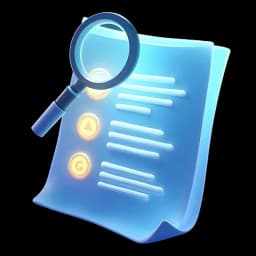
Quick Lecture Overview
Subscribing gives you access to a brief, insightful summary of each lecture to stay on track.
As you progress through the course, you’ll see comments used frequently in the code examples. But what are comments, and why are they important?
A comment is a line or block of text in your code that JavaScript ignores while running. Comments are there to help explain your code—both for yourself and for others.
They’re especially useful when:
Good comments explain why something is done, not just what.
JavaScript supports two kinds of comments:
Single-line comments start with . Anything after on the same line is ignored by JavaScript.
Example:
// This is a single-line comment
const age = 25; // Age of the user
When to use:
// console.log("This line is ignored!");
Multi-line comments start with and end with . Everything in between is ignored by JavaScript.
Example:
/*
This is a multi-line comment.
You can use it to write detailed notes
or explain complex sections of code.
*/
When to use:
/*
This function calculates the sum of two numbers.
It accepts two arguments: a and b.
Returns the result of a + b.
*/
function sum(a, b) {
return a + b;
}
Be Concise but Clear: Avoid writing long paragraphs. Instead, focus on key points.
// Bad comment
// This function takes two numbers as input, adds them together, and then returns the result.
// Good comment
// Adds two numbers
Explain the Why, Not Just the What:
// Bad comment
// Increment the value
value++;
// Good comment
// Increment value to reflect the next step in the process
value++;
Use Comments as Reminders: Mark unfinished tasks with a comment:
// TODO: Add validation for negative numbers
Let’s add comments to a simple program:
// This program calculates the area of a rectangle
// Length of the rectangle
const length = 10;
// Width of the rectangle
const width = 5;
/*
Calculate the area by multiplying
length and width
*/
const area = length * width;
console.log("Area of the rectangle:", area); // Display the area
In this example:
Here’s a template to get you started:
// Declare a variable for the user's name
const name = "James Bond";
// Declare a variable for the user's favorite hobby
const hobby = "driving Aston Martins";
/*
This program combines the name and hobby
to create a fun introduction message.
*/
console.log(`${name} loves ${hobby}.`);
Now that you know how to use comments, you’re ready to make your code clean and understandable. In the next lesson, I’ll teach you how to create JavaScript variables
Go ahead—practice adding comments to your existing code and start building great habits for writing readable, maintainable JavaScript!
By logging in, you'll unlock full access to this and other free tutorials on JSM Pro.
Why? Logging in lets us personalize your learning experience, track your progress, and keep you in the loop with new workshops, coding tips, and platform updates.
You'll also be the first to know about upcoming launches, events, and exclusive discounts.
No spam—just helpful content to level up your skills.
If that sounds fair, go ahead and log in to continue →
Enter your name and email to get instant access
##Looks like we found a thief monkey By the way, I liked the trick how you reached till here. You have a good sense of humor. You will improve a lot if you join our course with this passion.
var
(function-scoped, outdated)let
(block-scoped, modern and recommended)const
(block-scoped, cannot be reassigned)_
, or $
let let = 5;
is invalid)myVar
and myvar
are different)string
, number
, boolean
, null
, undefined
, bigint
, symbol
Objects
, Arrays
, Functions
Subscribing gives you access to a brief, insightful summary of each lecture to stay on track.
00:00:00 As you progress through the course, you'll see me use comments frequently in all code examples.
00:00:05 But what are comments and why are they important?
00:00:08 Well, a comment is a line or block of text in your code that JavaScript ignores while running.
00:00:14 Comments are there to help explain your code, both for yourself and for others.
00:00:20 They're especially useful when you want to revisit your code after weeks or months and need a reminder of what the code does.
00:00:27 Or when you're working with a team and others need to understand your logic.
00:00:30 Or when you simply want to mark areas for improvement or unfinished tasks.
00:00:36 Good comments explain why something is done, not just the what.
00:00:40 So let me show you how they work.
00:00:42 JavaScript supports two kinds of comments.
00:00:45 The first ones are single-line comments.
00:00:48 Single-line comments start with a double forward slash.
00:00:53 Anything after the double forward slash on the same line will be ignored by JavaScript.
00:00:59 So, this is an example of a single line comment.
00:01:02 You might want to use it for quick notes or explanations, or to disable a single line of code while debugging.
00:01:09 Then there are multi-line comments, and they start with a forward slash asterisk, and end with a forward slash asterisk.
00:01:17 but they allow you to stretch them across multiple lines.
00:01:20 So, if you want to write some detailed notes or explain complex sections of the code in multiple lines, you can do that using multi-line comments instead
00:01:29 of just using single lines.
00:01:31 You want to use those for detailed explanations of how a function or a script works, or when you want to document the larger sections of the code.
00:01:39 But of course, when talking about comments, I just have to mention some of the best practices of writing comments.
00:01:45 And the first step would be to be concise but clear.
00:01:49 Avoid writing long paragraphs and instead focus on key points.
00:01:54 An example of a bad comment would be, This function takes two numbers as inputs and adds them together and then returns the result.
00:02:02 Well, hey, if I have that function and I see what it does, then you don't have to repeat yourself, right?
00:02:08 But a good comment would be just adds two numbers, which explains the functionality in a very simple yet concise way.
00:02:16 And then the second tip would be to explain the why, not just the what.
00:02:21 So let me give you an example.
00:02:22 Let's say you want to increment the value.
00:02:25 And you can do that by using value++, but you can do that in a good way to say increment value to reflect the next step in the process.
00:02:33 And then a third quick tip would be to use comments as reminders.
00:02:38 You can mark unfinished tasks with the to do keyword.
00:02:42 For example, to do, add validation for negative numbers or something like an exclamation mark.
00:02:48 This is a bug and needs to be fixed.
00:02:51 You can see how these comments change colors.
00:02:54 How can that be?
00:02:55 Well, this is one example of a cool extension that I installed.
00:02:59 So head over to your extensions and search for comments.
00:03:03 It's called better comments, 8 million downloads, improve your code commenting.
00:03:08 Whenever you add predefined keywords like to do or exclamation mark or a question mark, it'll change the color of that comment to let you know that it's special.
00:03:18 So if we put everything we learned together into a simple quick example, It'll look something like this.
00:03:24 This program calculates the area of a rectangle.
00:03:28 First, you have the length, you have the width, and then you have a variable called area, which simply calculates the area by multiplying the length and
00:03:36 the width.
00:03:37 And you can even put the comments after a specific line to explain what that line does.
00:03:43 But I want to end this lesson on comments with a bit of a controversial opinion.
00:03:47 And that is that you shouldn't really use comments to explain what your code does.
00:03:53 You should instead write code that is simple and easy to understand.
00:03:58 Of course, if you had variable names like L and W and A, In that case, you might actually need the comments to explain that L stands for length or W for width,
00:04:10 right?
00:04:11 But if you use proper variable names and small functions and you think about how you approach writing your code, well, think about it.
00:04:19 In that case, you don't even need any comments because the code speaks for itself.
00:04:25 Of course, when you have super complex functions you cannot understand, go ahead and write a comment, right?
00:04:30 But in most cases, let the code do the talking.
00:04:34 Now that you know how to use comments, you can make your code understandable.
00:04:37 But first, you have to actually write some code.
00:04:40 So let me teach you how to create JavaScript variables.