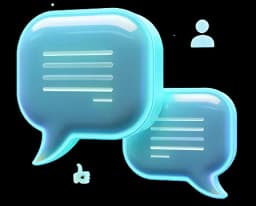
Join the Conversation!
Subscribing gives you access to the comments so you can share your ideas, ask questions, and connect with others.
In this lesson, you'll learn about the differences between strict and loose equality in JavaScript, and why understanding these concepts is crucial for writing reliable code.
Equality is a fundamental concept in JavaScript. We say two values are equal when they are the same value. For example:
console.log("This is a string." === "This is a string."); // true
console.log(2 === 2); // true
Note that we use three equal signs to represent this concept of equality in JavaScript.
JavaScript also allows us to test loose equality. It is written using two equal signs . Things may be considered loosely equal even if they refer to different values that look similar. An example would be:
console.log(5 == "5"); // true
Let's explore each one in more detail:
The strict equality method of comparison is a preferred option because its behavior can be easily predicted, which leads to fewer bugs and unexpected results. The JavaScript interpreter compares the values as well as their types and only returns true when both are the same.
console.log(20 === "20"); // false
The code above will print because even though the values seem to be the same, they are of different types. The first one is of type String and the second is of type Number.
Here's just one short thing that I wanted to show you. If we strictly compare objects, we're never going to get true. Let's test it out:
console.log({} === {}); // false
// we get false, even though they have the same type and content, weird
// the same thing happens for arrays as they are actually objects under the hood
5console.log([] === []); // false
For the sake of simplicity, we're not going to go into too much depth about non-primitive data types like objects and arrays. That is a rather complex topic on its own. Because of that, later in the course we have a whole separate section called "Value vs Reference." In there, we're going to explore the mentioned inconsistencies of the equality operator.
We write loose equality using a double equal sign . It uses the same underlying logic as strict equality except for a minor, yet huge, difference.
The loose equality doesn’t compare the data types.
You should almost never use the loose equality .
Douglas Crockford, in his excellent book JavaScript: The Good Parts, wrote:
JavaScript has two sets of equality operators: and , and their evil twins and .
The good ones work the way you would expect. If the two operands are of the same type and have the same value, then produces true and produces false.
The evil twins and do the right thing when the operands are of the same type, but if they are of different types, they attempt to change the values. The rules by which they do that are complicated and unmemorable. These are some of the interesting cases:
'' == '0' // false
0 == '' // true
0 == '0' // true
false == 'false' // false
false == '0' // true
false == undefined // false
false == null // false
null == undefined // true
Here are a few more examples:
Using the operator:
true == 1; // true, because 'true' is converted to 1 and then compared
"5" == 5; // true, because the string of "5" is converted to the number 5 and then compared
Using the operator:
true === 1; // false
"5" === 5; // false
That's exactly how it should be. On the other hand:
5 == "5" // true
This isn't and should never be equal. is a string, and should be treated like that. As I mentioned, most JavaScript developers completely avoid loose equality and rely only on strict equality . It is considered better practice and causes fewer bugs. From now on, you're going to see me use only strict equality .
And for the end, I found for you a great visual representation of strict versus loose equalities:
JavaScript Equality TableAs you can see, using the loose equality we get these green boxes all over the place. They're unpredictable. But if we switch to the strict equality , we get this nice predictable line.
So what's the moral of the story? Always use three equal signs .
"Please login to view comments"
Subscribing gives you access to the comments so you can share your ideas, ask questions, and connect with others.
How did you manage to remove the blur property and reach here?
Upgrading gives you access to quizzes so you can test your knowledge, track progress, and improve your skills.
By logging in, you'll unlock full access to this and other free tutorials on JSM Pro.
Why? Logging in lets us personalize your learning experience, track your progress, and keep you in the loop with new workshops, coding tips, and platform updates.
You'll also be the first to know about upcoming launches, events, and exclusive discounts.
No spam—just helpful content to level up your skills.
If that sounds fair, go ahead and log in to continue →
Enter your name and email to get instant access
##Looks like we found a thief monkey By the way, I liked the trick how you reached till here. You have a good sense of humor. You will improve a lot if you join our course with this passion.
var
(function-scoped, outdated)let
(block-scoped, modern and recommended)const
(block-scoped, cannot be reassigned)_
, or $
let let = 5;
is invalid)myVar
and myvar
are different)string
, number
, boolean
, null
, undefined
, bigint
, symbol
Objects
, Arrays
, Functions
Subscribing gives you access to a brief, insightful summary of each lecture to stay on track.
00:00:00 As promised, let me teach you what is the difference between strict and loose equality in JavaScript.
00:00:05 Equality is a fundamental concept in JavaScript, and we can say two values are equal when they are the same value.
00:00:14 For example, if we say this is a string and make it equal to this is a string, the output will of course be true because those two are the same.
00:00:23 Similarly, If you try to compare the number 2 to another number 2, the result will of course be true.
00:00:30 Now notice how we're using a triple equal sign right here to represent this concept of equality in JavaScript.
00:00:37 But JavaScript also allows us to test something known as loose equality.
00:00:43 It is written by using two equal signs.
00:00:45 So if I remove an additional equal sign from both of these, the result will of course still be true.
00:00:51 So what is the difference between strict and loose equality?
00:00:55 Well, things may be considered loosely equal even if they refer to different values that look similar.
00:01:02 An example of that would be comparing a number 5 to a string of 5. If we do this test, you can see that we get back true.
00:01:13 Even though they are not the same values, they feel similar.
00:01:18 But of course, that would not pass under strict equality, because if we add another equal sign and save it, you can see that now the last result is false.
00:01:27 So the question is, which one should you use?
00:01:30 Well, the strict equality operator almost always.
00:01:34 It is a preferred option because its behavior can be more easily predicted, which leads to fewer bugs and unexpected results.
00:01:42 When using the strict equality, the JavaScript interpreter compares both the values as well as their types and only returns true when both are the same.
00:01:52 You can think of the strict equalities as the good guys.
00:01:56 that'll look something like this, strict equality and strict inequality.
00:02:01 And then you can look at the other guys as the evil twins, which would be loose equality and loose inequality.
00:02:09 Now, why are they the evil twins?
00:02:11 Well, that's because it's hard to guess what the result is.
00:02:15 Let me ask you a couple of questions.
00:02:17 If we try to console.log the strict equality of an empty string with a string of zero, what do you think the output will be?
00:02:27 Are these two the same?
00:02:29 Well, of course not, right?
00:02:30 So the answer should be false.
00:02:33 And indeed, we do get the false.
00:02:36 But take a look at this.
00:02:37 See what the evil twins give us?
00:02:39 They give us false as well.
00:02:41 Okay, that's good.
00:02:42 So the evil twins here are not that evil, right?
00:02:45 But what happens if we try to console.log an empty string with a number of zero, so not a string of zero.
00:02:53 Well, you would say that's even clearer.
00:02:55 This is obviously false, right?
00:02:58 Well, not really.
00:02:59 For some reason, that is true, given the loose equality, because it tries to convert this into some kind of a falsi value,
00:03:07 and the zero is also falsi by default, so we get something that looks like this.
00:03:12 But if we make that a strict equality, we're gonna get the results we predict we're gonna get.
00:03:18 False.
00:03:18 Because these are obviously not the same.
00:03:20 There's more examples I can give you.
00:03:22 What if you compare the number 0 to a string of 0? You already know that.
00:03:27 We have tested with that before.
00:03:29 But obviously, this should be false.
00:03:32 But with double equality, it turns to be true.
00:03:35 But with triple equality, it'll be false.
00:03:37 What about this one?
00:03:38 maybe comparing something like a boolean false with a string of false.
00:03:43 What do you think what this one will be?
00:03:45 Well, you might expect that these are two different things, right?
00:03:48 But then you might say, hey, maybe the loose equality will actually give us true on this, right?
00:03:53 Like it give us true on this one.
00:03:55 But if we save it, no, for some reason, this one is false with both loose and strict equality.
00:04:02 So what I'm trying to say is that it's quite unpredictable.
00:04:06 And instead of doing all the guesswork, it's always better to use the triple equality to know exactly what you're going to get.
00:04:13 I also found a great visual representation of strict versus loose equalities.
00:04:18 As you can see, using the double equals or loose equality, we get these green boxes all over the place.
00:04:25 For example, comparing one to true or zero to false and so on.
00:04:30 It's quite unpredictable.
00:04:32 But if we switch this over to strict equality, you get this nice, super predictable line.
00:04:38 So, what is the moral of the story?
00:04:40 Well, always use triple equals unless you have a very good reason to use two.
00:04:46 Oh, and you may be wondering, but what if we tried comparing some objects?
00:04:50 That's a very good question.
00:04:52 If I console.log the comparison of an empty object with another empty object, what do you think the output will look like?
00:05:01 They're practically the same, right?
00:05:03 So we're expecting true with both loose and strict equality, right?
00:05:08 Well, not really.
00:05:09 It's false.
00:05:10 And the same thing happens with two empty objects.
00:05:13 Even our editor tells us that.
00:05:15 This condition will always return false since JavaScript compares objects by reference, not value.
00:05:22 Even though the content is the same and the type is the same, the output is false.
00:05:27 For the sake of simplicity, we're not going to dive into too much depth about comparing non-primitive data types like objects and arrays.
00:05:35 That is a rather complex topic on its own.
00:05:37 Because of that, later in the course, we're going to have a whole separate module called the value versus reference.
00:05:44 In there, we're going to explore the mentioned inconsistencies of the equality operator when using complex data types.
00:05:51 But with that said, for now, just remember the moral of the story.
00:05:55 Always use triple equal signs unless you have a good reason to use two.