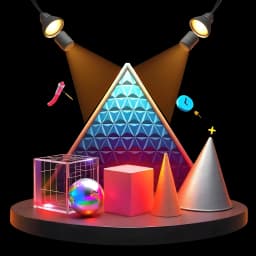
Get Full Access
$149 /month
Lock in a year & save—2 months free!
Access to all courses
Quizzes, mini-challenges, AI summaries, inline sandboxes
Participation in a private community
Build real production-grade apps
Mock interviews & project reviews
Weekly live sessions & member-only access