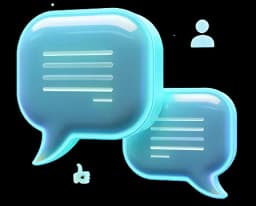
Join the Conversation!
Subscribing gives you access to the comments so you can share your ideas, ask questions, and connect with others.
Learning Objective: Understand JavaScript’s data types and their significance.
In the previous lecture, we talked about how we can store values in variables. These values need to be in the form of one of JavaScript’s predefined data types. If this sounds abstract—don’t worry! We’ll go through it step by step, and by the end of this lesson, it’ll all make sense.
Think of data types as different kinds of containers that hold specific types of information. JavaScript has two main categories of data types:
These are the basic building blocks of data in JavaScript. Let’s break them down with fun examples:
Numbers represent both whole numbers and decimals in JavaScript.
Example: Let’s say you’re counting the number of stars in the sky (simplified, of course):
const stars = 1000000; // A million stars!
const pi = 3.14; // A decimal value for mathematical calculations.
Strings are sequences of characters enclosed in quotes. Think of them as text.
Example: Imagine you’re sending a message:
const message = "Hello, world!";
Booleans represent yes/no, true/false values. They’re super useful for decision-making in code.
Example: Are the cookies baked?
const isBaked = true; // Yes, they’re ready!
const isBurnt = false; // Thankfully, not burnt.
d. Null
represents the absence of a value. It’s like an empty container.
Example: You’ve reserved a table at a restaurant, but the details aren’t finalized yet:
let reservation = null; // No table assigned yet.
means a variable has been declared but not given a value yet.
Example: Imagine writing a note, but forgetting to fill in the details:
let myNote;
console.log(myNote); // undefined
Symbols are unique and immutable identifiers, often used in advanced JavaScript.
Example: Let’s create a unique identifier:
const id = Symbol('uniqueID');
console.log(id); // Symbol(uniqueID)
Objects are the most important data type in JavaScript and the foundation of modern web development.
Think of an object as a collection of related data. For example:
const person = {
name: "James Bond",
age: 007,
car: {
make: "Aston Martin",
model: "DBS",
year: 1969,
},
};
You can access specific properties using dot notation:
console.log(person.car.make); // Aston Martin
Arrays are a type of object that store ordered lists of items.
Example: Imagine listing your favorite movies:
const movies = ["Casino Royale", "Skyfall", "Spectre"];
console.log(movies[1]); // Skyfall
Arrays are perfect for keeping track of collections like shopping lists, to-do tasks, or top-secret missions.
You can easily check the data type of a value using the typeof operator. This is incredibly useful for debugging and understanding your variables.
Example:
console.log(typeof 42); // "number"
console.log(typeof "Hello!"); // "string"
console.log(typeof true); // "boolean"
console.log(typeof null); // "object" (a JavaScript quirk!)
console.log(typeof undefined); // "undefined"
console.log(typeof person); // "object"
console.log(typeof movies); // "object"
Open VS Code and create a new file called .
Experiment by declaring variables for each data type:
Use and to check the data type of each variable. Example:
const age = 25;
console.log(typeof age); // "number"
In the next lessons, we’ll explore each data type in greater detail. You’ll learn how to work with strings, numbers, Booleans, and objects effectively. By the end, you’ll have a solid foundation to build powerful programs.
So, go ahead—experiment with these examples, get comfortable with , and let’s dive deeper into JavaScript types.
"Please login to view comments"
Subscribing gives you access to the comments so you can share your ideas, ask questions, and connect with others.
How did you manage to remove the blur property and reach here?
Upgrading gives you access to quizzes so you can test your knowledge, track progress, and improve your skills.
By logging in, you'll unlock full access to this and other free tutorials on JSM Pro.
Why? Logging in lets us personalize your learning experience, track your progress, and keep you in the loop with new workshops, coding tips, and platform updates.
You'll also be the first to know about upcoming launches, events, and exclusive discounts.
No spam—just helpful content to level up your skills.
If that sounds fair, go ahead and log in to continue →
Enter your name and email to get instant access
##Looks like we found a thief monkey By the way, I liked the trick how you reached till here. You have a good sense of humor. You will improve a lot if you join our course with this passion.
var
(function-scoped, outdated)let
(block-scoped, modern and recommended)const
(block-scoped, cannot be reassigned)_
, or $
let let = 5;
is invalid)myVar
and myvar
are different)string
, number
, boolean
, null
, undefined
, bigint
, symbol
Objects
, Arrays
, Functions
Subscribing gives you access to a brief, insightful summary of each lecture to stay on track.
00:00:00 In the previous lecture, we talked about how we can store values in variables.
00:00:04 These values need to be in the form of one of JavaScript's predefined data types.
00:00:11 If this sounds abstract, don't worry about it.
00:00:14 We'll go through it step by step, and by the end of this lesson, it'll all make sense.
00:00:19 Think of data types as different kinds of containers that hold specific types of information.
00:00:26 JavaScript has two main categories of data, primitive and complex data types.
00:00:32 Primitive data types are the basic building blocks of data in JavaScript.
00:00:38 Let's break them down with a couple of examples.
00:00:40 First, we have the numbers.
00:00:42 Numbers represent both whole numbers and decimal numbers.
00:00:46 Let's say you're counting the number of stars in the sky, or you want to declare pi, which is a decimal value for math calculations.
00:00:54 You can do both using numbers.
00:00:56 Then there are strings.
00:00:59 And strings are just sequences of characters enclosed in quotes.
00:01:04 Think of them as just text.
00:01:06 Imagine you want your program to send a message.
00:01:08 you would declare it as a variable of a data type string and then say what that message does.
00:01:14 Then, if you console log it, you can see it right here in the browser.
00:01:18 After strings, we have booleans.
00:01:21 Booleans represent yes or no or true or false values.
00:01:26 They're super useful for decision-making in code.
00:01:29 For example, are these cookies baked?
00:01:33 Well, if isBaked is true, then yes they're ready, but if isBurnt is false, then thankfully they're not burnt.
00:01:41 Later on we'll explore how we can use Booleans to make different programming decisions.
00:01:46 such as if isBaked is true and isBurnt is false.
00:01:52 Only in that case, we can run a consulog saying cookies are ready.
00:01:57 But if isBaked is false, then we're not going to get that consulogged.
00:02:03 all thanks to the Booleans.
00:02:04 After the Booleans, we have a weird one called null.
00:02:08 Null represents the absence of a value.
00:02:11 It's like an empty container.
00:02:13 Let's say that you have reserved a table at a restaurant, but the details aren't finalized yet.
00:02:19 That would look something like this.
00:02:21 Let reservation is equal to null.
00:02:23 There's also a similar one called undefined.
00:02:26 And undefined means that a variable has been declared, but no value whatsoever has been given to it.
00:02:33 So let's say you're writing a note, but you forget to fill in the details.
00:02:37 And then if you try to console log that note, it'll turn out to be undefined.
00:02:42 And finally, there's also this weird data type called symbols.
00:02:47 Symbols are unique and immutable identifiers used in advanced JavaScript.
00:02:53 Let's say you want to create a unique ID of something.
00:02:56 You can do it by declaring a new variable of ID, equal to, and then you would wrap a string within this symbol keyword, which then will create a unique symbol.
00:03:07 This is not used super often, but hey, it is there.
00:03:11 These have been the primitive JavaScript data types.
00:03:13 But as I said, there's also complex data types called objects.
00:03:19 Objects are the most important data type in JavaScript, and they're the foundation of modern web development.
00:03:26 Think of objects as a collection of related data.
00:03:29 For example, we can declare a new person object by using the curly braces and then defining different primitive variables like a name of string or an age
00:03:39 of 707 right here within that object.
00:03:42 Or you can even nest additional objects within that parent object.
00:03:47 such as if you want to explain the details of a car that this person is driving.
00:03:52 And then you can access specific properties of that object by using the dot notation.
00:03:58 So if you want to consulog the car make, you would need to say person.car.make, and that would spit out Aston Martin.
00:04:07 Next to objects, we also have access to something known as arrays.
00:04:12 And arrays are technically just a special type of an object.
00:04:16 They store ordered lists of items.
00:04:20 Imagine listing your favorite movies, just like this.
00:04:23 const movies is equal to, and then you can use the square bracket notation instead of the curly brace notation that we use for the objects,
00:04:32 and then just define a list of different data types within it.
00:04:35 They can be strings, but you can also define different objects within it.
00:04:40 such as a movie name or a title right here.
00:04:43 And then if you want to get access to the values that you stored in that array, you can just say movies and then using the square bracket notation again,
00:04:51 you can access the values from zero to one or two.
00:04:56 Important thing is that arrays start from zero.
00:04:59 So this is the zeroth element, the first one and the second one.
00:05:03 Of course, we'll dive into so much more detail about all of these complex data types in upcoming lessons.
00:05:09 But for now, the only thing you have to know is that arrays are perfect for keeping track of collections like shopping lists,
00:05:16 to-do tasks, or top secret missions.
00:05:19 Now, when talking about data types, it's also important to know how we can identify different data types.
00:05:25 And we can do that by using the JavaScript's typeof operator.
00:05:29 You write it like this.
00:05:31 Typeof.
00:05:32 This is incredibly useful for debugging and understanding your variables.
00:05:36 So, as an example, we can see that the typeof operator of all of these values tells us exactly what it is.
00:05:45 42 is a number, hello is a string, true is a boolean, null is an object, This is a JavaScript quirk, which we can talk into a bit more detail later on,
00:05:56 but just so you know, everything in JavaScript is an object.
00:06:00 And then there's also the undefined, which has its own type.
00:06:03 And then both person and movies, if we declare them, of course, would be objects.
00:06:08 Even though we call it an array, behind the scenes, it is still an object.
00:06:14 Now, as a quick activity, you can try experimenting by declaring a couple of variables for each data type, like a number for your age,
00:06:22 a string for your favorite movie or a book, and some booleans as well.
00:06:26 Then console log them and see whether you can guess their type correctly.
00:06:29 Now, in the next lesson, let's explore each data type in more detail.
00:06:34 You'll learn how to work with strings, numbers, booleans, and objects so that by the end, you have a solid foundation to build powerful JavaScript programs.