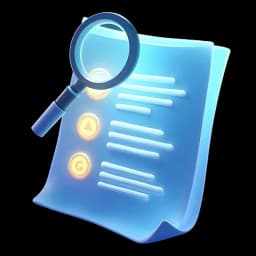
Quick Lecture Overview
Subscribing gives you access to a brief, insightful summary of each lecture to stay on track.
In this lesson, you'll learn how to use in JavaScript to perform basic mathematical operations, including addition, subtraction, multiplication, division, modulus, increment, decrement, and exponentiation.
Arithmetic operators in JavaScript are essential for performing mathematical operations on numeric operands. These include basic operations like addition , multiplication , and subtraction , which are familiar from school. These operators are fundamental in programming for handling calculations and data manipulation.
Here's how you can use arithmetic operators in JavaScript:
// Arithmetic Operators Demo in JavaScript
// Addition (+)
const sum = 10 + 5;
console.log("Addition: 10 + 5 =", sum); // Output: 15
// Subtraction (-)
const difference = 20 - 7;
console.log("Subtraction: 20 - 7 =", difference); // Output: 13
// Multiplication (*)
const product = 6 * 4;
console.log("Multiplication: 6 * 4 =", product); // Output: 24
// Division (/)
const quotient = 15 / 3;
console.log("Division: 15 / 3 =", quotient); // Output: 5
// Modulus (%) - Remainder after division
const remainder = 17 % 5;
console.log("Modulus: 17 % 5 =", remainder); // Output: 2
// Exponentiation (**)
const power = 3 ** 3;
console.log("Exponentiation: 3 ** 3 =", power); // Output: 27
// Increment (++)
let incrementValue = 5;
incrementValue++; // Equivalent to incrementValue = incrementValue + 1
console.log("Increment: 5++ =", incrementValue); // Output: 6
// Decrement (--)
let decrementValue = 8;
decrementValue--; // Equivalent to decrementValue = decrementValue - 1
console.log("Decrement: 8-- =", decrementValue); // Output: 7
These operations are straightforward and form the backbone of many programming tasks. Understanding how to use them effectively is crucial for any JavaScript developer. Now that we've covered , let's move on to ! 😊
By logging in, you'll unlock full access to this and other free tutorials on JSM Pro.
Why? Logging in lets us personalize your learning experience, track your progress, and keep you in the loop with new workshops, coding tips, and platform updates.
You'll also be the first to know about upcoming launches, events, and exclusive discounts.
No spam—just helpful content to level up your skills.
If that sounds fair, go ahead and log in to continue →
Enter your name and email to get instant access
##Looks like we found a thief monkey By the way, I liked the trick how you reached till here. You have a good sense of humor. You will improve a lot if you join our course with this passion.
var
(function-scoped, outdated)let
(block-scoped, modern and recommended)const
(block-scoped, cannot be reassigned)_
, or $
let let = 5;
is invalid)myVar
and myvar
are different)string
, number
, boolean
, null
, undefined
, bigint
, symbol
Objects
, Arrays
, Functions
Subscribing gives you access to a brief, insightful summary of each lecture to stay on track.
00:00:00 Let's talk about performing basic math operations in JavaScript.
00:00:04 Let's explore them one by one, starting with addition.
00:00:08 To add two numbers together, you simply need to use the plus operator in between.
00:00:13 For example, 10 plus 5 is of course 15. After that, similarly, we have the subtraction, where we have the difference between the two numbers,
00:00:23 and you just use the minus sign.
00:00:25 That results in 13. Next, we have the multiplication, where you can get the product of two numbers.
00:00:31 For example, 6 times 4 is 24. Next, we have the division, where if you try to divide 15 by 3, you of course get 5. All of this is super simple.
00:00:43 Then there's the remainder.
00:00:45 This is a bit of a new operator, which is typically not used in basic math, but is used a lot in programming.
00:00:51 The way that the remainder works is you try to fit the number 5 as many times into the number 17 as possible.
00:00:58 In this case, we can fit it three times.
00:01:00 So 5, 10, 15. And then you take the remainder that wasn't able to fit into another 5, in this case being 2. You can also raise the numbers to a specific power,
00:01:10 like 3 to the power of 3, outputs 27. And next, finally, we have increment and decrement, where you can, for example, take a value of 5 and use the plus
00:01:22 plus operator to increment it by 1. So the output is 6, or if you have an 8, minus minus, you get 7. And that's it.
00:01:32 These operations are straightforward and form the backbone of many programming tasks.
00:01:37 Yeah, sure.
00:01:37 You don't have to get back to middle school to understand how these work, and you don't need to know complex math to do programming,
00:01:44 but numbers are used quite a lot in any kind of applications we do, so it's good to know how to work with them.
00:01:50 Next, let's move on to comparison operators.