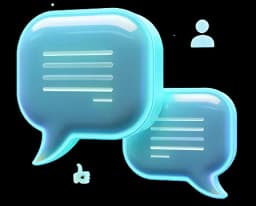
Join the Conversation!
Subscribing gives you access to the comments so you can share your ideas, ask questions, and connect with others.
In this lesson, you'll learn about various methods to search for substrings within a string in JavaScript, which are useful for checking the presence and position of text.
There are multiple ways to look for a substring within a string in JavaScript. Let's explore some of the most common methods.
The first method is . It searches for the in , starting from the given position , and returns the position where the match was found or if nothing can be found.
For instance:
const exampleString = 'I love ducks, he said, ducks are great!';
console.log(exampleString.indexOf('ducks')); // 7
console.log(exampleString.indexOf('Ducks')); // -1
The optional second parameter allows us to search starting from the given position. For instance, the first occurrence of is at position . To look for the next occurrence, let’s start the search from position :
console.log(exampleString.indexOf('ducks', 8)); // 23
is similar to , but it searches from the end of a string to its beginning.
console.log(exampleString.lastIndexOf('ducks')); // 23
If you're just interested in whether a string contains a substring, and not its position, you can use . It simply returns or .
console.log(exampleString.includes('ducks')); // true
As with the method, the optional second argument of is the position to start searching from.
The methods and check if a string starts or ends with a specific substring:
console.log(exampleString.startsWith('I')); // true
console.log(exampleString.endsWith('ducks')); // false
These methods provide powerful ways to search and check for substrings within strings, making it easier to manipulate and analyze text in JavaScript.
"Please login to view comments"
Subscribing gives you access to the comments so you can share your ideas, ask questions, and connect with others.