Lifetime access to this course
Object Methods
In this lesson, you'll learn about methods in JavaScript, which are functions associated with objects. Methods are a fundamental concept in object-oriented programming and are used to define behaviors for objects. ### What is a Method? A method is a function associated with an object. Simply put, a method is a property of an object that is a function. Methods allow objects to perform actions and are defined similarly to regular functions, but they are assigned as properties of an object. ### Defining Methods
The rest of this lesson is waiting.
Join JS Mastery Pro to unlock it.
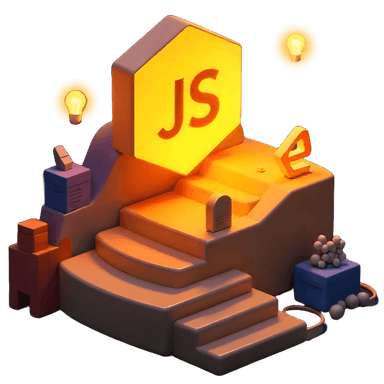
Complete Path to JavaScript Mastery
$300
Buy the Course OnlyBuild advanced web app
Certificate of completion included
Great for focused learners who just want this course
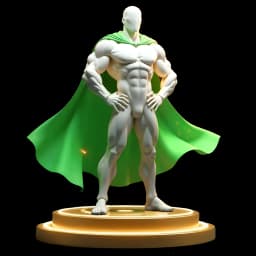
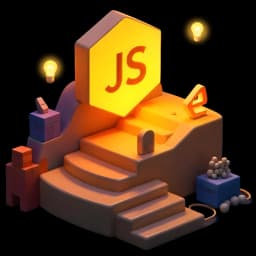
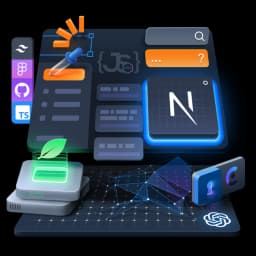
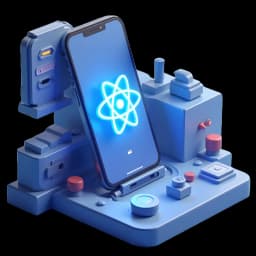
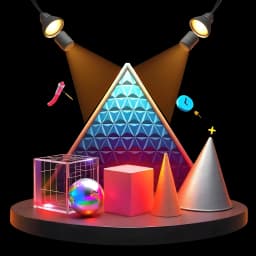
Get Full Access
$149 /month
Lock in a year & save—2 months free!
Access to all courses
Quizzes, mini-challenges, AI summaries, inline sandboxes
Participation in a private community
Build real production-grade apps
Mock interviews & project reviews
Weekly live sessions & member-only access