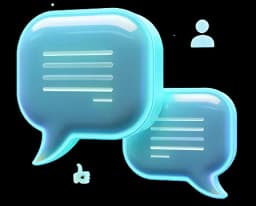
Join the Conversation!
Subscribing gives you access to the comments so you can share your ideas, ask questions, and connect with others.
Learning Objective: Understand JavaScript’s flexible number handling and how to work with basic numbers.
Numbers are a fundamental data type in JavaScript. Unlike some programming languages, you don’t need to specify whether a number is an integer or a float—JavaScript takes care of it for you. This is because JavaScript is dynamically typed.
In JavaScript:
const wholeNumber = 5; // Integer
const decimalNumber = 3.14; // Float
In other languages like C, you would need to explicitly declare the type:
int wholeNumber = 5;
float decimalNumber = 3.14;
In JavaScript, both integers and decimals fall under the same number type, simplifying how we work with them.
You can perform all the standard math operations in JavaScript, such as addition, subtraction, multiplication, and division.
const a = 10;
const b = 5;
console.log(a + b); // Addition: 15
console.log(a - b); // Subtraction: 5
console.log(a * b); // Multiplication: 50
console.log(a / b); // Division: 2
, short for Not a Number, is a special numeric value in JavaScript. It often occurs when you try to perform an invalid mathematical operation.
console.log("hello" * 3); // NaN
Interestingly, even though stands for "Not a Number," its type is still :
console.log(typeof NaN); // "number"
Why? In computing, is technically part of the numeric type system, even though it doesn’t represent a valid number.
We’re keeping it simple for now, but JavaScript also has operators for more advanced calculations, like modulus , exponentiation , and more.
You’ll learn about these in detail in the upcoming module, Operators and Equality, where we’ll explore:
For now, focus on getting comfortable with basic numbers and operations.
Let’s put this into practice:
Example:
const whole = 7;
const decimal = 3.14;
console.log(whole + decimal); // Addition
console.log(whole - decimal); // Subtraction
console.log(whole * decimal); // Multiplication
console.log(whole / decimal); // Division
You’ve just learned the basics of working with numbers in JavaScript. Keep practicing, as numbers are a key part of programming. In the next lesson, we’ll explore Booleans, which will help us add logic and decisions to our programs.
"Please login to view comments"
Subscribing gives you access to the comments so you can share your ideas, ask questions, and connect with others.
By logging in, you'll unlock full access to this and other free tutorials on JSM Pro.
Why? Logging in lets us personalize your learning experience, track your progress, and keep you in the loop with new workshops, coding tips, and platform updates.
You'll also be the first to know about upcoming launches, events, and exclusive discounts.
No spam—just helpful content to level up your skills.
If that sounds fair, go ahead and log in to continue →
Enter your name and email to get instant access
##Looks like we found a thief monkey By the way, I liked the trick how you reached till here. You have a good sense of humor. You will improve a lot if you join our course with this passion.
var
(function-scoped, outdated)let
(block-scoped, modern and recommended)const
(block-scoped, cannot be reassigned)_
, or $
let let = 5;
is invalid)myVar
and myvar
are different)string
, number
, boolean
, null
, undefined
, bigint
, symbol
Objects
, Arrays
, Functions
Subscribing gives you access to a brief, insightful summary of each lecture to stay on track.
00:00:00 Numbers are a fundamental data type in JavaScript.
00:00:04 Unlike some programming languages, you don't need to specify whether a number is an integer or a float to let it know whether it's a whole number or a
00:00:13 decimal number.
00:00:14 JavaScript takes care of it for you.
00:00:16 This is because JavaScript is dynamically typed.
00:00:20 So the only thing you have to do is declare it with const And no matter whether it's a whole number or a decimal number,
00:00:27 JavaScript will recognize it immediately.
00:00:29 Because both integers and floats fall into the same category.
00:00:33 The category of a number.
00:00:35 In JavaScript, you can also perform all the standard math operations, such as addition, subtraction, multiplication, and division.
00:00:44 And when talking about numbers in JavaScript, we can't not talk about none.
00:00:49 short for not a number.
00:00:52 It is a special numeric value in JavaScript that often occurs when you try to perform an invalid mathematical operation.
00:01:00 It looks something like this.
00:01:02 If you try to consulog the string of hello and multiply it by the number 3, JavaScript won't know what the heck to do with it,
00:01:10 so it'll say none.
00:01:12 not a number.
00:01:13 But interestingly enough, even though NAN stands for not a number, if you use the typeof operator on the NAN keyword, you're going to get back,
00:01:22 well, a number.
00:01:23 So why is that?
00:01:25 Well, that's because in computing, NAN is technically a part of the numeric type system, even though it doesn't represent the valid number.
00:01:33 I'm keeping it simple for now, but JavaScript also has more operators for advanced calculations.
00:01:40 And you'll learn about all of these in detail in the upcoming modules of operators and equality, where we'll explore arithmetic operators,
00:01:49 logical operators, assignment operators, and the comparison and equality topic.
00:01:55 But for now, focus on getting comfortable with the basic numbers and operations.
00:02:00 And in the next lesson, let's explore booleans, which will help us add logic and decisions to our programs.