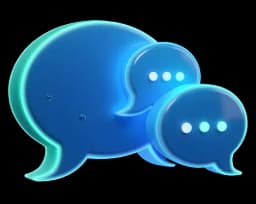
No Comments Yet
Be the first to share your thoughts and start the conversation.
Be the first to share your thoughts and start the conversation.
How did you manage to remove the blur property and reach here?
Upgrading gives you access to quizzes so you can test your knowledge, track progress, and improve your skills.
By logging in, you'll unlock full access to this and other free tutorials on JSM Pro.
Why? Logging in lets us personalize your learning experience, track your progress, and keep you in the loop with new workshops, coding tips, and platform updates.
You'll also be the first to know about upcoming launches, events, and exclusive discounts.
No spam—just helpful content to level up your skills.
If that sounds fair, go ahead and log in to continue →
Enter your name and email to get instant access
In this lesson, we explore the fundamentals of React Native, focusing on its components and how they differ from standard React components. The presenter discusses the coding similarities and differences, essential components for building mobile applications, and their functionality.
00:00:00 Alright, let's jump right in.
00:00:02 We've already talked about the benefits and drawbacks of React Native and how Expo can make your life easier.
00:00:09 Now it's time to dive into the code and see how it works.
00:00:12 If you've worked with ReactJS before, you'll find that React Native uses a similar syntax.
00:00:18 But of course, there are some differences you should be aware of.
00:00:22 So, let me show you the ins and outs of React Native code.
00:00:25 We can check out how it looks and how it functions when compared to React.js to help you understand the similarities, but also the differences between
00:00:33 the two.
00:00:34 So super quickly, you'll understand React Native's components and how to use them.
00:00:39 When coding in React Native, you use JavaScript, just like with React.
00:00:44 But instead of rendering HTML elements, you'll be rendering native mobile components.
00:00:51 Take a look at this basic React Native component.
00:00:54 Here, we're importing two important components from React Native library.
00:00:59 and text.
00:01:00 Then we create a functional component called app that returns the text component wrapped inside of a view.
00:01:06 What's interesting here is that we're using JSX syntax, which makes it super easy to create and visualize our components in a more HTML-like way.
00:01:15 But if you take a closer look, you can quickly see that this is neither a P tag, nor an H tag, nor anything that we're used to while writing code that
00:01:24 runs in the browser.
00:01:26 In React Native, we use text instead.
00:01:29 It's pretty straightforward.
00:01:30 The text component is used to display text in the app, and you can style it using the same CSS-like syntax as in React.
00:01:38 You can set the font size, color, and weight using the style prop.
00:01:42 React Native also offers a style sheet utility.
00:01:45 that allows you to define styles by creating a single JavaScript object.
00:01:50 This is super handy in larger applications as it optimizes performance.
00:01:54 But as we all know, Tailwind CSS is rising in popularity.
00:01:58 So in React Native world, Nativewind came into the picture, allowing you to write Tailwind-like CSS styles in React Native.
00:02:06 Isn't this crazy?
00:02:07 It feels like you're writing a regular web app, but instead you're developing apps for mobile.
00:02:13 Now let's talk a bit about the view component.
00:02:16 Think of it as a box or container that holds other components.
00:02:20 Similar to the Div element in HTML, but with some added functionality specific to mobile apps.
00:02:25 The View component is often used to create layout structures for other components.
00:02:32 It has many different props that can be used to control its appearance and behavior.
00:02:37 And one thing to note is that the View component uses Flexbox layout by default.
00:02:43 which makes it really easy to control how its children components are laid out.
00:02:48 So you can use Flexbox properties like Flex Direction, Justify Content, and Align Items to achieve any layout you want.
00:02:55 But what if you want to add some interactivity to your React Native apps?
00:02:59 Well, get excited because there are some amazing components that do just that.
00:03:04 Components for creating buttons, links, and other interactive elements.
00:03:08 The first one on the list is Touchable Opacity, which is great if you want to create a simple button.
00:03:14 Think of it like a cousin to a React button component, but with even more room for customization.
00:03:20 And instead of an onclick, in React Native, you're not clicking it, you're pressing it.
00:03:25 So let's provide an onpress.
00:03:27 The second similar component is called a Touchable Highlight, which allows views to respond to touch in a unique way.
00:03:36 When touched, the component reduces the opacity of the wrapped div, revealing the underlying color.
00:03:42 And then there's the touchable without feedback, which is there if you need to create an element that is clickable, but you don't want it to have any visual
00:03:51 feedback when pressed.
00:03:52 It is super useful when creating links or images that don't need any additional effects.
00:03:57 And apart from these touchable components, there's also activity indicator, which allows you to show a spinner or a loading indicator within your app.
00:04:06 Sure, there's also a simple button allowing you to set properties like the title, color, and an on press that is called when the button is pressed.
00:04:14 But whenever you need some more advanced styling or behavior, you'll find yourself using the touchable components much more often as they offer greater flexibility.
00:04:23 Now, the next super important component on the list of components I want to teach you right now, and which we'll use later on within our app,
00:04:32 is called a flat list.
00:04:34 A flat list is perfect for rendering long lists of items that need to be scrolled.
00:04:41 It's like the map function in React, but with some extra features like optimized scroll performance and item separation.
00:04:48 The way it works is you, of course, import it from React Native, and then you define some data, such as an array of objects you want to map over.
00:04:57 Then you put it all in a view, and you call it flat list.
00:05:02 A flat list by default already accepts some props, such as the data prop, to which you can pass an array of data you want to map over,
00:05:10 and then a render item prop, which allows you to define exactly how you want to represent each item in the array.
00:05:18 Pretty cool, right?
00:05:19 Now, when should you use a flat list and when should you just map over the elements?
00:05:25 Well, for larger lists where you want to have smooth scrolling, go for the flat list.
00:05:30 While for the smaller lists, the map function will do the job.
00:05:33 There's also something known as a scroll view.
00:05:36 You can think of it like a magic box that can hold multiple components and views, providing you a scrolling container for them.
00:05:43 It's like the overflow scroll property of a div in HTML, allowing you to easily navigate through a list of items or large amounts of content in our app.
00:05:53 You can put it within a view, call it scroll view, and then render some elements within it.
00:05:57 By using the scroll view component, you can make sure that users can easily explore all the content, making your app more intuitive.
00:06:04 And there's also a component which you'll find yourself using super often called safe area view, which gives you some sort of a safe zone to render your
00:06:14 app's content within without it being covered by the device's hardware features like the notch, home indicator, or a status bar.
00:06:22 It's great for building apps that are supported on different devices with different screen sizes and shapes.
00:06:28 The way you use it is whenever you think something might be too long or awkwardly placed, you just wrap it within a safe area view.
00:06:35 And while that default safe area view is amazing for most cases, sometimes it does fall short for some devices, making it not the optimal choice.
00:06:44 So I often like to use a package called React Native Safe Area Context, which works across all devices, even for the bottom bar.
00:06:53 But you don't have to worry about remembering all of that right now.
00:06:55 I'll show it to you once you start developing your own first app.
00:06:59 But bear with me for a moment.
00:07:01 I first want you to know which components exist so we can later on use them more easily.
00:07:06 For example, we'll surely have to display some images within our app, right?
00:07:10 So how can we do that in React Native?
00:07:13 Well, thankfully, once again, it is super similar to how it looks like in React.
00:07:17 You just use the Image component provided by React Native, you pass the source to it, which can be a path or a URL, and you're good.
00:07:25 But if you want to display an image as a background, then you should use the ImageBackground component.
00:07:31 which works in the same way, but just renders it as a background.
00:07:35 It's specifically designed to allow other components to be layered on top of it, whereas just the image is used for displaying images on their own.
00:07:44 And both of these can handle different image formats like PNGs, JPEGs, GIFs, and WebPs, but none support SVG files.
00:07:52 because of some native rendering limitations.
00:07:54 So if you want to use SVGs, there's a third-party package called React Native SVG.
00:08:00 Once again, don't try to remember it.
00:08:02 I'll show it to you once again later on, so you can use it within your own code.
00:08:06 Okay, now that you know how to use images, what about models?
00:08:09 Well, thankfully, React Native has components for that too.
00:08:13 It's called a model.
00:08:14 Just import it from React Native, set visible to true, add an animation type, and define what happens when you close it.
00:08:22 Pretty simple.
00:08:23 There's also an alert component, which you can, again, just very conveniently import from React Native and call it.
00:08:30 By saying alert.alert, provide a title and functions which you want to execute on cancel or on okay.
00:08:38 And if you're creating forms, you might want to create some kind of a toggle in React Native.
00:08:42 And for that, you can use a switch component.
00:08:45 Once again, it is super simple, imported, create a state for it, same as in React.
00:08:52 create a function that manages it, and then simply call it like this within your code, provide some colors, its value, and what happens when you click it.
00:09:01 There's also a component that I use super often called the status bar.
00:09:05 Both React Native and Expo have their own versions that allow you to control how status bar should look like for each screen within the app.
00:09:13 I prefer using the one from Expo.
00:09:15 You can define a view with some text, and just beneath it, you can define a status bar.
00:09:20 But what am I even trying to do?
00:09:22 I'm just listing all of the different components that you can use to build your mobile applications.
00:09:27 Well, I guess I'm just trying to show you that there are some differences, like P tags and H tags are now becoming text,
00:09:35 divs are becoming views.
00:09:37 But other than that, there are so many components that feel like you're writing React code within React Native, allowing you to build mobile applications.
00:09:47 From touchable opacities for buttons to models, alerts, and more, the list doesn't stop there.
00:09:54 Of course, I don't wanna waste your time.
00:09:56 We're not gonna go through every single component that exists in this video.
00:10:00 But I did take some time and I wrote down the most important ones within the free React Native guide, linked in the description.
00:10:07 So, if you wanna check them out there, see the differences and similarities, and just have a place where you can recap what you learned,
00:10:13 that guide is what you need.
00:10:15 But now that you know the most important components and concepts in React Native, let's dive right into action.
00:10:20 I'll show you how to set up your app, what files and folders are involved, and everything else that matters.
00:10:26 Step by step.