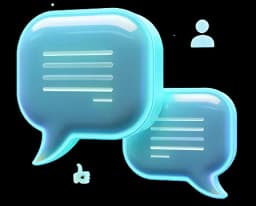
Join the Conversation!
Subscribing gives you access to the comments so you can share your ideas, ask questions, and connect with others.
Learning Objective: Understand what Booleans are, and how to use them to add basic logic to your programs.
A Boolean is a data type that can only have one of two values:
Booleans are essential for decision-making in programming. They help control the flow of your program, allowing it to act differently depending on conditions.
You can directly assign or to variables:
const isRaining = true;
const isWeekend = false;
Booleans are often used in statements to decide what code to execute:
const isRaining = true;
if (isRaining) {
console.log("Take an umbrella!");
} else {
console.log("Enjoy the sunshine!");
}
Booleans also play a key role in user interactions. For example:
const isLoggedIn = false;
if (isLoggedIn) {
console.log("Welcome back!");
} else {
console.log("Please log in.");
}
Boolean values often come from comparisons. For instance:
const age = 20;
console.log(age >= 18); // true
Comparisons return or , making them Booleans. We’ll dive into comparisons and logical operators in the Operators and Equality module.
Example:
const isBirthday = false;
const completedLesson = true;
if (isBirthday) {
console.log("Happy Birthday!");
}
if (completedLesson) {
console.log("Great job on completing today's lesson!");
}
In this lesson, you’ve learned how to use Booleans to control the flow of your program. Next, we’ll dive deeper into comparisons, logical operators, and how to combine multiple conditions in the Operators and Equality module.
Now, let’s dive into Null and Undefined!
"Please login to view comments"
Subscribing gives you access to the comments so you can share your ideas, ask questions, and connect with others.
By logging in, you'll unlock full access to this and other free tutorials on JSM Pro.
Why? Logging in lets us personalize your learning experience, track your progress, and keep you in the loop with new workshops, coding tips, and platform updates.
You'll also be the first to know about upcoming launches, events, and exclusive discounts.
No spam—just helpful content to level up your skills.
If that sounds fair, go ahead and log in to continue →
Enter your name and email to get instant access
##Looks like we found a thief monkey By the way, I liked the trick how you reached till here. You have a good sense of humor. You will improve a lot if you join our course with this passion.
var
(function-scoped, outdated)let
(block-scoped, modern and recommended)const
(block-scoped, cannot be reassigned)_
, or $
let let = 5;
is invalid)myVar
and myvar
are different)string
, number
, boolean
, null
, undefined
, bigint
, symbol
Objects
, Arrays
, Functions
Subscribing gives you access to a brief, insightful summary of each lecture to stay on track.
00:00:00 A Boolean is a data type that can only have one of two values.
00:00:05 True, meaning yes, correct, or on, and false, meaning no, incorrect, or off.
00:00:12 Booleans are essential for decision-making in programming.
00:00:16 They help control the flow of your program, allowing it to act differently depending on conditions.
00:00:24 So let me give you an example.
00:00:26 You can directly assign true or false to variables like, is it raining or is it the weekend?
00:00:33 You can then use those boolean variables to make conditions like within if statements to decide which code to execute.
00:00:41 Like if it is raining, then console log take an umbrella.
00:00:46 Else, console log enjoyed the sunshine.
00:00:48 In this case, of course, we're going to get back, take an umbrella, as is raining is true.
00:00:53 Therefore, we enter into this block of code.
00:00:57 Of course, in the real world, you might use Booleans like this.
00:01:01 You want to figure out if a user is logged in.
00:01:04 And if they are, you're gonna say, hey, welcome back.
00:01:07 But if they're not, they're gonna be prompted to log in.
00:01:11 Oh, and this is a pretty cool thing.
00:01:12 You don't necessarily have to explicitly declare a Boolean, like false or true.
00:01:17 Boolean values often come from comparisons.
00:01:20 For instance, if the age variable is set to 20, and you cons a log, age is greater than or equal to 18, The output of that will be true,
00:01:32 which is a Boolean, even though you didn't necessarily declare the variable, like const, age is greater than 18. Yep, that's a variable name and you set
00:01:46 it to true.
00:01:47 And then you went ahead and consulog that variable, which of course would give you true.
00:01:51 But as we have seen before, you can also expect to get a boolean as a result of a comparison.
00:01:59 So it still returns true.
00:02:01 We'll dive deeper into comparisons and logical operators in one of the upcoming modules.
00:02:06 But now that you've learned how to use Booleans to control the flow of your program, let's dive into two more JavaScript data types,
00:02:13 null and undefined.