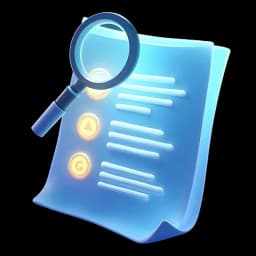
Quick Lecture Overview
Subscribing gives you access to a brief, insightful summary of each lecture to stay on track.
Learning Objective: Introduce the concept of objects as collections of data.
An Object is the most important data-type and forms the building block for modern JavaScript.
The type is special. All other types are called “primitive” because their values can contain only a single thing (a string or a number or whatever). In contrast, objects are used to store collections of data and more complex entities.
"in simple language object are used to group variables."
Create a variable of name, and age:
const name = "John";
const age = 25;
We can create an called person and put them together:
const person = {
name: 'John',
age: 25;
}
console.log(person) // { name: 'John', age: 25 }
Once you assign the value to an . We can now extract specific values from that object using the dot notation:
person.name;
person.age;
console.log(person.name); // John
console.log(person.age); // 25
Objects are very much useful, when it comes to storing data in real world. For example, you want to store the details of a person:
const person = {
name: "John",
age: 25,
isMarried: false,
hobbies: ["reading", "coding", "gaming"],
};
console.log(person); // { name: 'John', age: 25, isMarried: false, hobbies: ['reading', 'coding', 'gaming'] }
Example:
const person = {
name: "John",
age: 25,
isMarried: false,
hobbies: ["reading", "coding", "gaming"],
};
console.log(person.name); // John
console.log(person.age); // 25
In this lesson, you learned how to create an object and access its values using dot notation. Objects have many special features, which we will explore later. Now, let’s dive into Statically vs Dynamically Typed Languages!
By logging in, you'll unlock full access to this and other free tutorials on JSM Pro.
Why? Logging in lets us personalize your learning experience, track your progress, and keep you in the loop with new workshops, coding tips, and platform updates.
You'll also be the first to know about upcoming launches, events, and exclusive discounts.
No spam—just helpful content to level up your skills.
If that sounds fair, go ahead and log in to continue →
Enter your name and email to get instant access
##Looks like we found a thief monkey By the way, I liked the trick how you reached till here. You have a good sense of humor. You will improve a lot if you join our course with this passion.
var
(function-scoped, outdated)let
(block-scoped, modern and recommended)const
(block-scoped, cannot be reassigned)_
, or $
let let = 5;
is invalid)myVar
and myvar
are different)string
, number
, boolean
, null
, undefined
, bigint
, symbol
Objects
, Arrays
, Functions
Subscribing gives you access to a brief, insightful summary of each lecture to stay on track.
00:00:00 Object is the most important data type and forms the building block for modern JavaScript.
00:00:06 The object type is quite special because all other types we have discussed so far are also called primitive because their values can contain only a single thing,
00:00:16 be it a string or a number or whatever.
00:00:19 but in contrast, objects are used to store collections of data and more complex entities.
00:00:25 Now, to keep it simple, I just want to let you know that objects in their simplest form are used to group different variables together.
00:00:33 For example, let's say you have a variable of name set to John and age set to 25. These two variables in the current state are in no way related to one another,
00:00:44 but now we can create an object called person and put them together.
00:00:49 Now we know that both name and age belong to the same entity, the person.
00:00:55 This is an object.
00:00:57 And as you can see, we declare it the same way we do with all the other variables, and then put curly braces inside of which goes the data.
00:01:05 The one last thing I want to mention is that you can now extract specific values from the object using something known as the dot notation.
00:01:15 So whereas if you wanted to simply console log the name above when it's not in an object, you would just say name like this and get John.
00:01:25 But if you want to get something like a name out of an object, you would first have to mention that object name, like person,
00:01:32 and then use the dot notation to get access to its name.
00:01:36 You can do the same thing with the age, of course.
00:01:39 So you would get John of 25. There's also something known as a square bracket notation, where you can put square brackets and then use a string to define
00:01:49 what you want to get out of it, like this.
00:01:51 It would also give you back John, but more on that later.
00:01:55 There are many other kinds of objects in JavaScript, from arrays, which are used to store ordered data collections, to the date object to store information
00:02:05 about the date and time, and even the error object that is used to store information about an error.
00:02:12 All of these have their special features that we'll dive into later.
00:02:15 Sometimes people say something like array type or date type.
00:02:19 And even though arrays feel like their own type, formally they are not types of their own, but they belong to a single object data type.
00:02:29 and then they extend it in different ways.
00:02:31 That's all that I want to let you know for now.
00:02:34 Objects are complex concepts.
00:02:36 So first, let's master a couple more easy things, and then we can get back to them in one of the later modules, where I'm going to teach you everything
00:02:45 there is to know about objects.