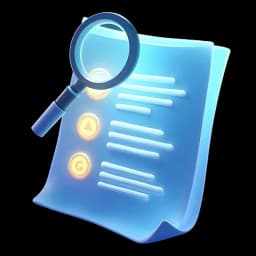
Quick Lecture Overview
Subscribing gives you access to a brief, insightful summary of each lecture to stay on track.
In this lesson, you'll learn about scope in JavaScript, a fundamental concept that determines the accessibility of variables, functions, and objects in different parts of your code.
Scope allows us to know where we have access to our variables. It shows us the accessibility of variables, functions, and objects in some particular part of the code.
Variables defined inside a function are in local scope , while variables defined outside of a function are in the global scope . Each function, when invoked, creates a new scope.
There are rules about how scope works, but usually, you can search for the closest { } curly braces around where you define the variable. That “block” of code is its scope .
When you start writing in a JavaScript document, you're already in the global scope.
const name = 'Adrian';
Variables written inside the global scope can be accessed and altered in any other scope.
const logName = () => {
console.log(name);
}
logName();
Variables defined inside a function are in the local scope.
// Global Scope
const someFunction = () => {
// Local Scope #1
const anotherFunction = () => {
// Local Scope #2
}
}
Block statements like , , and loops don't create a new scope with .
However, variables defined with or have block scope, meaning they are only available inside the block of code where they are created.
if (true) {
var name = 'Adrian'; // global scope
let likes = 'Coding'; // block scope
const skills = 'JavaScript and PHP'; // block scope
}
console.log(name); // logs 'Adrian'
console.log(likes); // Uncaught ReferenceError: likes is not defined
console.log(skills); // Uncaught ReferenceError: skills is not defined
Both and are important. However, a large number of global variables can occupy a lot of memory and make changes difficult to track.
It's advisable to avoid declaring unnecessary global variables and always declare variables in the scope where you intend to use them .
Understanding is crucial for writing efficient and error-free JavaScript code. It helps you manage variable accessibility and avoid potential conflicts.
By logging in, you'll unlock full access to this and other free tutorials on JSM Pro.
Why? Logging in lets us personalize your learning experience, track your progress, and keep you in the loop with new workshops, coding tips, and platform updates.
You'll also be the first to know about upcoming launches, events, and exclusive discounts.
No spam—just helpful content to level up your skills.
If that sounds fair, go ahead and log in to continue →
Enter your name and email to get instant access
##Looks like we found a thief monkey By the way, I liked the trick how you reached till here. You have a good sense of humor. You will improve a lot if you join our course with this passion.
var
(function-scoped, outdated)let
(block-scoped, modern and recommended)const
(block-scoped, cannot be reassigned)_
, or $
let let = 5;
is invalid)myVar
and myvar
are different)string
, number
, boolean
, null
, undefined
, bigint
, symbol
Objects
, Arrays
, Functions
Subscribing gives you access to a brief, insightful summary of each lecture to stay on track.
00:00:00 Let's talk about scope.
00:00:01 So what is it?
00:00:03 Well, scope allows you to know where you have access to your variables.
00:00:08 It shows us the accessibility of variables, functions, and objects in a particular part of the code.
00:00:16 But why would you want to limit the visibility of a variable in the first place?
00:00:20 Well, there's a couple of reasons.
00:00:22 First, security, because limiting the visibility of variables provides a level of security to your code.
00:00:28 Then there's the efficiency, as it helps you track bugs faster and reduce them.
00:00:33 And of course, the naming conflicts.
00:00:36 It completely solves the problem of naming variables, because imagine having a huge code base and not being able to create two variables of the same name.
00:00:44 that would be a mess.
00:00:45 So, we gotta understand how scope works.
00:00:49 There are a couple of different types of scope.
00:00:52 A global scope, a local scope, and a block scope.
00:00:56 See, variables defined inside a function are considered to be in a local scope, while variables defined outside of a function are in the global scope.
00:01:08 Each function on its own, when invoked, creates a new scope.
00:01:13 There are rules about how scope works, but let me tell you a secret.
00:01:17 The simplest way to find a scope is to search for the closest opening and closing curly brace around where you define the variable.
00:01:26 That block of code is its scope.
00:01:29 So let's dive deeper into the global scope.
00:01:31 When you start writing in a JavaScript document, you're already in the global scope.
00:01:36 So if I declare a variable like first name equal to Adrian right here.
00:01:42 and I try to console log it right below, of course I will have access to it because you have access to everything within the global scope.
00:01:50 But check this out.
00:01:52 Even if you create a new function called logName and make it equal to an arrow function that then console logs the same first name that we tried to console
00:02:04 log before right above it, now within this function scope, Will this work?
00:02:10 Well, let's execute the function and let's find out.
00:02:14 Yes, it works.
00:02:16 That's because the variables that are written inside of the global scope can also be accessed and altered in any other scope.
00:02:24 Think of it as the most important scope.
00:02:26 So what are some of the advantages of using global variables?
00:02:29 Well, you saw it here.
00:02:31 You can access it from anywhere, all the functions or modules in your program.
00:02:36 It's great for consistency if you want to store some constants that you want to use throughout the entire application.
00:02:42 And once again, it's great for data sharing.
00:02:45 For example, when you want to create a new function right below it, and you want to be able to use the same variable that you declared above.
00:02:53 But with great power comes great responsibility, right?
00:02:56 So there must be some disadvantages.
00:02:58 Well, of course.
00:03:00 Too many variables declared as global remain in the memory until the program execution is completed, which can cause out-of-memory issues.
00:03:09 Also, more importantly, it can lead to incredibly unpredictable results.
00:03:13 Why?
00:03:14 Well, because you can modify that global variable from any function.
00:03:18 Let me show you what I mean.
00:03:19 If I have function log name and I try to change this first name from within the function to something like John, or let's first consulog it here.
00:03:28 First, we have to change it to a let to be able to change it.
00:03:32 And then you'll notice that first, it consulogs Adrian, and then in the second one, it consulogs John, which leads to unpredictability.
00:03:42 See, it's the same variable, but we execute it two different times, and it has an entirely different value.
00:03:49 And the last disadvantage of using global variables is just refactoring challenges.
00:03:54 If at one point you no longer need this global variable, then you will need to change its occurrence across all the different functions that are calling it,
00:04:03 or else you're going to get a lot of errors.
00:04:05 So what's the alternative?
00:04:07 Well, we can use the local scope.
00:04:10 In simple terms, variables defined inside the function are in the local scope.
00:04:16 So for example, if I declare a function like this, const some function, this place within it, in between those two curly braces,
00:04:25 is considered a local scope.
00:04:27 So I'll say local scope number one.
00:04:30 Now, if I declare a variable first name right here within this local scope, Will I be able to use it right below?
00:04:39 What do you think?
00:04:40 Well, let's execute the function.
00:04:42 And of course, the answer is yes, we can use it.
00:04:46 But now, what if you try to use this same variable outside of this scope?
00:04:51 Let me show you what happens.
00:04:53 I will simply move this console log out of it and run it.
00:04:57 And as you can see, JavaScript doesn't know about the first name within the global scope because the variable is contained within this block or function scope.
00:05:07 And even if you hover over it, it'll say first name is declared, but its value is never read.
00:05:12 Like it doesn't know we're trying to call it from here.
00:05:15 And that's exactly what the concept of scope is.
00:05:18 Think of these as different places from within which we're storing the variables and then different places from within which we're trying to call them.
00:05:27 So why would you ever use the local scope if it can cause the error of not knowing where the variable is?
00:05:33 Well, it has many advantages.
00:05:35 The first one is the data integrity.
00:05:38 The use of local variables offers a guarantee that the values of variables will remain intact while the task is running.
00:05:47 You cannot change it from outside of that local scope.
00:05:51 Next, we have the naming flexibility.
00:05:54 You can give local variables the same name in different functions because they're only recognized by the function that they're declared in.
00:06:02 What do I mean by that?
00:06:03 Well, if we have one function with its own local scope, and we have another function, I'll call it some function too, with its own scope.
00:06:12 I can declare the same first name variable and make it of different value.
00:06:17 See, we have two different first names.
00:06:20 One is console logging Adrian, and the other is console logging John, and they both return different values.
00:06:27 No naming conflicts.
00:06:29 And the third advantage of using local variables is just memory efficiency.
00:06:34 Local variables are deleted as soon as the function is executed, releasing the memory space that they occupy.
00:06:40 And when it comes to disadvantages, there aren't many.
00:06:44 The only disadvantage is, well, the limited scope.
00:06:48 If you need to use a variable in the parent scope, like right here in the global scope in this case, then you have to declare it there.
00:06:55 And then there's the third type of scope called the block scope.
00:07:00 Block statements like ifs, fors, and while loops don't create a new scope with the var keyword.
00:07:08 However, if you use let and const to declare variables, which of course you should, nobody uses var anymore, then these variables will have a so-called
00:07:19 block scope, meaning that they are only available inside of the block of code where they're created.
00:07:25 Let me give you an example.
00:07:27 const, is this a block of code?
00:07:33 I'll make it equal to a boolean variable, and then I'll open up an if statement, and then I'll execute it if this is true.
00:07:42 Is this a block of code?
00:07:43 Once again, even though this is not a function, as I told you, you can see the opening and closing curly brace denoting that this is indeed a block of
00:07:53 code with its own scope.
00:07:55 So if you try to create a variable of first name by using the var keyword, which I told you never to use, and then you try to console log this variable
00:08:08 outside in the global scope, Check this out.
00:08:11 It actually works, right?
00:08:13 But if you try to define it with the let keyword, it'll not work because let and const create their own block scope, which is actually preferable,
00:08:25 right?
00:08:26 That's one of the primary reasons of why we decided to ditch the var keyword in the first place.
00:08:31 See, it is within a block, but it makes itself accessible in the global scope.
00:08:36 Doesn't make sense.
00:08:37 Just do what you're supposed to do.
00:08:39 const and let do that nicely.
00:08:41 They allow you to use it from within the scope that it has been defined within.
00:08:46 So, which scope is the best?
00:08:48 Well, both local and global variables are important.
00:08:53 However, a large number of global variables can take up a lot of memory and make things difficult to track.
00:09:00 I would recommend to avoid declaring unnecessary global variables and always declare variables in the scope where you intend to use them.
00:09:11 Let me repeat that, always declare variables in the scope where you intend to use them.
00:09:18 If you later on need to use them from within outside of that scope, then bring them one level up.
00:09:24 But first, start from the smallest possible scope and then bring it to the outside.
00:09:28 As I told you, understanding how scope works is super important for writing efficient and error-free JavaScript code.
00:09:36 because it helps you manage from where you can access the variables.
00:09:39 And if you're writing quality code, meaning you only create variables from within where they have to be used, well, that'll help you avoid a lot of bugs
00:09:49 and conflicts in your code.