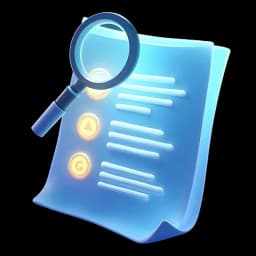
Quick Lecture Overview
Subscribing gives you access to a brief, insightful summary of each lecture to stay on track.
In this lesson, you'll learn about the concept of reference in JavaScript, particularly how it applies to objects and arrays. This understanding is crucial for managing data and avoiding unintended side effects in your code.
In our previous lesson, we explored how primitive values are copied by value, meaning each variable gets its own copy of the data. However, when it comes to non-primitive values like objects and arrays, JavaScript uses references .
Let's revisit the example from our last lesson:
const person = {
firstName: 'Jon',
lastName: 'Snow',
};
const otherPerson = person;
person.firstName = 'JOHNNY';
console.log(person); // { firstName: 'JOHNNY', lastName: 'Snow' }
console.log(otherPerson); // { firstName: 'JOHNNY', lastName: 'Snow' }
When a variable is assigned a primitive value, it simply copies that value. We saw this with numbers and strings. However, when a variable is assigned a non-primitive value (such as an object, array, or function), it is given a reference to that object’s location in memory.
In the example above, the variable doesn’t actually contain the value .
Instead, it points to a location in memory where that value is stored.
const otherPerson = person;
When a reference type value is copied to another variable, like , the object is copied by reference instead of value. In simple terms, and don’t have their own copy of the value. They point to the same location in memory.
person.firstName = 'JOHNNY';
console.log(person); // { firstName: 'JOHNNY', lastName: 'Snow' }
console.log(otherPerson); // { firstName: 'JOHNNY', lastName: 'Snow' }
When a property is modified on , the object in memory is updated, and as a result, also reflects that change.
We can demonstrate this behavior with a simple equality check:
const person = { firstName: 'Jon' };
const otherPerson = { firstName: 'Jon' };
console.log(person === otherPerson); // FALSE
You might expect to resolve to true because they look identical. However, they point to two distinct objects stored in different locations in memory.
Now, let's create a copy of the object by copying the reference:
const anotherPerson = person;
console.log(person === anotherPerson); // TRUE
and hold references to the same location in memory and are therefore considered equal.
We've learned that primitive values are copied by value, while objects and arrays are copied by reference. This means that changes to one reference affect all references pointing to the same object. In the next lesson, we'll explore how to make a true copy of an object, allowing you to modify one without affecting the other. Understanding these concepts is essential for writing robust and predictable JavaScript code.
By logging in, you'll unlock full access to this and other free tutorials on JSM Pro.
Why? Logging in lets us personalize your learning experience, track your progress, and keep you in the loop with new workshops, coding tips, and platform updates.
You'll also be the first to know about upcoming launches, events, and exclusive discounts.
No spam—just helpful content to level up your skills.
If that sounds fair, go ahead and log in to continue →
Enter your name and email to get instant access
##Looks like we found a thief monkey By the way, I liked the trick how you reached till here. You have a good sense of humor. You will improve a lot if you join our course with this passion.
var
(function-scoped, outdated)let
(block-scoped, modern and recommended)const
(block-scoped, cannot be reassigned)_
, or $
let let = 5;
is invalid)myVar
and myvar
are different)string
, number
, boolean
, null
, undefined
, bigint
, symbol
Objects
, Arrays
, Functions
Subscribing gives you access to a brief, insightful summary of each lecture to stay on track.
00:00:00 In our previous lesson, we explored how primitive values are copied by value, which means that each variable gets its own copy of the data.
00:00:09 However, when it comes to non-primitive values, like objects and arrays, JavaScript uses references.
00:00:17 So let's revisit the same example from the last lesson.
00:00:21 What really happened here?
00:00:23 Well, when a variable is assigned a primitive value, it simply copies that value.
00:00:29 We saw this with numbers and strings, but when a variable is assigned a non-primitive value, which is what we're doing here,
00:00:38 such as an object or an array or even a function, It is given a reference to that object's location in the memory.
00:00:46 In this example, the variable otherPerson doesn't really contain the value of firstName and lastName.
00:00:54 Instead, it points to a location in the memory where that value is stored.
00:01:00 So let's say that this person object, which I will put in a single line just for easier readability.
00:01:06 Yep, you can totally do that, is stored.
00:01:09 on a memory location of hash123ABC.
00:01:14 When we make the other variable equal to this variable, it does not paste this value into it.
00:01:21 What it does is it attaches it to the same memory location of where that value is stored.
00:01:28 So now, those two objects become sort of binded.
00:01:31 When you change one, you're also changing the other because they point to the same location in the memory.
00:01:37 So in simple terms, person and other person don't have their own copy of the value.
00:01:43 They point to the same location in the memory.
00:01:46 Having understood that, now it should be clear to you why when a property like firstName on the person object is modified,
00:01:54 the object in the memory is updated, and as a result, the other person will also reflect that change.
00:02:00 And I can actually demonstrate this behavior with a simple equality check.
00:02:05 Let me show you.
00:02:07 I'll create those two objects from scratch.
00:02:10 This time, I'll give it just the first name.
00:02:12 Let's start with something like first name of John.
00:02:16 And I'll also create the other person, and I'll make it equal to the same exact value.
00:02:22 You know what?
00:02:22 I won't even type it out.
00:02:24 I'll go ahead and copy it, and paste it right here.
00:02:28 Now, previously, we talked about the concept of equality.
00:02:32 So what do you think?
00:02:33 If I console log the output of the triple equality check between person and other person, what do you think?
00:02:42 Are they really the same or are they not?
00:02:45 You might expect that they're going to be the same because they look identical.
00:02:49 However, they point to two distinct objects stored in different locations in the memory.
00:02:56 So for example, this one is 123ABC, and the other one is ABC123.
00:03:01 Therefore, these two are not the same.
00:03:06 But what happens if instead of creating two separate objects, you create a reference to that object?
00:03:14 So you say other person is equal to the person.
00:03:17 Well, as you know, then both of them will be stored in the same location in the memory, and the output will be true.
00:03:24 Because both person and another person hold the same reference to the same location in memory, and are therefore considered equal.
00:03:33 So, what's the takeaway?
00:03:34 Well, we've learned that primitive values are copied by value.
00:03:38 while objects and arrays are copied by reference.
00:03:42 This means that changes to one reference affect all references pointing to the same object.
00:03:48 But hey, what if you want to copy the object and change it without affecting the initial value you copied it from?
00:03:55 Well, in the next lesson, I'll teach you how to make a true copy of an object, allowing you to modify one without affecting the other.
00:04:04 Understanding that will make you an even better JavaScript developer.