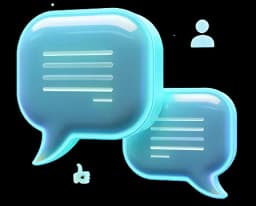
Join the Conversation!
Subscribing gives you access to the comments so you can share your ideas, ask questions, and connect with others.
In this lesson, you'll learn about reversing, repeating, and trimming strings in JavaScript, which are useful operations for manipulating text.
Let's explore how to perform these common string operations.
There isn't a built-in string method that reverses a string directly. However, we can use the knowledge we've previously gained! Remember how we can split a string into an array of characters ? Arrays have a method.
Here's the process:
const exampleString = 'test';
const reversedString = exampleString.split('').reverse().join('');
console.log(reversedString); // "tset"
If you want to repeat a string a certain number of times, you can easily do that using the method.
const dogSays = 'woof';
console.log(dogSays.repeat(5)); // "woofwoofwoofwoofwoof"
Sometimes, users might accidentally add extra spaces to their input, such as emails or usernames. We can clean these empty spaces using the method.
const str = " Hello World! ";
console.log(str.trim()); // "Hello World!"
These string operations are powerful tools for text manipulation in JavaScript, helping you clean, format, and transform strings as needed.
"Please login to view comments"
Subscribing gives you access to the comments so you can share your ideas, ask questions, and connect with others.
How did you manage to remove the blur property and reach here?
Upgrading gives you access to quizzes so you can test your knowledge, track progress, and improve your skills.