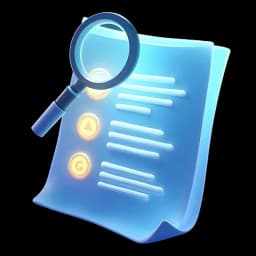
Quick Lecture Overview
Subscribing gives you access to a brief, insightful summary of each lecture to stay on track.
In this lesson, you'll learn about the difference between value and reference in JavaScript, which is crucial for understanding how data is stored and manipulated in your programs.
JavaScript differentiates data types into two categories:
Understanding how these types are copied and manipulated is key to avoiding unexpected behavior in your code.
When copying primitive values, JavaScript behaves as you might expect. The value is copied directly, and changes to one variable do not affect the other. Here are some examples:
let x = 1;
let y = x;
x = 2;
console.log(x); // 2
console.log(y); // 1
let firstPerson = 'Mark';
let secondPerson = firstPerson;
firstPerson = 'Austin';
console.log(firstPerson); // Austin
console.log(secondPerson); // Mark
In both cases, the original value is copied, and changes to the original variable do not affect the copied variable.
When copying complex values, such as objects and arrays, JavaScript behaves differently. Instead of copying the value, it copies a reference to the value. This means changes to one variable can affect the other .
const animals = ['dogs', 'cats'];
const otherAnimals = animals;
animals.push('llamas');
console.log(animals); // ['dogs', 'cats', 'llamas']
console.log(otherAnimals); // ['dogs', 'cats', 'llamas']
const person = {
firstName: 'Jon',
lastName: 'Snow',
};
const otherPerson = person;
person.firstName = 'JOHNNY';
console.log(person); // { firstName: 'JOHNNY', lastName: 'Snow' }
console.log(otherPerson); // { firstName: 'JOHNNY', lastName: 'Snow' }
In both examples, changes to the original array or object also affect the copied variable. This is because both variables reference the same underlying data.
This behavior can be surprising if you're not expecting it, but it makes sense once you understand that complex values are stored as references. In the next lesson, we'll dive deeper into why this happens and how you can manage it effectively in your code. Understanding the difference between value and reference is essential for writing robust and predictable JavaScript programs.
By logging in, you'll unlock full access to this and other free tutorials on JSM Pro.
Why? Logging in lets us personalize your learning experience, track your progress, and keep you in the loop with new workshops, coding tips, and platform updates.
You'll also be the first to know about upcoming launches, events, and exclusive discounts.
No spam—just helpful content to level up your skills.
If that sounds fair, go ahead and log in to continue →
Enter your name and email to get instant access
##Looks like we found a thief monkey By the way, I liked the trick how you reached till here. You have a good sense of humor. You will improve a lot if you join our course with this passion.
var
(function-scoped, outdated)let
(block-scoped, modern and recommended)const
(block-scoped, cannot be reassigned)_
, or $
let let = 5;
is invalid)myVar
and myvar
are different)string
, number
, boolean
, null
, undefined
, bigint
, symbol
Objects
, Arrays
, Functions
Subscribing gives you access to a brief, insightful summary of each lecture to stay on track.
00:00:00 Let's talk about the difference between value and the reference.
00:00:04 This is a general programming concept, and typically understanding it is only reserved to those who study computer science at a university.
00:00:12 But I believe that understanding how programming languages store values in the memory is important not only for languages like C or C++,
00:00:22 but also for JavaScript.
00:00:24 Even though JavaScript abstracts the way in which it stores the data in the memory and doesn't make you do that on your own,
00:00:31 it doesn't mean that you shouldn't understand how it works behind the scenes.
00:00:34 So, let me explain it.
00:00:36 See, in JavaScript, we have two different data type categories.
00:00:40 We talked about it already.
00:00:41 Primitive types, such as numbers, strings, booleans, nulls, and undefined, and complex values, which are objects and arrays.
00:00:50 You know that already, right?
00:00:52 But what happens when we try to copy different values?
00:00:55 Let's say that you're trying to copy primitive values, right?
00:00:58 I create a variable called let x and set it equal to 1, and then I create a variable of let y, which we can make equal to x.
00:01:08 Now, if I cons a log, x and y, as a matter of fact, let me do it in a new line so we can see exactly what each number is.
00:01:17 So I'll say x and then render it, and then I'll render y right below it.
00:01:23 If we do that, you'll notice that y takes the value of x, which makes sense, right?
00:01:28 But now, what happens if all of a sudden we change the value of x to something like 2? What do you think the output will be?
00:01:37 Well, we first set x to be 1, then we set y to be x and x is 1, which would mean that y is now 1, right?
00:01:46 And then we reset X to 2, so X is 2, Y is 1. Makes sense, right?
00:01:52 We get 2 and 1. Let me show you the same example using strings.
00:01:57 I'll create a variable of firstPerson and make it equal to mark.
00:02:03 And I'll also create a variable of secondPerson.
00:02:06 At the start, make it equal to the first person, and then I will reset the first person to something like Austin.
00:02:14 If I do that and console log both the first person as well as the second person, what do you think the output will be?
00:02:22 Well, the first person gets changed to Austin while the second person still remains Mark.
00:02:28 Again.
00:02:29 It is intuitive and it makes sense.
00:02:32 The original value is copied and changes to the original variable do not affect the copied variable.
00:02:39 But now, what happens when we copy complex values such as objects and arrays?
00:02:46 In that case, JavaScript behaves a bit differently.
00:02:49 Instead of copying the value itself, it instead copies a reference to the value.
00:02:55 This means that changes to one variable can affect the other.
00:03:00 Let me show you what I mean.
00:03:01 I'll say const animals and make it equal to an array of dogs and cats.
00:03:08 Then if I create an array of other animals and make it equal to the original array of animals, And if now I change the original array of animals by pushing
00:03:21 something like lambas to it, if we consulog the original animals array, it'll now include lambas.
00:03:28 Makes sense, right?
00:03:29 We just added it to it.
00:03:30 But remember the example with primitive values of strings and numbers.
00:03:34 If I now try to consulog the other animals, it shouldn't contain lambas, right?
00:03:39 Because we used its value before we pushed that additional one.
00:03:43 If I save it, well, check this out.
00:03:48 Both of the arrays now contain llamas.
00:03:50 So I'll call this something like arrayCopyingExample.
00:03:55 And I will also now, right below it, render an objectCopyingExample, just to show you that the same thing happens with objects.
00:04:04 I'll define a person object that'll have a first name of John and last name of Snow.
00:04:13 Now we can create another person, which is similar to John Snow.
00:04:17 So I'll say other person is equal to person.
00:04:21 And then if I call the person dot first name and change it to something like Johnny and icons a log the original person object Makes sense,
00:04:33 right?
00:04:34 We get first name Johnny last name snow But now if I cons a log the other person What do we get?
00:04:44 Well, his name also changed to Johnny, even though we changed the variable after we originally assigned it.
00:04:52 So in both of these cases, changes to the original array or object also affect the copied variable.
00:05:00 This is because both variables reference the same underlying data.
00:05:05 This behavior can be super surprising and a bit weird if you're not expecting it, but it makes complete sense once you understand that complex values are
00:05:16 stored as references.
00:05:17 In the next lesson, I'll tell you a bit more about why this happens and how you can manage it effectively in your code.
00:05:23 Because understanding the difference between a value and a reference is essential for writing robust and predictable JavaScript code.