Lifetime access to this course
Strings Introduction
In this lesson, you'll learn about strings in JavaScript, which are used to store and manipulate text. ### Strings in JavaScript In JavaScript, and in any programming language for that matter, we need a way to store text. In JavaScript, we use strings to store text. A string is a primitive data type that represents a sequence of characters. ### Creating Strings
The rest of this lesson is waiting.
Join JS Mastery Pro to unlock it.
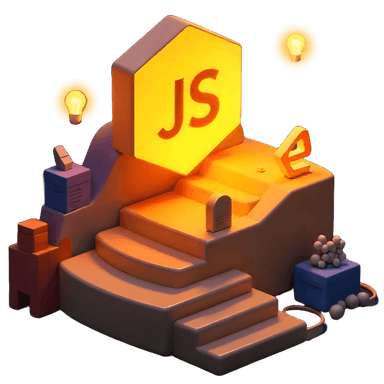
Complete Path to JavaScript Mastery
$300
Buy the Course OnlyBuild advanced web app
Certificate of completion included
Great for focused learners who just want this course
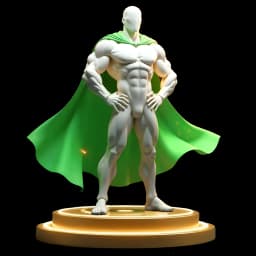
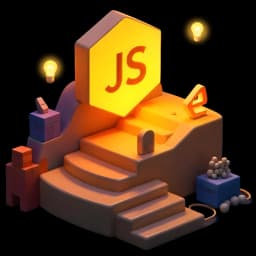
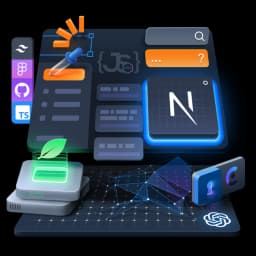
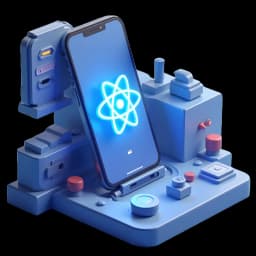
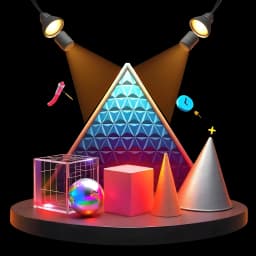
Get Full Access
$120 /month
Lock in a year & save—2 months free!
Access to all courses
Quizzes, mini-challenges, AI summaries, inline sandboxes
Participation in a private community
Build real production-grade apps
Mock interviews & project reviews
Weekly live sessions & member-only access