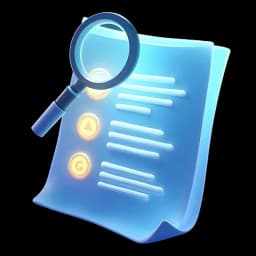
Quick Lecture Overview
Subscribing gives you access to a brief, insightful summary of each lecture to stay on track.
In this lesson, you'll learn about deep cloning in JavaScript, which is essential for creating independent copies of complex objects without maintaining references to the original.
Deep cloning involves creating a complete copy of an object, including all nested objects, so that changes to the new object do not affect the original. This is crucial when working with complex data structures.
Consider the following object:
const person = {
firstName: 'Emma',
car: {
brand: 'BMW',
color: 'blue',
wheels: 4,
}
};
We can use the spread operator to create a shallow copy of the object:
const newPerson = { ...person };
This removes the reference from the outer object, allowing us to change properties like without affecting the original:
newPerson.firstName = 'Mia';
console.log(person); // { firstName: 'Emma', car: { brand: 'BMW', color: 'blue', wheels: 4 } }
console.log(newPerson); // { firstName: 'Mia', car: { brand: 'BMW', color: 'blue', wheels: 4 } }
However, if we change a property of the nested object:
newPerson.car.color = 'red';
console.log(person); // { firstName: 'Emma', car: { brand: 'BMW', color: 'red', wheels: 4 } }
console.log(newPerson); // { firstName: 'Mia', car: { brand: 'BMW', color: 'red', wheels: 4 } }
Both objects are affected because the object is still referenced.
To create a deep clone, we need to remove references from all nested objects. One way to achieve this is by using and .
const newPerson = JSON.parse(JSON.stringify(person));
This method converts the object to a string and then back to an object, effectively removing all references.
Let's test the deep clone by modifying the new object:
newPerson.firstName = 'Mia';
newPerson.car.color = 'red';
console.log(person); // { firstName: 'Emma', car: { brand: 'BMW', color: 'blue', wheels: 4 } }
console.log(newPerson); // { firstName: 'Mia', car: { brand: 'BMW', color: 'red', wheels: 4 } }
The original object remains unchanged, confirming that is a deep clone.
Deep cloning is a powerful technique for working with complex objects in JavaScript. By using and , you can create independent copies of objects, ensuring that changes to one do not affect the other. Mastering this concept is crucial for managing data effectively in your applications. If you're still a bit confused, take your time to review this section and practice with different examples. Mastery takes time!
By logging in, you'll unlock full access to this and other free tutorials on JSM Pro.
Why? Logging in lets us personalize your learning experience, track your progress, and keep you in the loop with new workshops, coding tips, and platform updates.
You'll also be the first to know about upcoming launches, events, and exclusive discounts.
No spam—just helpful content to level up your skills.
If that sounds fair, go ahead and log in to continue →
Enter your name and email to get instant access
##Looks like we found a thief monkey By the way, I liked the trick how you reached till here. You have a good sense of humor. You will improve a lot if you join our course with this passion.
var
(function-scoped, outdated)let
(block-scoped, modern and recommended)const
(block-scoped, cannot be reassigned)_
, or $
let let = 5;
is invalid)myVar
and myvar
are different)string
, number
, boolean
, null
, undefined
, bigint
, symbol
Objects
, Arrays
, Functions
Subscribing gives you access to a brief, insightful summary of each lecture to stay on track.
00:00:00 In the last lesson, when I showed you how to shallow clone a razor object, you thought, okay, this is great.
00:00:05 Spread operator is all I need.
00:00:07 But then, unfortunately, I just had to come in and ruin your dreams.
00:00:12 You need to learn how to do deep cloning too.
00:00:15 See, deep cloning involves creating a complete copy of an object.
00:00:20 including all nested objects so that changes to the new object do not affect the original, even on a deeper level.
00:00:28 This is crucial when working with more complex data structures.
00:00:32 Let me give you an example.
00:00:34 Let's say that we have a person with a first name of Emma, and Emma drives a blue BMW.
00:00:40 Now if you use the spread operator to copy that person, which does remove the reference from the outer object, allowing us to change the properties like
00:00:49 first name without affecting the original.
00:00:51 So if I go ahead and change the name of the new person to something like Mia, and I console log both of these people, I'll say person and other person
00:01:01 or new person in this case, you'll see that the first name changes.
00:01:05 But as soon as I try to change Mia's car color from blue to red, that doesn't work.
00:01:13 Emma's car color will change to red as well, which means that the both objects are affected because the car object is still referenced.
00:01:22 So we have to create something known as a deep clone.
00:01:26 And to create a deep clone, we need to remove references from all nested objects.
00:01:32 One way to achieve this is by using the JSON.stringify() and JSON.parse() methods.
00:01:39 See, instead of spreading the person, I can do something like const newPerson is equal to JSON.parse() json.stringify and then pass in the person.
00:01:51 What this does is it converts this object of person into a string, which removes all the references, and then it parses it back into an object,
00:02:03 effectively removing all references.
00:02:05 So if we test it out now, you can see that we can change the first name to Mia, but also her car color.
00:02:12 So if I check out the output, you can see that Emma's car remains blue.
00:02:17 This means that we have effectively deep cloned an object.
00:02:20 The original person object remains unchanged, confirming that the new person is a deep clone.
00:02:27 See, this is a powerful technique for working with complex objects in JavaScript.
00:02:32 By using JSON Stringify and then JSON Parse, you create independent copies of objects, ensuring that changes to one do not affect the other.
00:02:43 Mastering this concept is crucial for managing data effectively in your applications.
00:02:49 And if you're still a bit confused, take your time to review this entire module and practice with different example, because mastery takes time and practice.
00:02:59 Great work on coming to the end of this module that I wouldn't say is hard, but it's definitely tough to understand as it touches the concepts of memory,
00:03:08 references, and values and how those values are stored.
00:03:12 Great work.