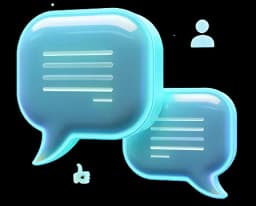
Join the Conversation!
Subscribing gives you access to the comments so you can share your ideas, ask questions, and connect with others.
In this lesson, you'll learn how to use in JavaScript to compare values and understand the concept of equality, including the differences between strict and loose equality.
As you've already learned about arithmetic operators, comparison operators work similarly in that they are fundamental tools in programming. You've most likely heard of operators like greater than , less than , or equal to .
Comparison operators compare two values and return a boolean value: or . This is the main takeaway: the return value of a comparison operator is always a boolean.
The topic of equality in JavaScript is closely connected to comparison operators. We'll cover both topics in this lesson. Let's start with an example using two numbers:
// Comparison Operators Demo in JavaScript
const x = 10;
const y = 5;
const z = "10"; // A string with the same value as x
// Greater than (>)
console.log("Is x > y? ", x > y); // true (10 is greater than 5)
// Greater than or equal to (>=)
console.log("Is x >= y? ", x >= y); // true (10 is greater than 5)
// Less than (<)
console.log("Is y < x? ", y < x); // true (5 is less than 10)
// Less than or equal to (<=)
console.log("Is y <= x? ", y <= x); // true (5 is less than 10)
// Loose equality (==) - Compares values, but ignores types
console.log("Is x == z? ", x == z); // true (10 == "10" because == allows type conversion)
// Strict equality (===) - Compares both value and type
console.log("Is x === z? ", x === z); // false (10 is a number, "10" is a string)
// Loose inequality (!=) - Checks if values are different, ignoring type
console.log("Is x != z? ", x != z); // false (10 == "10" due to type coercion)
// Strict inequality (!==) - Checks if values OR types are different
console.log("Is x !== z? ", x !== z); // true (10 is a number, "10" is a string)
Everything we've covered so far is straightforward. The key point to remember is that comparison operators always return a boolean value.
The only aspect that requires a deeper look is the difference between: strict , and loose , equality.
Understanding when to use each is crucial, and we'll explore that in the next lesson!
"Please login to view comments"
Subscribing gives you access to the comments so you can share your ideas, ask questions, and connect with others.
How did you manage to remove the blur property and reach here?
Upgrading gives you access to quizzes so you can test your knowledge, track progress, and improve your skills.
By logging in, you'll unlock full access to this and other free tutorials on JSM Pro.
Why? Logging in lets us personalize your learning experience, track your progress, and keep you in the loop with new workshops, coding tips, and platform updates.
You'll also be the first to know about upcoming launches, events, and exclusive discounts.
No spam—just helpful content to level up your skills.
If that sounds fair, go ahead and log in to continue →
Enter your name and email to get instant access
##Looks like we found a thief monkey By the way, I liked the trick how you reached till here. You have a good sense of humor. You will improve a lot if you join our course with this passion.
var
(function-scoped, outdated)let
(block-scoped, modern and recommended)const
(block-scoped, cannot be reassigned)_
, or $
let let = 5;
is invalid)myVar
and myvar
are different)string
, number
, boolean
, null
, undefined
, bigint
, symbol
Objects
, Arrays
, Functions
Subscribing gives you access to a brief, insightful summary of each lecture to stay on track.
00:00:00 Comparison operators in JavaScript are used to compare values.
00:00:04 And for you to be able to compare values, you also need to understand the concept of equality.
00:00:10 And in JavaScript, there are two types of equality, strict and loose equality.
00:00:15 As we already talked about arithmetic operators, comparison operators work in a similar way.
00:00:20 You've most likely already heard of operators like greater than, less than, or equal to.
00:00:25 Comparison operators compare two values and return a boolean value, either a true or a false.
00:00:33 This is the main takeaway.
00:00:34 The return value of a comparison operator is always a boolean, and the topic of equality in JavaScript is closely connected to comparison operators.
00:00:45 So, I want to teach you both of these topics in the same lesson.
00:00:48 Let's start with an example of using two numbers.
00:00:52 We have an x which is equal to 10, as well as y which is equal to 5. And we also have a z value which is equal to a string of 10. First,
00:01:02 we can explore the greater than operator.
00:01:04 It is super simple to use.
00:01:06 You simply say x is greater than y.
00:01:10 And for that, you're gonna get true because 10 is greater than 5. You also have access to greater than or equal to, and in this case,
00:01:18 it's also true because x is, well, greater than or equal to 5. Next, we're switching it around and checking if y, which is 5, is less than or less than
00:01:29 or equal to 10 and in both of these cases we get back the answer true because 5 is less than 10. Next we have the topic of loose equality.
00:01:39 Loose equality is written down by using two equal signs.
00:01:44 What it does is it compares the values but it ignores the types.
00:01:49 So here we're checking whether x is loosely equal to z.
00:01:55 And x, in this case, is the number 10, and z, in this case, is a string of 10. And the output of that, believe it or not,
00:02:03 is true.
00:02:04 It's saying that a number of 10 is equal to a string of 10 because we used loose equality.
00:02:10 This is typically not the result you want to get.
00:02:13 Because when you're comparing numbers, you want to be working with numbers, right?
00:02:16 So that's why it's always recommended to use strict equality, denoted by a triple equal sign, which compares both the value and the type.
00:02:27 So if you check whether X is triple equal to Z, X being a number of 10, and Z being a string of 10, you're going to get back false,
00:02:36 because 10 is a number, and 10 right here is a string.
00:02:40 So of course, that ends up being false.
00:02:43 And similarly to how we have equality, we also have inequality, where you can say that x is not loosely equal to z, where you're going to get false because
00:02:53 it thinks that it is, or you can check for a strict inequality with a double equal sign right here.
00:03:00 So a number, x, is not strictly equal to a string of x, you're gonna get true because it is not.
00:03:07 Look, these comparison operators are pretty simple, especially the greater than, greater than or equal to, or lower than or equal to.
00:03:14 Where it does get a bit more complicated is with different equalities.
00:03:19 And I get that.
00:03:20 That does require a deeper look to fully understand the difference between strict and loose equality.
00:03:26 Understanding when to use which is crucial.
00:03:29 So let's dive deeper into that within the next lesson.