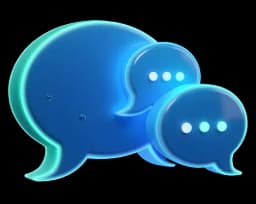
No Comments Yet
Be the first to share your thoughts and start the conversation.
Be the first to share your thoughts and start the conversation.
How did you manage to remove the blur property and reach here?
Upgrading gives you access to quizzes so you can test your knowledge, track progress, and improve your skills.
By logging in, you'll unlock full access to this and other free tutorials on JSM Pro.
Why? Logging in lets us personalize your learning experience, track your progress, and keep you in the loop with new workshops, coding tips, and platform updates.
You'll also be the first to know about upcoming launches, events, and exclusive discounts.
No spam—just helpful content to level up your skills.
If that sounds fair, go ahead and log in to continue →
Enter your name and email to get instant access
In this lesson, we focus on building the user interface for a home screen using React Native. The process begins with setting up the structure and styling of components, particularly emphasizing the header and search bar functionalities.
showsVerticalScrollIndicator
to false.00:00:02 To start developing the UI over home screen, head over into app, tabs, and then index.tsx.
00:00:10 Here is where we have this welcome.
00:00:11 So for now, let's focus on implementing the header component.
00:00:15 I'll clear this entire view and create another.
00:00:19 This one will have a class name equal to flex-1 and bg of primary.
00:00:26 Finally, we're going to go away from this light screen to a nice dark color.
00:00:31 Within this view, we can render an image coming from React Native.
00:00:35 See how WebStorm very easily auto imports it at the top.
00:00:39 And we can render a source and give it a source of images.
00:00:43 Now keep in mind, if I just press Enter, it'll auto import it once again from at forward slash constants forward slash images.
00:00:51 And I'll call the images.bg for the background.
00:00:55 Let's position it a bit by giving it a class name equal to absolute.
00:01:00 Let's also give it a W full, so it takes the full width of the screen, as well as a Z index of zero, so we can show some elements on top of it.
00:01:09 Right below it, we can render a scroll view.
00:01:12 This is used to make the whole screen scrollable.
00:01:15 So if there's a lot of vertical content that might not fit within a single view, then you need to wrap everything within a scroll view for users with smaller
00:01:24 devices to be able to see it.
00:01:26 Let's give it a class name, equal to flex-1.
00:01:31 and a padding X of 5 to give it some horizontal spacing.
00:01:34 Within it, for now, let's just render a single image that'll have a source equal to icons, again, coming from constants,
00:01:45 dot logo, with a class name equal to W of 12 for the width, H of 10 for the height, margin top of 20 to divide it a bit from the top,
00:01:56 margin bottom of five, and margin X of auto to center it in the middle.
00:02:02 There we go.
00:02:02 This is starting to look like a real app already.
00:02:05 Now, if we had more content, we would have this ugly scroll bar at the right side.
00:02:10 So to remove it, we can give an additional prop to the scroll view called show, or rather shows, vertical scroll indicator,
00:02:19 which I'll set to false.
00:02:21 And I'll also give it a content.
00:02:24 container style, which will be equal to an object where min height will be set to 100% and also padding bottom will be set to 10. So now you can see if
00:02:37 you scroll up and down, it kind of moves, the logo moves, right?
00:02:40 But we have no content to show.
00:02:42 So very soon we'll be able to add some content.
00:02:45 But first things first, we have to ask ourselves, what is the first thing that we want to show on the homepage?
00:02:51 And it'll be the search bar.
00:02:52 which will be our first component of the day.
00:02:55 So let's create a new components folder.
00:03:02 And within it, let's create a new file called search-bar.tsx.
00:03:10 And within it, run rnfe to quickly create a new component.
00:03:16 Now we want to be able to see that component somewhere.
00:03:19 So let's head back into the index.tsx and let's head right below this logo image and render the view that'll have a class name of flex1 and margin top
00:03:34 of 5. And within it, just to render a self-closing search bar component coming from at forward slash components forward slash search bar.
00:03:44 Now, you won't be able to see it yet, because right now, it is just a single piece of text, which is hard to see on a dark background,
00:03:51 but of course, we're gonna style it further.
00:03:53 So, let's start by giving this view a class name, equal to flex-row, items-center, bgdark200, rounded-full and padding X of five,
00:04:07 as well as padding Y of four to create some spacing.
00:04:10 In between it, we can render an image, which is going to be kind of like a search icon.
00:04:16 So let's import the image component and give it a source equal to icons.search with a class name equal to size of five.
00:04:28 A resize mode equal to contain.
00:04:33 As well as a tint color equal to hash AB8BFF.
00:04:40 And of course, don't forget to import the icons coming from Constance.
00:04:45 If you do that, you should be able to see this little icon at the top left.
00:04:49 Below it, we can render a text input.
00:04:52 Okay.
00:04:53 So a text input is like an input component in React or in regular HTML.
00:04:59 And it allows you to pass similar things to it, such as the onPress functionality, which for now I'll just leave as an empty callback function,
00:05:07 a placeholder that you can pass to it, which can be something like search for now, a value, which can be equal to an empty string for now,
00:05:16 an onChangeText, which for now will be equal to an empty callback function, a placeholder text color, which can be similar to our tint color,
00:05:26 a8b5db.
00:05:29 There we go, now we can see it.
00:05:30 And finally, we can give it a class name of flex-1, margin left of 2, and text-white.
00:05:38 So now we have what appears to be a very simple search, and when you click on it, you can see that your keyboard actually opens up,
00:05:45 giving you a way to type something in.
00:05:47 Now, what we need to do to make this somewhat functional is pass the real on-press value from the index.
00:05:54 So typically, when you have smaller components like a search bar on its own, it doesn't necessarily have to be concerned with what it is doing.
00:06:02 Typically, you have some logic that you pass from the parent component to the child component, in this case, the search bar.
00:06:10 So what we can do is define the router right here in the homepage.
00:06:16 You can say something like import use router coming from expo-router.
00:06:22 You can define that router right here by saying const router is equal to use router.
00:06:28 By the way, this is the first time we're using the router right here.
00:06:31 And what we're doing here is essentially using something known as a hook.
00:06:35 So in React, and therefore in React Native, when something starts with the word use, that means that it is called a hook.
00:06:44 And hooks are typically called at the top of our functional components.
00:06:48 And then they expose additional functionalities.
00:06:51 In this case, the router functionality allows us to move between different screens programmatically, not necessarily by clicking a button or something,
00:07:00 but we can use it to go to another page or screen when something happens.
00:07:05 In this case, I will pass two props to the search bar.
00:07:09 The first one will be the onPress functionality that will for now call the callback function and then call the router.push() which will push it to forward
00:07:20 slash search.
00:07:21 Okay, so we want to move to the search screen once pressed, and I'll also pass a placeholder equal to search for a movie.
00:07:29 Now, if we head into the search bar, we can accept these different props.
00:07:33 So right here at the top, I can destructure them and accept the placeholder as well as the on press.
00:07:41 And these can be of a type of props, So right above it, we can define a TypeScript interface called props and just say that the placeholder will always
00:07:52 be of a type string and onPress will be optional and it'll be of a type function that returns void, meaning nothing.
00:08:03 The goal of this function is not to return something, rather it is to use the router functionality to push to a different URL.
00:08:11 So if you haven't used TypeScript before, don't worry.
00:08:14 What this interface does is it simply tells our React application, or React Native in this case, what the types of those props should be.
00:08:22 So now onPress, we can call the onPress functionality that we're passing through props, and also we can pass in the placeholder.
00:08:32 There we go, search for a movie.
00:08:34 Now check this out.
00:08:36 As soon as I click search for a movie, it actually redirects me to another screen called the search screen, which we'll implement very,
00:08:44 very soon.
00:08:45 But of course, it doesn't make sense to start implementing the search a movie screen if we don't yet have any movies to search for.
00:08:52 So in the next lesson, let's go ahead and fetch the movies data from a third party API.