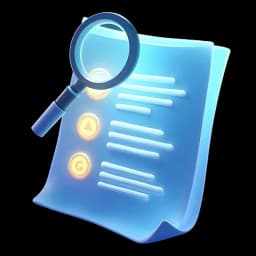
Quick Lecture Overview
Subscribing gives you access to a brief, insightful summary of each lecture to stay on track.
In this lesson, you'll learn about the basic syntax and functionality of logical operators in JavaScript, which are used to combine multiple conditions.
Logical operators are used to combine two or more conditions. JavaScript includes three logical operators: (OR), (AND), and (NOT).
Complete knowledge of logical operators requires an understanding of if/else statements and truthy and falsy values. In this lesson, we'll cover the syntax of logical operators, and we'll revisit them in more detail after covering those topics.
The double ampersand is known as the AND operator. It checks whether all operands are truthy values. If they are, it returns ; otherwise, it returns .
// **AND Operator (&&)**
console.log(true && false); // false
console.log(true && true); // true
console.log(false && false); // false
You can also pass multiple conditions:
console.log(true && true && false); // false
The double vertical bar is known as the OR operator. It checks whether at least one operand is a truthy value. If there is at least one truthy value, it returns ; otherwise, it returns .
// OR Operator (||)
// The double vertical bar **||** is known as the OR operator. It checks whether **at least one operand** is a truthy value. If there is at least one truthy value, it returns **true**; otherwise, it returns **false**.
console.log(true || false); // true
console.log(true || true); // true
console.log(false || false); // false
You can also pass multiple conditions:
console.log(true || true || false); // true
The exclamation mark is known as the NOT operator. It reverses the boolean result of a condition.
The syntax is straightforward:
**// NOT Operator (!)**
// The exclamation mark **!** is known as the NOT operator. It reverses the boolean result of a condition.
console.log(!true); // false
console.log(!false); // true
As you can see, the NOT operator simply converts boolean to , and boolean to .
By logging in, you'll unlock full access to this and other free tutorials on JSM Pro.
Why? Logging in lets us personalize your learning experience, track your progress, and keep you in the loop with new workshops, coding tips, and platform updates.
You'll also be the first to know about upcoming launches, events, and exclusive discounts.
No spam—just helpful content to level up your skills.
If that sounds fair, go ahead and log in to continue →
Enter your name and email to get instant access
##Looks like we found a thief monkey By the way, I liked the trick how you reached till here. You have a good sense of humor. You will improve a lot if you join our course with this passion.
var
(function-scoped, outdated)let
(block-scoped, modern and recommended)const
(block-scoped, cannot be reassigned)_
, or $
let let = 5;
is invalid)myVar
and myvar
are different)string
, number
, boolean
, null
, undefined
, bigint
, symbol
Objects
, Arrays
, Functions
Subscribing gives you access to a brief, insightful summary of each lecture to stay on track.
00:00:00 The last lesson was pretty interesting, right?
00:00:02 But now we're going to dive into something even more important than equality.
00:00:05 And that is the basic syntax and functionality of logical operators in JavaScript.
00:00:11 We can use them to combine multiple conditions.
00:00:14 So in simple words, logical operators are used to combine two or more conditions.
00:00:21 And JavaScript only has three logical operators.
00:00:24 or, and, and not.
00:00:27 The complete understanding of logical operators requires you to know about if and else statements and truthy and falsy values.
00:00:35 We haven't yet gotten to those.
00:00:36 So in this lesson, I'll cover the syntax of logical operators and then we'll revisit them in more detail after covering those topics.
00:00:45 First, let's talk about the double ampersand operator.
00:00:49 or better known as the AND operator.
00:00:52 It checks whether all operands are truthy values.
00:00:56 If they are, it returns true.
00:00:58 Otherwise, it returns false.
00:01:00 So, if we try to compare true and false, obviously the result is false, because one of these operators is, well, false.
00:01:09 If we compare true and true, we're going to get true.
00:01:12 And if we compare false and false, we're going to also get false.
00:01:16 So it is true only when both operands are true.
00:01:21 What you can also do is pass multiple conditions, like you're chaining them together.
00:01:26 Like you can pass another true and maybe even another.
00:01:31 And they're going to be true as long as there is not a single false in line.
00:01:36 but as soon as there's one false, then the entire condition turns into false.
00:01:42 no matter where it is like we can also have it maybe somewhere here in between but as soon as we hit false the result of that comparison will be false
00:01:52 so just to recap the double ampersand known as the AND operator checks whether all operands are truthy values and if they are it returns true otherwise
00:02:03 it returns false after the AND we also have the OR operator or the double vertical bar it checks whether at least One operand is a truthy value.
00:02:16 If there is at least one truthy value, it returns true, otherwise it returns false.
00:02:22 So in this case, not only true and true will return true, rather true and false, where there is one true value returns true,
00:02:31 as well as two true values, true and true, also return true.
00:02:35 The only case where the OR operator will return false is if both operands are falsy.
00:02:41 And as before, you can also pass multiple conditions, such as false, false, and false.
00:02:49 It'll still be false, but as soon as there's at least one true, it'll immediately convert over to true.
00:02:55 And finally, we have the NOT operator, or also known as the exclamation mark.
00:03:02 It reverses the Boolean result of a condition.
00:03:04 Pretty simple, right?
00:03:06 If you have a truthy value or true, and you add an exclamation mark or not before it, it's like you're basically saying,
00:03:13 that's not true, which means that it is false.
00:03:16 Or if you're saying that's not false, then it must mean that it is true.
00:03:21 In simple words, the not operator simply converts Boolean values.
00:03:25 You'll see yourself using all these three logical operators quite often when you're developing your JavaScript apps.
00:03:31 There's the AND operator, where everything has to be true for the result to be true.
00:03:36 There's the OR operator, where at least one value has to be true.
00:03:40 And then there's the NOT operator, which simply reverses the Boolean result.